Array of Objects in Java
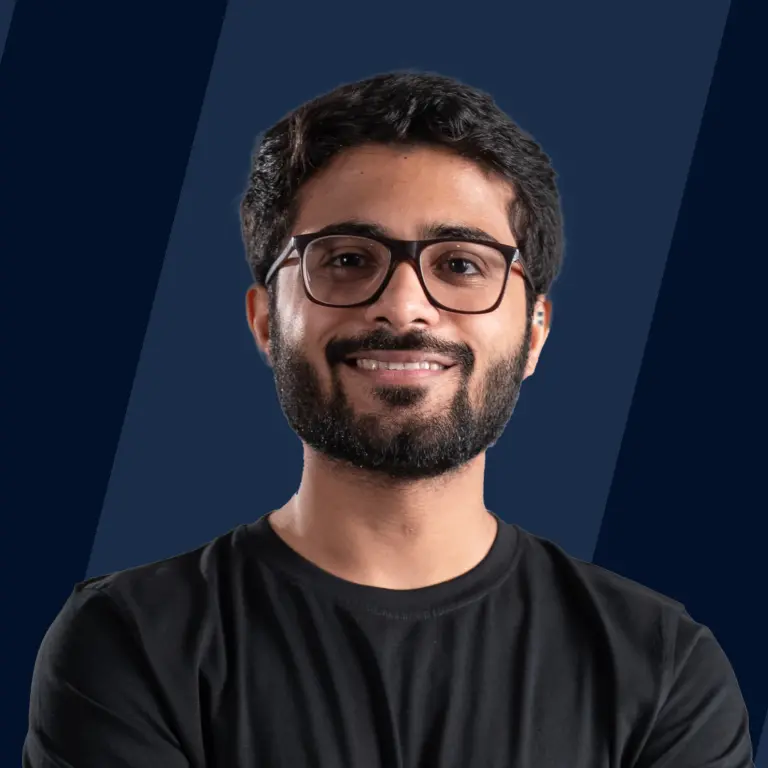
Java emphasizes object-oriented programming, relying heavily on classes and objects. While a single object can be managed using an Object variable, multiple objects are efficiently handled using an Array of Objects. Unlike typical arrays that store primitive types like integers or booleans, an Array of Objects accommodates object references. In Java, classes serve as user-defined data types, and when elements of such a type are stored in an array, it's termed an "array of objects." Essentially, this approach facilitates organized and dynamic management of multiple class-based entities within Java programs.
Create an Array of Objects in Java
An array of objects in java is created with the help of the Object class. The Object class is kind of the root class of all the classes.
To create an array of objects, we use the class name which is followed by a square bracket([]), and then we also provide the variable name. Below given is the syntax for creating an array of objects in Java.
Syntax:
Note: Here, we have used the class name followed by square brackets, and then we have provided an object name objectArrayReference.
Apart from that, there is one more way through which we can create the array of objects in Java. Here, we write our class name, and as usual, we were writing. But it is followed by the variable name, and then we write our square brackets.
The major point to be noted here is that, in both the above declarations, we see that objectArrayReference is an array of objects.
For example, suppose you have a class Groceries then we can create an array of Groceries objects as given below:
Explanation:
In the above example, we are storing the class Groceries object reference in the variable groceriesObjectsReference in the array.
Instantiate the Array of Objects
To instantiate an array of objects in java we usually use the ‘new’ keyword. This we do before using the array of objects in our program.
Syntax
For example, if we have a class called Groceries, and we want to declare and instantiate an array of Groceries objects with two objects/object references then it will be written as:
After instantiating the array of objects like we did above, we create the individual elements of the array of objects by using the new keyword.
The below figure shows the structure of an Array of Objects :
Initializing Array Of Objects
After declaring the array of objects, we have to initialize it with values. The initialization of arrays of objects is done differently from the initialization of primitive types.
It is mandatory to initialize each element of an array of objects. By this, we mean that each object or object reference must be initialized. An array of objects contains references to the actual class object. Hence, after declaring the arrays of objects and instantiating them, we create the actual objects of the class.
There are different ways to initialize the array of objects. They are stated below:
- Using the constructor
- Using a separate member method
Let us now look briefly into each of the above ways.
1. By Using the Constructor
When we are initializing or creating the actual object, we can assign initial values to each of the objects by passing values to the constructor separately. The actual objects are individually created with their distinct values.
The below program shows how the array of objects in Java is initialized using the constructor.
Output:
Explanation:
In the above code, we have created the Groceries class, in which we have passed the product and price as attributes. Also, we have created the constructor of the Groceries class to set the data to the Groceries object, and it also has a display() method to display the Groceries data.
Now, in the main class, we are declaring an array of Groceries data-type, then we instantiate the array of objects with the new keyword and allocate the memory for two objects. After that, we initialize the object's reference of the array by passing the attributes data in the constructor of the Groceries class with the new keyword. Finally, we display the required data.
2. By Using a Separate Member Method
We can also initialize the array of objects in java with the help of the separate member method. By separate member method we mean the member function or method we create inside the respective class. that member method is used to assign the initial values to the objects in the array.
The below program shows how the array of objects is initialized using a separate member method.
Output:
Explanation:
In the above code, we have created the Groceries class, in which we have passed the product and price as attributes. Here we also have a separate member method in the Groceries class to set the data to the Groceries object, and it also has a display() method to display the Groceries data.
Now in the main class, we are declaring an array of Groceries data type, then we instantiate the array of objects with the new keyword and allocated the memory for two objects. Then we initialize the object's reference of the array data with the new keyword. This time without sending any attributes in the constructor of the Groceries class, as there is no parameter constructor, except the default one. So we assign the data in the Groceries object by calling the separate member method set-data. After storing the data we display the data.
Example Program for an Array of Objects in Java
Now, we already know what is arrays of objects in java, now let us take a relevant example to enhance your understanding.
Code:
Output:
Explanation:
In the above code, there is a Student class with name and age as an attribute. Here we also have a separate member method setData in the Student class to set the data to the Student object, and it also has a display() method to display the Student data.
Now, in the main class, we will have seen how we can individually store the object reference in a variable, and also how we can declare an array to store the object's reference in it. Then we repeat the same process of declaring, instantiating, initializing, and displaying the data in both variable and array.
How to Sort an Array of Objects in Java?
The array of primitive data types is sorted by using the sort method of the Arrays class. We can also sort the array of objects by using the sort method of the Arrays class.
But in the case of an array of objects, the only difference here is that the class to which the objects belong should implement the ‘Comparable’ interface so that the array of objects is sorted. You also need to override the compareTo method, Since the object doesn't contain any primitive data type so the inbuilt compareTo method will not be able to sort the elements on its own, so by overriding compareTo we will ask the compiler to forget the previous compareTo implementation and sort elements according to the provided new information. By that, we will be able to sort the array of objects based on any field we decide. The array of objects is sorted in ascending order by default.
Now you must be thinking, what is Comparable interface and compareTo method, so before looking at the code, let us learn a bit about both of them.
- Comparable interface: The main purpose of the Comparable interface in java is to sort the objects of any user-defined class. It is used to provide them with a particular order. The comparable interface is from the java.lang package. It consists of only one method - compareTo(Object). The compareTo object helps to sort based on a single data member. It can, for example, sort according to price, height, etc.
- compareTo method: The compareTo method in java deals with the comparison of the current object concerning the specified or the other objects. It returns three things based on the object passed to it. They are stated below:
- It returns a positive integer, if current Object > specified Object
- Returns a negative integer if, current object < specified object
- And, it returns a ZERO if the current object == specified object
Code:
Output:
Explanation:
In the above code, there is a Student class that implements the Comparable interface for sorting the objects. It has name and age as the attributes. Here it also has a separate member method setData in the Student class to set the data to the Student object, and it also has an overridden function string which will convert the array of objects to string, it also has a compareTo method overridden for sorting array of objects in ascending order concerning the age attribute.
Here the compareTo method is returning this.age - o.age, let us understand this clearly --
- The this. age is the age of the current object which is being compared,
- And, the o.age is the age of other specified objects.
If the compareTo returns positive integer then, that means this.age ( current ) object is greater than o.age( specified ) object. Naturally, the current element shifts right, since it is large and the default sorting order of the comparable interface is to be maintained.
Otherwise, if the compareTo returns negative integer, then that means this.age( current ) object is less than o.age( specified ) object. Hence, the current element automatically shifts to the left (to be sorted in ascending order).
Finally, in case compareTo returns zero that means both this.age( current ) and o.age( specified ) objects are same. Here, there is no change in the order of the elements.
By this, we can sort the arrays of the object in ascending order. For descending order we can do vice-versa, i.e. o.age - this.age.
Now, in the main class we have already seen how can we individually store the object reference in a variable. Also, we have seen how can we declare an array to store the object's reference in it. Then we repeat the same process of declaring, instantiating, and initializing. After that, we sorted the array using the sort method of the Arrays class in Java. Finally, we display the string array in both original and sorted order.
Conclusion
In this article, we learned about the array of objects in java. Let us recap the points we discussed throughout the article:
- Java programming language is all about objects as it is an object-oriented programming language, and we can store multiple objects.
- If we want to store a single object in our program, then we can do so with the help of a variable of type object. But when we are dealing with numerous objects, then it is advisable to use an array of objects.
- Java is capable of storing objects as elements of the array along with other primitive and custom data types. Note that the ‘array of objects’, signifies references to the object.
- An array of objects is created using the ‘Object’ class.
- We need to instantiate the array of objects using the new keyword before being used in the program.
- We initialize the array Of objects, which can be done in two ways either using the constructor or by using a separate member method
- By using the constructor, when we create actual objects, we can assign initial values to each of the objects by passing values to the constructor.
- By using a separate member method, we can have a separate member method in a class that will assign data to the objects.
- We have also learned to sort an array of objects in java using comparable interface and compareTo method. There we have seen how the compareTo method returns by comparing the current object field with the specified object field(this. field - o.field) for sorting in ascending order, and comparing the specified object field with the current object(o.field - this. field) for sorting in descending order.