apply() in JavaScript
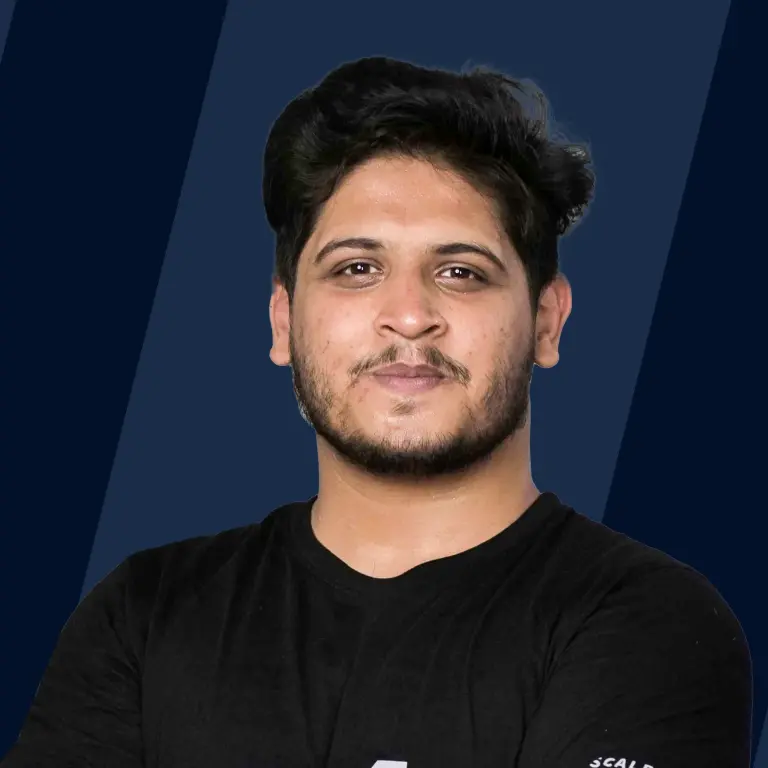
Overview
The apply in Javascript is used to call a function in a different object with the given this value, and the arguments are passed in the form of an array. This method allows us to write methods that can be used on different objects and hence increase the reusability of code.
What is apply() in Javascript?
The apply in Javascript is used when we need to call a method on object x with a different object y.
Example:
Output:
Explanation: In this example, we duplicated the drive() method on two objects, car and bike. Instead, we can use car.drive.apply(bike) to call the apply() method of car with the bike object.
Syntax of apply() in Javascript
The apply in Javascript accepts two arguments, the this value (object on which the function is to be called) and the list of the arguments (optional) that will be passed to the method it calls (fn).
Parameters of apply() in Javascript
- thisArg - The value of this that will be passed to the method fn that will be called by apply.
- argumentsList (optional) - The list of arguments that should be passed to the method fn.
Return Value of apply() in Javascript
The value that is returned by the method that the apply() method calls with the given thisArg and argumentsList.
Exceptions of apply() in Javascript
The apply() in Javascript doesn't thrown any generic exception when it is called. But it may throw exceptions based if we pass any invalid inputs.
Example: The apply() method throws Uncaught TypeError: second argument to Function.prototype.apply must be an array if any non-array value is passed as the second argument.
Output:
Examples
1. apply() Method to Call a Function
In this example, we will call a method using apply() for different input objects.
Output:
Explanation: We call the intro() method for the two objects student1 and student2 with different arguments. The this inside the intro() method refers to student1 for the first apply call and student2 for the second apply call.
2. apply() for Function Borrowing
Function borrowing allows one object to borrow a method of another object. In this example, we use the specs() method of the mobile object on the laptop object.
Output:
Explanation: We used the specs() method of the mobile object to print the details of the laptop object using the apply() method. The apply() method calls the specs() method of object mobile with the laptop object passed in the argument.
3. apply() to Append two Arrays
Given two arrays, array1 and array2, we want to append array2 at the end of array1. For example, if the inputs are [1, 2, 3] and [4, 5, 6], the output should be [1, 2, 3, 4, 5, 6]
Output:
Explanation
- Case 1 - When executing array1.push(array2), the entire array2 is appended to the last of array1.
- Case 2 - When executing array1.push.apply(array1, array2), all the individual elements of array2 are appended one by one to the end of array1. We are passing array1 as an argument because it will be used by the apply() method with the this reference to append array2 in the last. The internal call may look likethis.push(array2[0], array2[1], array2[2])
4. apply() with Built-in Functions
Given an array of numbers, find the maximum number using Javascript's Math.max() method. Let's pass the given array to the Math.max() method.
The output of the above code will be NaN because the Math.max() method does not accept an array as input. Instead, each value must be passed as an argument to get the correct maximum value.
The above code returns 5, which is the maximum value. This maximum value can be found for the given input array using the apply() method.
Output:
Explanation We called the Math.max() using the apply() method with the values array as an argument. The first parameter, thisArg, is not required for this method. So, we passed the null value.
Behaviour of apply() in Javascript: Strict vs Non-Strict Mode
The behaviour of the apply() method in Javascript changes between strict and non-strict mode. The strict mode can be enabled in Chrome by adding 'use strict;' on the top.
ECMAScript 5 and later let scripts opt in to a new strict mode, which alters the semantics of JavaScript in several ways to improve its resiliency and which make it easier to understand what's going on when there are problems. MDN Web Docs
- In strict mode, if null or undefined is passed as the first argument to the apply() method, it will use the value as it is.
- In non-strict mode (default), if null or undefined is passed as the first argument to the apply() method, it will be replaced with the global object.
Example: non-strict mode
If the above code is executed on the Chrome console, it will print the window object as it is the global object
strict mode
The above code prints null which is the value passed as an argument.
Conclusion
- The apply in Javascript is used to call a function with the given this (Object) value, and the arguments passed in the form of an array
- The apply in Javascript is used when we need to call a method on object x with a different object y.
- The apply() method is used for different use-cases like function borrowing, appending two arrays, etc.