Python String split()
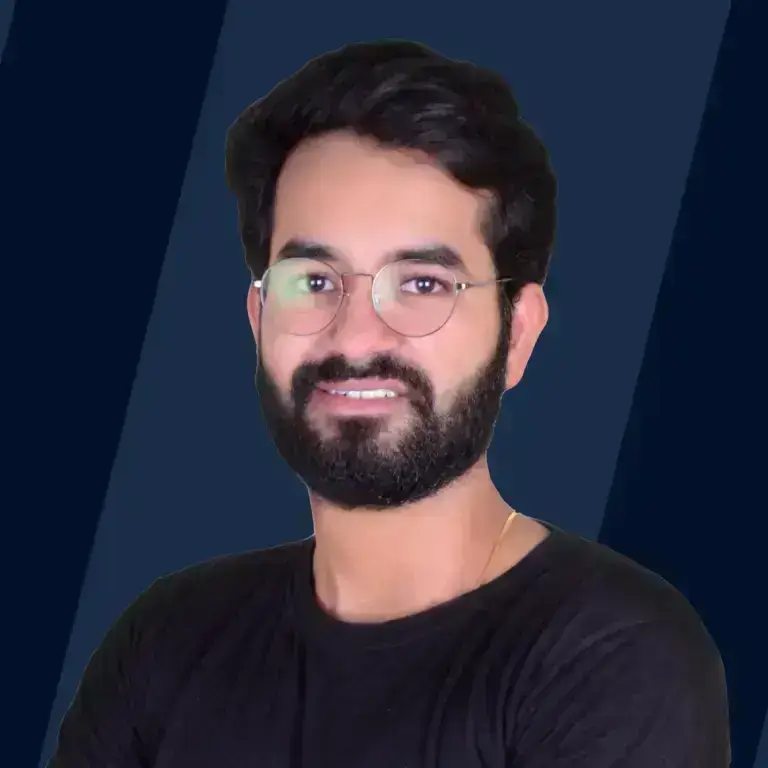
The split() function in Python is used to split a given string into a list of small substrings according to a separator and finally it returns that list where elements are nothing but the split parts of the string.
Example of split() in Python
Here we are going to discuss a simple example based on the split function.
Code:
Output:
Explanation:
The string S1 is split by whitespaces as we have not provided any separator, The string S2 is split by commas. Subsequently list for both is being returned.
Syntax of Split () Function in Python
The syntax of split() method in Python is as follows:
The myString is the string on which the split function called.
Parameters of Split () Function in Python
split() function in Python takes two parameters:
- separator: The separator is the character by which the main string is to be split into smaller substrings. If it is not provided the whitespace is considered as separator by default.
- maxsplit: It tells the number of times the string should be split, It is a number value and if not provided, by default it is -1 that means there is no limit.
Return Value of Split () Function in Python
Return Type: list
This Function returns a python list containing the split strings.
What is split() function in Python?
Take a case where you have given a list of names separated by commas and you have to separate all of them and display them,
In that case, you can use the split() function that will easily separate all the names by commas, and then you can easily display them.
As the name suggests, the split() function in Python is used to split a particular string into smaller strings by breaking the main string using the given specifier. The specifier is the character separator to use while splitting the string, for example - "@", " ", '$', etc.
Example : Using maxCount Parameter
We can set the Maximum Number of parts in which string should be split.
Code:
Output:
Explanation:
The string S1 will be split at whitespaces three times as we have provided max parameter as 3. The string S2 will be split at '@' two times as we have provided max parameter as 2
Example : Combining the Returned Array
We can also do other useful operations on returned list i.e. combining it into a single string.
Code:
Output:
Explanation:
String S1 is split by '#' and then is stored in a list and further join operations is performed on that list subsequently the list combines to the new string.
How to Parse a String in Python using the split() Method?
Python's split() method is a powerful tool for parsing strings, enabling us to divide a string into a list of substrings based on a specified delimiter. This method is particularly useful when working with text data that needs to be separated into manageable parts for further processing or analysis.
Basic Usage of split() Method:
To split a string by spaces (default behavior):
Output: ['Python', 'is', 'amazing']
Splitting Using a Specific Separator:
You can specify a separator to divide the string at every occurrence of this separator:
Output: ['apple', 'banana', 'cherry']
Limiting the Number of Splits with maxsplit:
The maxsplit parameter controls the maximum number of splits:
Output: ['one', 'two', 'three:four']
Conclusion
- The split() function in python is used to split a particular string into smaller strings by breaking the main string using the given specifier.
- It returns a list containing the split strings.
- It works for a string and takes 2 parameters, specifier and maximum count for split.
- Finally the list returned can be iterated over and all the split strings can be easily accessed in that list.