Anonymous Class in Java
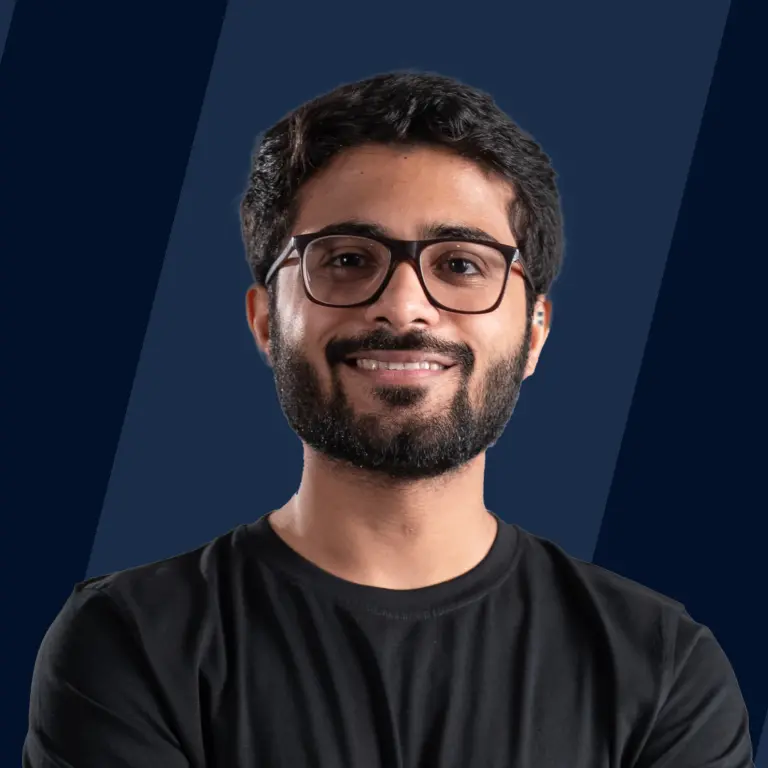
In Java, an anonymous class is a local class without a name that can be used to define and instantiate a class simultaneously. An anonymous inner class has no name and produces only one object.
Anonymous classes can be useful for providing quick implementations of interfaces or abstract classes in situations where creating a named class would be overly lengthy or cumbersome.
Introduction to Anonymous Class in Java
In Java programming, we can create classes that can contain other classes nested in it. We can also create nested classes without giving any name to it.
An Anonymous Class is a nested class without a name. It must be defined within another class, which is why it is also known as an Anonymous Inner Class in Java.
An anonymous inner class can be helpful when creating an instance with specific "extras" without having to subclass a class. The "extras" are overloading methods of a class or interface that the instance extends.
Syntax of Anonymous Class in Java
An Anonymous inner class must be defined inside another class:
Here, SuperType can be a class or interface type that the anonymous class extends or implements, and constructorArguments are the arguments to the superclass's constructor.
Types of Anonymous Class in Java
Anonymous classes are typically used to extend subclasses or implement interfaces. So, there can be two types of anonymous classes in Java:
- An anonymous class that extends a superclass.
- An anonymous class that implements an interface.
Example: Anonymous Class Extending a Class
Let's see an example of an anonymous class that extends a class.
In the example below, we have created a class Demo. It has a single method, view(). We then created an anonymous class that extends the class Demo and overrides the view() method.
When we run the program, an object d1 of the anonymous class is created. The object then calls the anonymous class's view() method.
Output:
Example:
Let's see an example where the anonymous inner class is defined inside the argument of a constructor call.
Output:
Explanation:
- In this example, an anonymous inner class defines a Runnable implementation for a new Thread instance. The Runnable interface provides a single run() method that is called when the thread is started.
- The Thread instance is started using the start() method, which causes the anonymous inner class's run() method to be executed within a new thread of execution.
Example: Anonymous Class Implementing an Interface
We can have an anonymous inner class that implements an interface. Let's see this using the example given below.
Output:
Explanation:
- The Demo interface declares a single method called view(). The MyClass class has a method called myMethod(), which creates a new instance of an anonymous class that implements the Demo interface.
- The main() method of the Main class creates an instance of MyClass and calls its myMethod() method. This causes the anonymous class to be instantiated and its view() method to be called, which prints the message "Inside the Anonymous Class" to the console.
Advantages of Anonymous Classes
- Objects in anonymous classes are created only when they are necessary. Objects, in other words, are built to accomplish certain jobs like instantiating a class.
- Anonymous classes makes our code more concise as we dont have to write class definition again and again, we can just extend other classes when needed.
- Anonymous classes enable you to declare and make instance of a class at the same time.
- Anonymous classes can save much time and reduce the effort to make number of .java files necessary to execute an application.
Conclusion
- An anonymous inner class in Java is one that does not have a name and produces only one object.
- An anonymous class must be defined inside other class. Hence, Anonymous class is also called Anonymous Inner Class in Java.
- There can be 2 types of anonymous classes in Java:
- An anonymous class that extends a class.
- An anonymous class that implements an interface.