What is an Array of Strings in C?
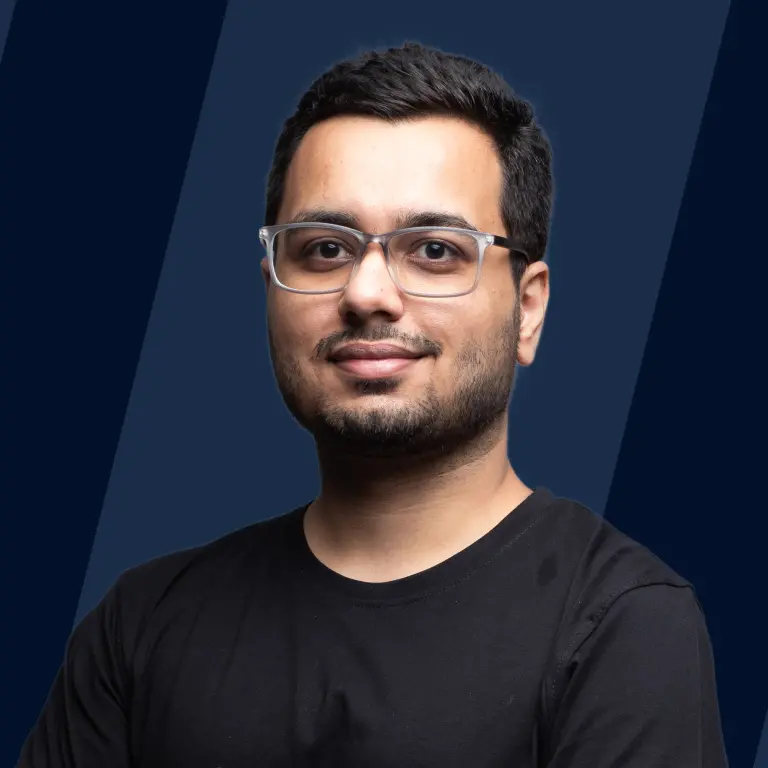
In C, an array represents a linear collection of data elements of a similar type. Thus, an array of strings means a collection of several strings. The string is a one-dimensional array of characters terminated with the null character. Since strings are arrays of characters, the array of strings will evolve into a two-dimensional array of characters.
Syntax
It is possible to define an array of strings in various ways, but generally, we can do so as follows:
Initialization of Array of Strings
Initialization in C means to provide the data to the variable at the time of declaration. It is straightforward to initialize an array of strings. We can create an array of some size and then place comma-separated strings inside that. See the example below to get a good idea about it.
Explanation:
In this example, we have initialized a two-dimensional character array car with a maximum of 5 strings of size 20.
Strings as an Array of Characters
As we know, C strings are nothing but an array of characters. Therefore, we can initialize the string as an array of characters instead of directly writing it in double quotes. Both approaches are the same. In the latter scenario, C interprets the string similarly and appends a null character at the end.
We can also create our array of strings with this approach of writing strings as an array of characters. Let's re-write the same initialization,
Example of Array of String in C
Reading and Displaying Array of Strings
Output:
Explanation:
- We have created an array of strings with a fixed size of 5 and initialized that array with the strings at the time of declaration.
- Subsequently, we loop in a nested manner, in which the outer loop accesses the array item, and the inner loop prints the characters of that string until the end.
Functions of Strings
Several functions help us while manipulating strings in C. Some of them are described below.
strcat() Function in C
This function is used to concatenate two strings.
It accepts two pointers, one to the base address of the destination string and the other to the source string, then concatenates the source to the destination and returns the concatenated destination string.
strlen() Function in C
This function is used to find the length of the string.
It accepts a string and returns the size.
strcmp() Function in C
This function is used to compare two strings.
It accepts two pointers to the strings and compares them. If they are identical, it returns 0; otherwise, it returns a non-zero value.
strcpy() Function in C
This function is used to copy a string.
It accepts two pointers to the destination and source string and copies the characters of the source to the destination. Subsequently, it returns the pointer to the destination string.
strrev() Function in C
This function is used to reverse a given string.
It accepts a string and performs a reverse operation, finally returning the pointer to the exact string.
Some Invalid Operation on an Array of String
1. If we access a memory block that doesn't exist or is out of limits, it will contain some garbage, so we should always access the memory according to its limit. All those operations in which we access invalid memory will be called invalid operations in an array of strings.
2. The strings pointed by a pointer( in an array of strings having type char*) are internally translated as constant char array or read-only, so you cannot change them. That's why all operations that try to modify the string by its pointer will be invalid.
An important point to note that might create confusion is that you cannot modify a constant string, but you can always change the pointer value to point to another string.
Output:
Apart from the strcpy, using functions like strcat, gets, fgets, and scanf to modify these types of strings will also be invalid.
3. When we write an array of strings with 2D array notation, the pointer becomes constant. We cannot change it to point to another string.
Output:
The above code gives an error as the array's name is a constant pointer, and we are trying to change it. But we can change the content of that string.
Output:
In the above code, we change the content of the string pointer to arr[0], which is valid as it still points to the same location.
How Does Array of Strings Work in C?
Strings in C are collections of characters, more formally arrays of characters terminated by a null character. The last character will be 0 to determine that it is the end of the character array. So, the array of strings works similarly. It is just a two-dimensional character array.
Methods about How to Declare an Array of Strings in C
Use 2D Array Notation to Declare Array of Strings in C
As we know, strings are nothing but an array of characters; hence, the array of a string would be a two-dimensional array of characters. We can use a 2D character array to declare an array of strings in C.
Use the char* Array Notation to Declare Array of Strings in C
Before discussing this approach, let's understand what * means. When we write the * in front of the data type, it becomes a pointer, which can point to the memory block of the same data type. Now, if we assign the array to the pointer, it points to the array's base address. This means we can store the array's base address in the pointer and then traverse the entire array. So char* creates a pointer pointing to the string's base address. To declare an array of strings, we can create an array of these kinds of pointers. See the example below for a better illustration.
An important point to note is that internally, this pointer to string notation is translated as const character array, which means you cannot modify them.
Output:
Conclusion
- An array of strings in C is a one-dimensional array of strings and a two-dimensional array of characters.
- We can declare the array of strings using the pointer method (char*) or 2D notations.
- The initialization of the array of strings is simple: we can either provide a string wrapped inside double quotes to create an array of pointers or write a nested array of characters.
- When we create an array of strings using the char* notation/pointer approach, all strings are internally interpreted as a constant character array, so we cannot change them later. However, in the case of an array of strings using 2D notations, the string pointers are constant, so we could change their content but not the address to which they point.
- We cannot access invalid or out-of-bound memory while accessing strings, which could create undefined behaviour or errors.