Converting Array to List in Java
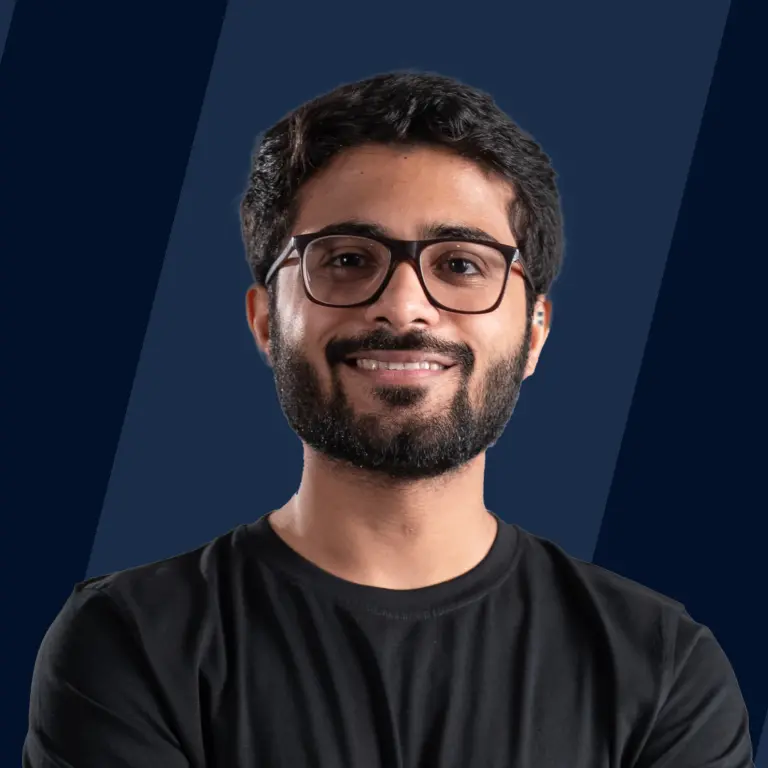
Convert the given array to a list in Java.
Examples:
Output:
Arrays: An array consists of elements of the same type, all identified by a single name. It can hold both primitive data types and class objects, depending on how it's defined. For primitive types, their values are placed next to each other in memory. Class objects are located in a specific area of memory known as the heap.
List: The List interface in Java, part of the java.util package, extends the Collection framework. It represents an ordered group of elements, including duplicates, and maintains the sequence in which elements are added, enabling element retrieval and addition at specific positions. Classes such as ArrayList, LinkedList, Vector, and Stack provide concrete implementations of the List interface.
Some methods for transforming an array to list in Java are:
- Brute Force or Naive Method
- Using Arrays.asList() Method
- Using Collections.addAll() Method
- Using Java 8 Stream API
- Using Guava Lists.newArrayList()
Brute Force or Naive Method
This is the most straightforward approach to converting an array to a list in Java. In this method, an empty list is first converted, and the array elements are added to the list.
Algorithm:
- Obtain the array that needs to be transformed.
- Initialize an empty list.
- Loop over the elements in the array.
- Add each element to the list during the iteration.
- Provide the completed list as the output.
Example:
Output:
In this simplified example, we convert an array to a list in Java using a straightforward method. We define a function arrayToList() that accepts an array and returns a list by iterating over the array with a for loop and adding each element to a newly created list. The main method declares a string array newarray, converts it to a list finallist using arrayToList(), and then prints finallist. This method, while direct, is less efficient due to the manual element-by-element addition.
Using Arrays.asList() Method
This is a direct approach used to convert an array to list in Java. In this approach, the Array is passed as the parameter to the List constructor using Arrays.asList() method of the Arrays class.
Algorithm:
- Retrieve the array intended for conversion.
- Generate the list by passing the array into the list constructor, utilizing the Arrays.asList() method.
- Deliver the resultant list.
Example:
Output:
Another way of converting an array into the list is by using the Array.asList() method of the Arrays class in Java. Here, in the main method, an array named newarray is declared to store string elements. And this newarray is passed as a parameter to the function Arrays.asList() which converts this array into a list, and is stored in a list named finallist. Finally, the finallist is printed, our desired output.
Using Collections.addAll() Method
As we know, List is a part of the Collection framework; we can use methods of the Collection framework to convert Array into List. This approach will convert the array to the list using the Collections.addAll() method.
Collections.addAll() method uses list and array as parameters. It adds all the elements from the list into an array.
Algorithm:
- Retrieve the array intended for conversion.
- Initialize a blank list.
- Incorporate the elements of the array into the list by using them as arguments in the Collections.addAll() method.
- Supply the assembled list as the result.
Example:
Output:
In this example, we have used a direct approach to convert an array into a list using a predefined Collection framework method. Here, we have created a function arrayToList(), which takes an array as a parameter and returns a list. This function creates an empty list list1. The Collections.addAll() method takes both list1 and the array as parameters and adds all the elements from the array into the list sequentially.
In the main method, an array named newarray is declared to store string elements. And this newarray is converted into a list by calling the function arrayToList() and stored in a list named finallist. Finally, the finallist is printed, our desired output.
Using Java 8 Stream API
Introduced in Java 8, the Stream API facilitates the processing of object collections through a chain of methods, delivering outcomes without altering the original data. In this case, Arrays.stream(Object[]) is employed to obtain a stream.
The Collectors.toList() method collects the input elements into a new list and returns them in the form of a collector.
Algorithm:
- Retrieve the array for conversion.
- Transform the array into a Stream.
- Utilize Collectors.toList to convert the Stream into a List.
- Accumulate the resultant list with the collect() function.
- Provide the assembled List as the result.
Example:
Output:
In the above example, various Stream and Collectors class methods are used; let's understand their functionality.
- Stream.of(): This method returns a Sequential Stream with the specified elements.
- Stream.map(): This method returns a stream that consists of the results of applying the specified function to the elements of this stream.
- Stream.collect(): This method returns the results of operations performed on the stream.
- Collectors.joining(): This method of Collectors Class combines various elements of a character or string array into a single string object.
- Collectors.toList(): This method of the Collectors Class is a static (class) method. It returns a Collector Interface that gathers the input data into a new list.
In this example, the Stream API transforms an array into a list through the arrayToList() function, which takes an array as input and returns a list. The Arrays.stream(array) method converts the array into a stream. The array newarray, containing string elements, is converted into a list named finallist by invoking arrayToList(), and the resulting list is then printed as the final output.
Using Guava Lists.newArrayList()
Lists.newArrayList() is a method of Lists class that belongs to com.google.common.collect package. com.google.common.collect is known as the Guava Package. This Lists class of the Guava package has a method newArrayList that creates mutable empty ArrayList object having elements of the given array.
Algorithm:
- Retrieve the array intended for conversion.
- Initialize a blank list.
- Incorporate the array into the list by supplying it as an argument to the Lists.newArrayList() function.
- Deliver the compiled list as a result.
Example:
Output:
In this example, the com.google.common.collect library converts an array to a list in Java. A function named arrayToList() is defined to take an array as input and return a list, using Lists.newArrayList() to transform the array into an ArrayList. The main method initializes a string array newarray, which is then converted to a list finallist using arrayToList(), and the resulting list is printed as the final output.
Conclusion
- The Brute Force or Naive Method method can be less efficient for large arrays.
- Arrays.asList() Method offers a quick and direct way to convert an array to a list. However, the resulting list is backed by the original array and does not support adding or removing elements.
- Collections.addAll() Method facilitates the addition of all elements from an array to a list.
- Java 8 Stream API leverages the Stream API for a more functional programming approach.
- Lists.newArrayList() utilizes the Guava library's utility method to create a new ArrayList from an array.