Arraylist to Array Java
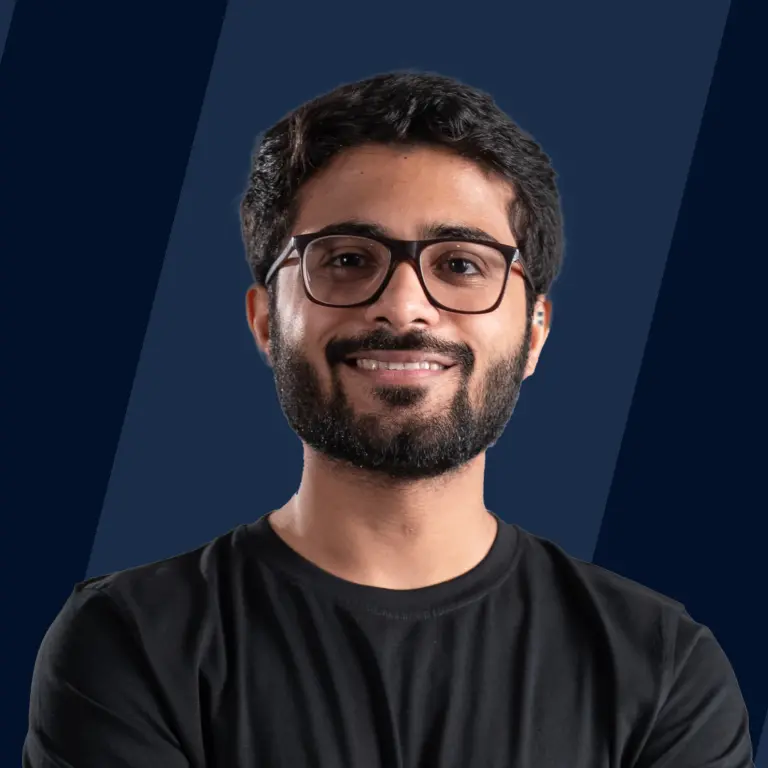
Java's data structures, Arrays and ArrayLists, serve to store multiple items of a single type. Arrays are fixed in size and unchangeable after declaration, while ArrayLists are resizable, offering flexibility. Key to applications and systems are these linear structures, with Arrays as Object class instances and ArrayLists from Java.util's collection framework, have distinct roles. This article explores how to seamlessly convert an ArrayList into an Array in Java, demonstrating Java's adaptability and the importance of choosing the right structure for specific needs.
How To Convert ArrayList To Array In Java ?
Elements in ArrayLists are objects of wrapper classes like Integer, Double, Boolean, Short, etc which can store one primitive value. Whereas elements in arrays are of primitive types. Examples are int, double, byte, short, etc. Java provides mechanisms like autoboxing(converting a primitive value to a corresponding object of wrapper class) and unboxing(converting an object of a wrapper class to a corresponding primitive type) to change the data type from primitives to objects and vice-versa. A trivial way of converting an ArrayList to an Array is by declaring an array of the given size and keeping adding the numbers sequentially. But, is this the only way?
ArrayList To Array Conversion In Java
Let's walk through 3 different ways of conversion
1. Manual conversion using the get() method
As discussed earlier, we'll declare an array of the given size, then iterate over each number and add it to the array. And here's the code to do that in java
Let's have a walkthrough of the code. Initially, we're importing the List interface and ArrayList class from the java util library then declared an ArrayList of integers . We've added a few elements to the ArrayList.
To convert it to an array, we've created an array of the given size. We've created one primitive array, and an object array to demonstrate the unboxing concept. While appending elements to ObjectArr[], elements are stored as Integer class objects which is a straight operation since the get() method returns an object. Whereas appending in PrimitiveArr[] is not a direct operation, java performs unboxing which converts the object to a primitive type, and then it is stored in the array.
Once we add all the elements to the array, we're printing the elements of the newly created arrays.
Output
The time complexity of this code will be since we are iterating through all the elements and adding them to the array so . Fetching the elements, and unboxing are considered to be done in constant time .
The space complexity of this code will be since we are declaring a new array of the given size n.
Here we've converted an ArrayList to Array in Java but the code seems to be a little long right?
2. Object[] toArray() method in java
This way of converting an ArrayList to Array in Java uses the toArray() method from the java List interface and returns an array of type Object. It will convert the list to an array without disturbing the sequence of the elements.
And here's the code to do that in java
Let's have a walkthrough of the code. We've declared an array of type Object and assigned the value returned by the toArray() method. This will dynamically create an array of the required size and append the elements to it.
We can also pass an object array as the parameter, this method signature will be useful if we already have an array of size which is larger enough to store the elements such that the elements of the list are stored in the array which is passed as parameter. The size of the array need not be exact but should be large enough, else the array remains unchanged.
Then we’re printing the elements of the newly created arrays.
Output
The time complexity of this code will be , thinking why ? Although there could be other ways of doing this with lesser time complexity(like multi-threading). The default implementation of the toArray() method is the same as our manual conversion, we can change the implementation if required.
The space complexity of this code will not be affected if elements are appended to the parameter array, else since a new array will be created.
Remember if the elements are to be stored in a primitive int[] array or object Integer[] array, then each element has to be typecasted and then stored.
Directly assigning the Object[] array to an Integer[] array will throw an error in java. And here's the java code to demonstrate that
3. T[] toArray(T[] arr) method in java
This way of converting an ArrayList to Array in Java uses the toArray() method and returns an array whose type is the same as the parameter passed to the array. The parameter array will not only decide the type of returned array but also stores the elements in it if the array is large enough. If the parameter array is smaller than required then a new array will be returned.
And here's the code to do that in java
Let's have a walkthrough of the code. We've declared an Integer array and an Object array of the required size. Then passed it to the toArray() method, since the size of the array is large enough the elements are added to the same array. The code will work fine with both the Integer array and Object array because Integer and Object fall under the supertype of the elements in ArrayList.
Then we’re printing the elements of the newly created arrays.
Output
The time complexity of this code will be since the default implementation of the toArray() method is the same as our manual conversion.
The space complexity of this code will be if the parameter array is newly created, else the space complexity will not be affected since elements are added to an existing array.
Remember the type of parameter array has to be the supertype of the elements in the ArrayList i.e. if the elements in ArrayList are of Integer type, then the converted array can be of type Integer or Object. Else the program will throw an error.
Here's the java code to demonstrate that
Conclusion
-
Arrays are of fixed size and can store elements of primitive types.
-
ArrayLists are resizable arrays and can store elements of type wrapper class objects.
-
Java provides the flexibility of converting ArrayLists to Array and vice versa.
-
There are three ways of conversion - manual conversion using get() method, using Object[] toArray() method, using T[] toArray(T[] arr) method.
-
In conclusion, on which way to opt for converting an ArrayList to Array in Java, we can go for
- manual conversion if we do not want to explicitly typecast the elements.
- Object[] toArray() if we want to create a new Object[] array or add elements to an existing Object[] array.
- T[] toArray(T[] arr) if we want to add elements to the parameter array or make the resultant array type the same as our parameter array.