How to Create 2D ArrayList in Java?
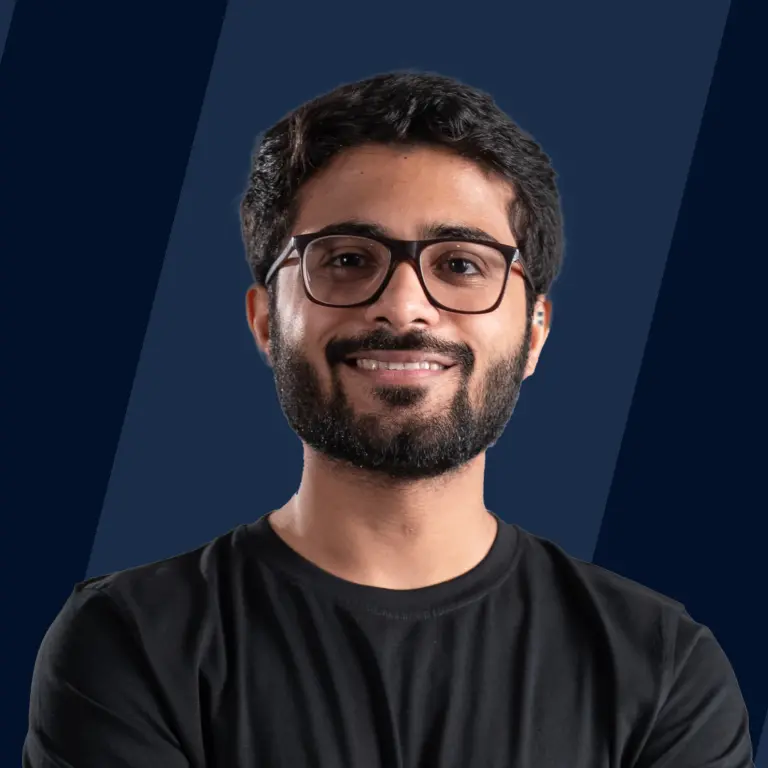
Overview
You must've heard about Arrays in Java, but what's ArrayList you wonder. Well, ArrayList functions just like arrays, you can add or remove elements, but then how does it make ArrayList any different from Arrays?
While Arrays are fixed data structures, ArrayList is not, you can add elements to your heart's content here! (or well till Integer.MAX_VALUE)
While Array is a basic functionality provided by Java, ArrayList however comes from Java's collection framework and so unlike Arrays where we used to access array members using [], we use methods in the case of ArrayLists instead.
Curious to know more about how ArrayList differs from Array? We already have an article you should check out! click me
Let's get back to our topic and see how we can create 2D ArrayLists in Java
Introduction to 2D ArrayList in Java
ArrayList implements the list interface in Java The standard Array class is not as adaptable as the ArrayList class. It uses all of the List Interface's functions by implementing the List interface. It occurs in the java.util package.
The ArrayList class implements the List Interface and inherits from the AbstractList class. Its components can be accessed at random. It cannot be used for primitive types like int, char, etc.; we must construct a wrapper class for these data types.
Let's look at the hierarchy of ArrayList in Java
In ArrayList, we can retrieve elements by their indexes much like arrays, since they use arrays to store elements Being an ordered collection it maintains the insertion order of elements We can also duplicate and null values with 2D ArrayLists
Now, 2D ArrayLists are also called as ArrayLists of Objects, why is that? It's because the 2D ArrayList java class only contains objects. Now what do we do if we have to store an integer-type element in there? Well then we'll need to use integer objects of wrapper class instead of primitive type int
Syntax
Before moving on further let's stop and see how we can add ArrayList class to our program in the first place We can do this with the import directive as shown below
OR
After this, we can create an ArrayList objects
Let's now look at the syntax of a 2ArrayListst in Java
Initialize 2d Arraylist Java
You've finished making the ArrayList now. When you're ready, initialise it with values. Here are a few strategies for you.
Use Arrays.asList
You can initialize a 2d Arraylist in Java by passing an Array that has been converted to a List using the Arrays.asList function.
Syntax
We provide you with a 2d ArrayList java example to serve as an example.
Output
Here we've created an ArrayList named as arraylist which stores 3 characters C, A, and T in the form of a list, we've used Arrays.aslist() for returning characters as a list like
We then use the print statement to print the list of contents
Anonymous Inner Class Method
Let's look at the syntax of the Anonymous inner class method in Java
Now let's see an example in Java for implementthe ing Anonymous inner class method
Output
We've created an ArrayList of strings named str and added three words using the Anonymous inner class method as you can see here
The contents of the list are then printed
Add Method
As the name implies, this technique is widely used to add elements to collections.
Let's take a look at its Syntax
Let's see an example in Java for implementing Add method
Output
Collection.nCopies Method – 2d Arraylist Java Example
You can initialize the ArrayList with comparable values using this method. then, this is its basic syntax.
Let's now think on this illustration. As an illustration, let's build an ArrayList with 10 entries and initialise it to the number 10.
How Does 2D ArrayList Work?
Let's now see how this 2D ArrayList we keep talking about works
We can see from the example diagrammatic depiction of the operation of two-dimensional arrays in Java that each column is represented by the values of the row and column level indices. The first index denotes the value of the row, while the second indexes denote the value of the column. In the format of a[0][0], a[0][1], etc., this is shown
The Java ArrayList can retain the insertion order by the value insertion trigger.
Arrays also have unique indices. Vectors are also Java components that perform the same function as two-dimensional and multi-dimensional array lists; the important difference between these items is that vectors do not synchronize. This is one of the key items that distinguishes the two dimensional ArrayList from vectors. the ability to operate alone.
In multidimensional and two-dimensional array lists, the ability to access every item without regard to the order is primarily a key advantage. With the aid of a new term, space for the 0th row can be allocated, as demonstrated in this line. The 0th row also permits the default storage of a value of 0. The value of the array list is then changed to a new value. The substitution entails converting the value from 0 to 13. After an array list modification, the new value is reported to the console.
If any detail is to be removed from the ArrayList, several factor transfers must be carried out, which slows down the operations that control the factors inside the array list.
Besides the default constructor, there are many overloaded constructors for you to create the Java initialize ArrayList. Let’s list down some of the constructor methods
2d Arraylist Java Constructor Methods – 2d Arraylist Java Example
Arraylist()
This function creates an empty Arraylist using the default constructor.
The syntax for this method is as follows.
Your data is classified by its type, such as String in this case. In addition, the name of your Arraylist is list name.
ArrayList (int capacity)
Use Arraylist if you want to create a 2d Arraylist in Java with a defined size or capacity (int capacity)
Its standard syntax is
For instance, you wish to build a new Arraylist with the name Arraylist, a string type, and a 15-item capacity. Then, this is your syntax.
Arraylist ( Collection <? Extends E> C)
This 2d Arraylist method, in contrast to the previous two methods, takes an existing collection as an argument. Consequently, this method can construct an Arraylist with elements, as opposed to the two ways above, which create an empty ArrayList.
Syntax in general for this
Example of 2D ArrayList in Java
let's now see an example of how 2D ArrayList works in Java
Output:
The example demonstrates how to create a two-dimensional array list, add a value to the list, and then attempt to replace the item with another value. Declaring the headers for the two-dimensional array list creation is the first crucial step. In this instance, import java.util.*. A class is then declared. The main function is connected to the declared class. The new array has been declared in the main function. As a result, the array declaration step is included in the main function
The array is declared using the values from the array list. The values are then added to the array list using the add method. With the aid of a new term, space for the 0th row can be allocated, as demonstrated in this line. The 0th row also permits the default storage of a value of 0. The value of the array list is then changed to a new value. The substitution entails converting the value from 0 to 13. Following arraylist updates, the value is then reported to the console.
Program to Create 2d ArrayList in Java
Using Fixed-Size Array
The first approach will produce an arraylist1 with three rows and three columns and the name ArrayList. We will build an ArrayList object in each row and column and add data to it in order to put an ArrayList of Strings into arraylist1.
The example below demonstrates how arraylist [0][0], which is the first row and the first column of arraylist1, are filled first. This process continues until the ArrayList is filled. Here, we are only adding data to the first row; the next two rows are empty, and therefore the output reads as null.
Output:
Creating ArrayList of ArrayList
The following Java method to generate a two-dimensional list will work for us because it is two-dimensional: make an ArrayList of arraylists. We can initialize the 2D ArrayList Java object to outerArrayList1 in order to place an innerArraylist function inside of outerArrayList1.
Adding our data to the innerArraylist function and subsequently the outerArrayList command is the last and last step. Keep in mind that we can use the outerArrayList command to combine multiple ArrayLists.
Output:
Iterating through 2d Arraylist in Java
Using for loop
An index-based loop is an option. The ArrayList's elements can then be printed after traversing the list. This may be the simplest method for iterating through and printing an Arraylist's elements.
We demonstrate this way with the help of this 2d arraylist in Java example.
Output
using for-each loop
The 2D ArrayList Java Example that follows will demonstrate how to navigate and loop through an ArrayList using a for-each loop.
Output:
Using Iterator Interface
You may iterate through the 2d Arraylist and output its value with the aid of the iterator interface.
This is how you do it.
Output
Here, using an iterator, we loop through ArrayListList in this method. All the numbers are first stored in an arraylist, after which the content of the first list is copied into the second arraylist. Following an iteration of this list using an iterator, the contents of the list are printed using the whole method.
An Iterator is an object that can be used to loop through collections, like ArrayList and HashSet.
Using ListIterator Interface
You can navigate both forward and backward using the ListIterator interface.
Output:
Here, we've used the ListIterator to print a list's contents. First, the letters are added using the add() function, which we covered previously in the article. Next, we loop through the list using the ListIterator to print the list's contents as well. In this example, we've used the hasPrevious() function to iterate backward because we know that ListIterator can be used to iterate both forward and backward.
Using forEachRemaining () Method
Let’s consider this 2D arraylist java example below:
Output:
Here, as you can see we've uthe sed forEachRemaining () Method for iterating through this list after storing the characters in letters_list and using an iterator as we've discussed previously
The list is then printed usthe ing forEachRemaining () Method
Conclusion
- Array is a basic functionality provided by Java, ArrayList however comes from Java's collection framework
- The first index denotes the value of the row, while the second indexes denote the value of the column. In the format of a[0][0], a[0][1], etc.
- The Java ArrayList can retain the insertion order in accordance with the value insertion trigger
- The array is declared using the values from the array list. The values are then added to the array list using the add method
- 2D ArrayList java class only contains objects. if we have to store an integer type element we'll need to use integer objects of wrapper class instead of primitive type int