How to Add Property to an object in JavaScript?
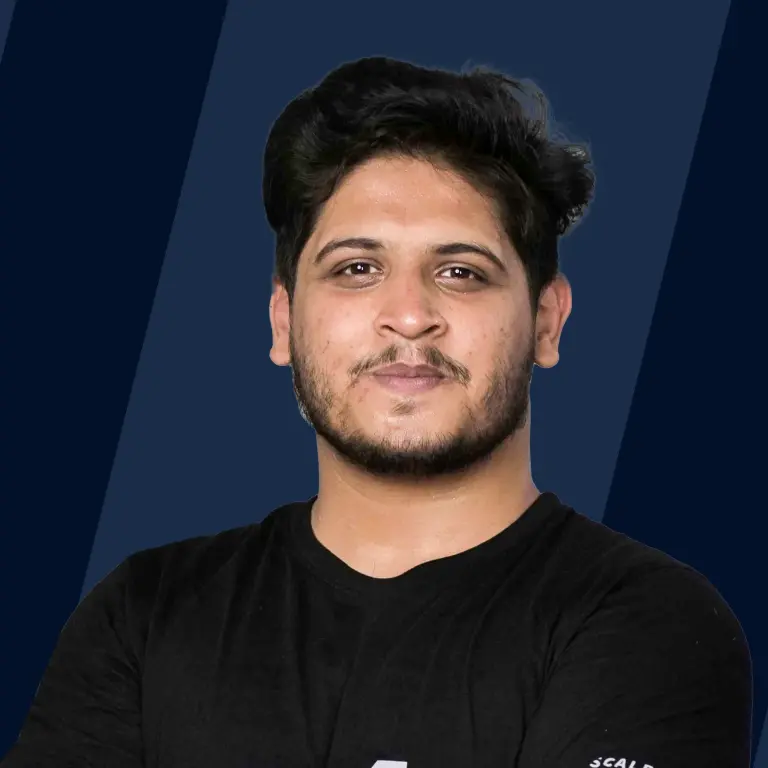
Overview
Object in JavaScript is a collection of properties and these properties are basically key-value pairs. Properties define the characteristics of an object in JavaScript and these can be changed, deleted, and even added dynamically even after the object has been created. Some of the ways to add property to object JavaScript include: using dot notation, using bracket [ ] notation, using defineProperty() method, using spread operator, and using Object.assign() method.
Introduction
To start with, let us revise and go through the concept of objects and their properties in brief. Object in JavaScript is a collection of unordered properties and these properties are basically key-value pairs that define the characteristics of an object. Just like JavaScript variables, object names & their properties are case-sensitive. Let us take an example of an object in JavaScript where we will define some of the properties inside it.
Here, we have created an object named car with brand and year as its properties with assigned values. Now, if we have to access these properties of car object, we can simply use dot notation or square-bracket notation. Note that if we try to access properties of an object which are unassigned, then it will return undefined and (not null).
Have you ever wondered that if it is possible to add more properties to an existing object that has already been created? The answer to this question is yes, we can do so and can add properties to an object in JavaScript dynamically even if the object has already been created.
There are many methods and ways by which we can add properties to an existing object in JavaScript and we will discuss all of them in detail as we move through the course of this article.
- Using dot notation (.)
- Using square brackets notation [ ]
- Using Object.defineProperty() method
- Using Object.assign() method
- Using spread operator syntax
Using Dot Notation (.)
One of the simplest ways to add, access, or modify properties of an existing object in JavaScript is by using dot notation.
Syntax
Here, object denotes existing object name, and to add property to object JavaScript, we are using the dot operator. new_property is basically the key and new_value is the value assigned to this new property that is to be added inside the object.
Note that this dot notation will not work for the cases where the property key name includes special characters like spaces, dashes, digits, etc.
Example to Demonstrate the use of Dot Notation in JavaScript
In the below JavaScript example code, we have created an object named obj with some initial properties defined inside it.
Now to demonstrate the use of dot notation, we are adding two new properties with assigned values inside obj: obj.roll_no = 121 and obj.secondName = 'Jain' with the help of the dot operator.
In the end, we are printing the object obj to check newly added properties (key-value pairs) inside it.
Note that with the help of the dot operator, we can also change the existing properties of an object. Example: obj.name = 'Raj';
Output:
As we can observe, new properties have been added dynamically using dot notation.
Example 2
Note that if we try to use any special character or numeric digit in the property name while adding using dot notation, it will generate a syntax error.
Output:
Using Square Brackets Notation [ ]
As we have discussed above, there are some disadvantages while working with dot notation in cases where the property name is invalid variable identifier (say all digits, having spaces, special characters).
To handle such cases, we can imagine object as an associative array and can use square bracket notation to add new properties inside an object.
Also in case of dynamic variables where property names are retrieved from user inputs or API calls, this square bracket notation is helpful over dot notation in JavaScript.
Syntax
Here, object denotes existing object name, and to add property to object JavaScript, we are using square brackets notation. new_property is basically the key and new_value is the value assigned to this new property that is to be added inside the object.
Example to Demonstrate the Use of Square Brackets Notation in JavaScript
In the below JavaScript example code, we have initially created an object named obj with some defined properties. Clearly, as we already know now that using square brackets notation also, we can access, add and even modify the existing properties.
To start with, we modified existing property of object obj i.e, obj['state'] = 'Mumbai'. We then created an empty nested object (obj.city = {}) within obj. We can also access nested objects using square bracket notation just like accessing a 2D array.
We have also showcased the combinational use of the dot operator and square brackets to access nested objects.
Output: As we can observe that the object obj has been displayed here with modified 'state' property and a newly added nested object 'city' with name and street properties.
Example 2
Taking another example to understand the advantages of square brackets notation, here we have an empty JavaScript object obj and to add properties inside it, we are running for-loop.
The property name is an expression that has to be evaluated on each loop iteration depending on the value of 'i' (when i==1, obj['num1'] = 1;), clearly helpful in case of dynamic property names.
Output:
Using Object.defineProperty() Method
JavaScript object class provides the defineProperty() method and using this, we can modify an existing object or add property to object JavaScript.
This method returns the modified object and also allows us to control or configure the behavior of the properties.
Syntax
Here, the configuration consists of majorly two properties which we can manually set: enumerable and writable.
If writable is set to false, it means that we can't modify or set a new value for this particular property of object whereas enumerable denotes that the value has to be retrieved dynamically using either for-loop or user-input, etc. Let us understand this theory practically seeing example codes.
Example to Demonstrate Use of defineProperty() Method in JavaScript
In the below JavaScript example code, we have initially taken an empty object named obj. To add properties inside it, we have used Object.defineProperty() method to add 'id' property to obj and set its configuration property - writable as 'false'.
Next, we tried modifying the 'id' property of obj but due to the writable configuration which was set to false, there is no effect on the value of object 'id'.
Output:
Using Object.assign() Method
There is another interesting way by which we can add property to object JavaScript i.e, by using the Object.assign() method. This method allows us to add properties of one source object to another target object. We can just define all those properties inside the source object that we need to add to an existing target object.
Note that the properties inside the target object will be overwritten by the source object’s properties if the same key name is found in both the objects.
Syntax
Here, the Object.assign() method of JavaScript has been used and source & target denotes that the properties of the source object will now be added or assigned to the target object as well.
Example to demonstrate the use of Object.assign() Method in JavaScript
In the below JavaScript example code, we have two objects student and info with already defined properties inside them. Now our task is to add properties of the info object to the student object and for this, we can use Object.assign() method by passing student and info as parameters respectively.
Output:
Using Spread Operator (...)
There is another unique way to add property to object JavaScript by using the spread operator. Spread operator (...) creates a copy of the existing object with all its properties and therefore, using inline property definition, we can further add more properties to that object. Let us look at the syntax to understand this in a better manner!
Syntax
Here, ...object virtually creates a copy of existing properties of object, and using inline definition, we can add more properties (property1, property2....) to the existing object.
Also, the spread operator allows us to merge the properties of two objects into a new object.
Note that this approach of using the spread operator to merge two objects to form a new one, does not overwrite existing properties of the object if common keys are found in both source and target objects.
Example to Demonstrate the use of Spread Operator Method in JavaScript
In the below JavaScript example code, we have an object named obj with already defined properties inside it. We will now check the functionality of the spread operator using which we can add more properties inside obj.
We will use the spread operator on object obj and using inline definition, we will add some more properties ('nationality', 'gender') inside obj. To check the resultant object, we have displayed the object values in the end.
Output:
Conclusion
- Properties define the characteristics of an object in JavaScript and these can be changed, deleted, and even added dynamically after the object is created.
- Just like JavaScript variables, object property-names are also case sensitive.
- Some of the ways to add property to object JavaScript include:
- using dot notation**
- using bracket [ ] notation**
- using defineProperty() method**
- using spread operator**
- using Object.assign() method**
- Dot notation method works ideally for static property name values without any invalid identifier.
- Square bracket notation works best when values are to be retrieved by user input, for-loop, or by API calls dynamically to set property names.
- Object.defineProperty() method is useful when we need to control or configure the behavior of properties of an existing object in JavaScript.
- Spread operator syntax method is useful when we want to assign or add values from the target object to the source object by making a copy of the actual given object.
- We can also pass the target object and the source object as parameters to the Object.assign() method to add or assign the values of one object to another object.