JavaScript Arrow Function
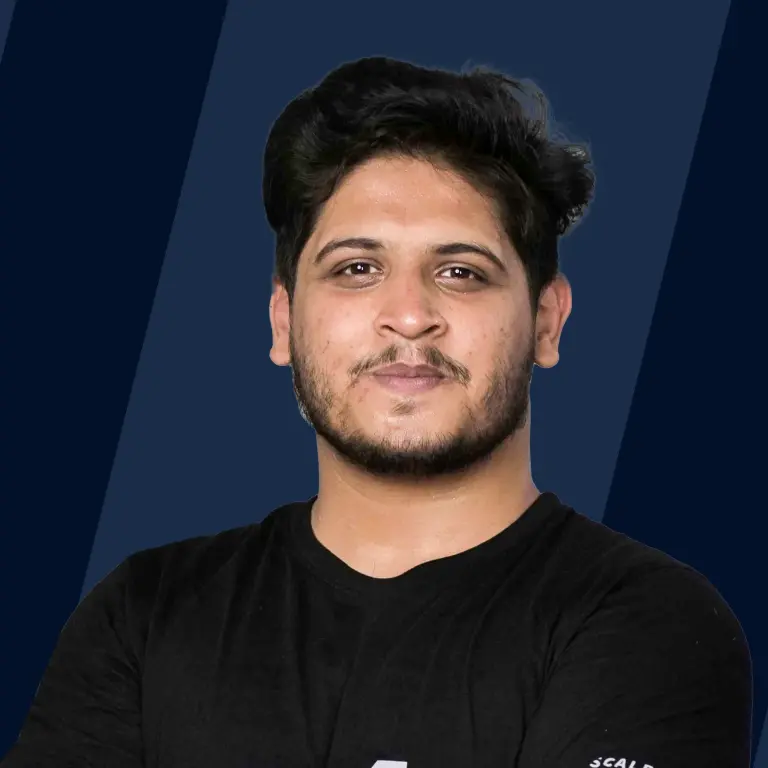
Arrow function in JavaScript was introduced in ES6 it offers a concise syntax compared to traditional functions. They support both single and multiple parameters, with or without default values, and can be written with implicit returns for single expressions.
What is Arrow Function in JavaScript?
Arrow function in JavaScript is an alternative to regular function in Javascript. ES6 introduced the feature of the arrow function, which looks cleaner compared to the regular javascript function.
Syntax
where,
- ArrowFunctionSyntax is the name of the function,
- (arg1,arg2,arg3....argN) are the function arguments
- statement(s) is the function body.
Example
Let's see a function to multiply two numbers in javascript.
1. Using Regular Javascript function.
2. Using Arrow function
Arrow Function in JavaScript without Parameters
When an arrow function in javascript does not take any parameters, you use empty parentheses () to denote the absence of parameters.
Syntax
Example
Output:
Arrow Function with Parameters
JavaScript arrow functions can take one or more parameters.
Syntax
- Single parameter: param => { statements }
- Multiple parameters: (param1, param2, ...) => { statements }
Examples
Single Parameter
Multiple Parameters
Arrow Function in JavaScript with Default Parameters
Arrow functions in JavaScript can be defined with default parameters, allowing functions to automatically use a predefined value when no argument or undefined is provided for that parameter.
Syntax
Example
Using async with Arrow Functions
Arrow functions can be made asynchronous by adding the async keyword, allowing them to execute asynchronous code using await.
Advantages of JavaScript Arrow Functions
- Streamlines syntax, leading to more concise code.
- Implicit return in single-expression functions eliminates the need for return keyword.
- Enhances code clarity, especially in functional programming patterns.
- Lexically captures the this context, simplifying usage in callbacks and methods.
- Facilitates clearer and simpler function chaining and composition.
- Reduces the likelihood of this-related bugs in callbacks and closures.
Limitations of JavaScript Arrow Functions
- Lack of a prototype property, making them unsuitable for prototype-based operations.
- Incompatibility with the new keyword, preventing their use as constructor functions.
- They inherently bind to the enclosing lexical context's this, limiting dynamic this context assignment.
- Identified as anonymous functions, which can complicate debugging efforts.
- Unable to act as generator functions, as they cannot leverage the yield keyword.
Traditional Functions Vs Arrow Functions
Feature | Traditional Functions | Arrow Functions |
---|---|---|
Syntax | function name() { ... } | const name = () => { ... } |
this Binding | The this value can change based on the context in which it's called. | Inherits this from the parent scope where the function is defined. |
Constructor | Can be used as constructors with the new keyword. | Cannot be used as constructors; they throw an error if used with new. |
Arguments Object | Have their own arguments object. | Do not have their own arguments object but can access it from the parent scope. |
Return | Requires an explicit return statement for non-void functions. | Implicitly returns the expression's result without a return statement if the function body is a single expression. |
this with Arrow Function
- Arrow functions do not have their own this context; instead, they capture the this value of the enclosing scope at the time they're created.
- This makes them unsuitable for methods in objects where this should refer to the object itself.
- They're ideal for callbacks where you want to preserve the outer this value, such as in event handlers or asynchronous code.
- Arrow functions cannot be used as constructors; attempting to use new with an arrow function will throw an error.
- Due to lexical this binding, arrow functions are a common choice for writing concise functional expressions.
Supported Browsers
Every browser except Internet Explorer supports the arrow function.
Conclusion
- Arrow function in JavaScript is a cleaner alternative to the regular function in Javascript.
- What is arrow function in JavaScript, It is a function that does not have its own arguments, prototype, and new.target properties.
- Every browser except Internet Explorer supports the arrow function.
- Objects can be directly returned using the arrow function by wrapping the object in parenthesis.