Array to String In Java
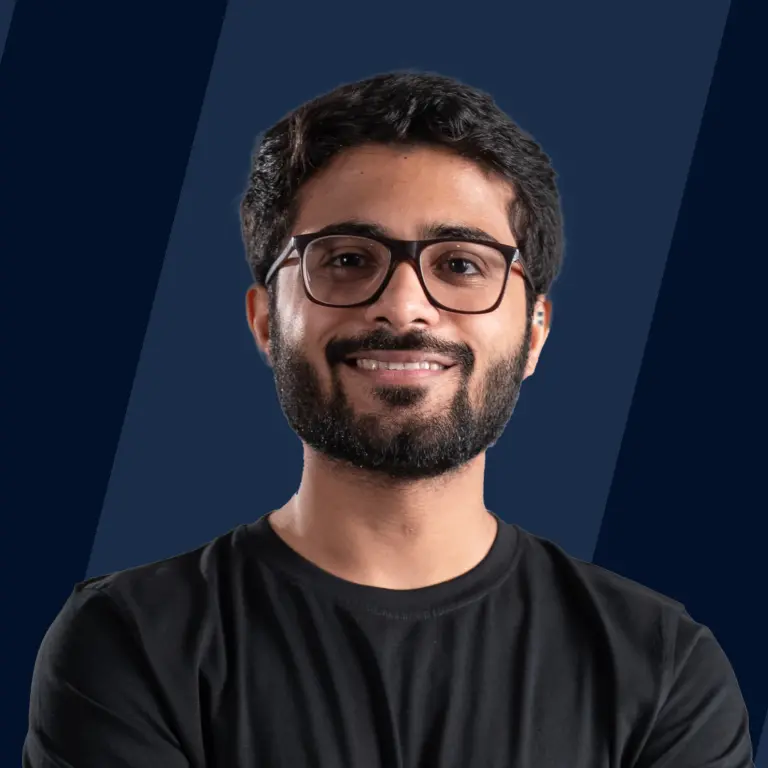
Overview
In Java programming language, an array is a data structure that stores the same type of value in a contiguous memory location which we can access using the index, whereas a string is an object that stores a sequence of characters, so there can be some situation where we want to convert an array to string, so for this we have some methods like toString, StringBuilder append, etc. Using these methods we can convert our array to a string object.
How to Convert an Array to String in Java ?
In java programming language we sometimes need to convert an array to a string, so for this, we have some methods using which we can convert a given array to a string. These methods are explained below with the help of examples.
Example of a situation where we want to convert an array to string :
If we have an array (stuname) of size two that contains the firstname and lastname of a student and we want to convert this array into a single string, then we can use the methods explained below to convert this array into a string.
Arrays.toString() Method :
The Arrays.toString() method is one of the most used methods to convert an array to a string. In the Arrays.toString() method we pass the name of the array that we want to convert to string, and this method returns the content of the array in string form, the values are separated using comma(,) and all these values are enclosed using a square bracket([]).
Example :
Output :
Here in this example, we pass the name of the array as a parameter to the method Arrays.toString() and it returns the string.
The space and time complexity of this method is , where n is the length of the final string.
StringBuilder append(char[]) :
We can also use StringBuilder append() method to convert the array to string. This method java.lang.StringBuilder.append(char[] arr) is the inbuilt method that takes an array as an argument and converts the array representation to a string.
Example :
Output :
The space and time complexity of this method is , where n is the length of the final string.
Java Streams API :
We can use java stream API to convert an array to a string. In java 8 and above versions of java we have String.join() method which creates a new string by joining elements and separating them with a specified delimiter.
Note :
-
The java stream API is used to operate on collections of objects. The stream is a series of objects that support different methods(like map, filter, etc) that can be used to get the desired results.
-
A delimiter is a character or a set of characters that define the start or end of separate elements. This character is used when joining the array elements to create a string, in our example, the delimiter used is an empty string(“”). So when the elements of the array are joined together using the Joiner.join() method the elements will be separated using an empty string. Here in this case we use an empty string as the delimiter.
Example :
Output :
Here in this example, we are converting an array to a string using java stream, here the specified delimiter is an empty array. Hence we pass an array and it returns a string after joining every element.
The space and time complexity of this method is , where n is the length of the final string.
StringUtils.join() :
We can use StringUtils.join() method of the StringUtils class from the Apache Commons Lang library to convert an array to a string. It takes an array, joins the array's elements together, and returns a string.
Example :
Output :
Here in this program, the StringUtils.join(names,“”) method takes two arguments first is the name of the array here it is “names” and the second argument is the delimiter, a delimiter is used between two elements when joining them together, in our example, the delimiter used is a " "(a string containing a single space only). We used " " as a delimiter so that when joining the elements together a space comes between the elements, we can also use other things as the delimiter a blank string.
The space and time complexity of this method is , where n is the length of the final string.
Joiner.join() :
We can convert an array to a string in java using the joiner.join() method which is provided by the Guava library, it is very simple to use, we can also add a delimiter, in the below example, the delimiter is an empty string.
A delimiter is a character or a set of characters that define the start or end of separate elements. This character is used when joining the array elements to create a string, in our example, the delimiter used is an empty string(“”). So when the elements of the array are joined together using the Joiner.join() method the elements will be separated using an empty string.
Example :
Output :
The space and time complexity of this method is , where n is the length of the final string.
Conclusion
In java, we sometimes need to convert an array to a string, for this we have some methods through which we can convert an array to a string.
The methods which we can use to convert an array to a string are :
- Arrays.toString() method : We pass the array name and it returns a string.
- StringBuilder append(char[]) : StringBuilder.append() also takes an array name and return a string.
- Java streams API : Java stream API have the String.join() method in java version 8 and above, this method takes two input parameter first is a delimiter and the other is the array.
- StringUtils.join() : The apache commons lang library provides StringUtils class which provides the StringUtils.join() method which takes two arguments array name and delimiter and converts the array to a string.
- Joiner.join() : The Guava library provides Joiner.join() that takes the name of the array and delimiter character and returns a string.