Boolean in JavaScript
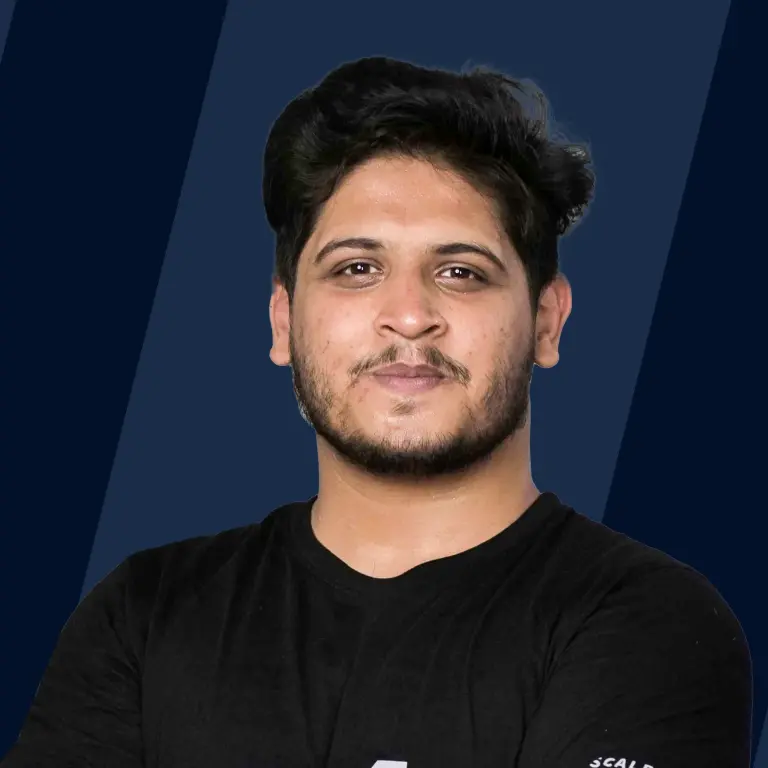
In real life, a statement can be valid or invalid that is either true or false, in the same way, Boolean object in JavaScript is a logical data type with any one of the two values possible at a time i.e either true or false.
Boolean in JavaScript is a primitive wrapper object for the boolean data type and is used for the representation and manipulation of boolean values that are used in programming.
Description
Whenever there is a need to convert any value to a Boolean in JavaScript, it can be passed as a parameter for the conversion to a boolean data type. It can result in any of the two values that are either true or false. If the passed value is 0, null, false, NaN, undefined, empty, or an empty string, the boolean object has the value of false. In contrast(opposite) to this, all the remaining values, be it an empty array, string as "false", or any object, the boolean value has the value of true.
Boolean in JavaScript can be used as a function that helps convert other data type values like numeric strings to the boolean data type values. In the cases in which other values can not be converted, the Boolean function returns an Uncaught SyntaxError.
Boolean Values
Sometimes in programming, you will need a data type that can only have one of two values, like: -> ON / OFF -> True / False -> YES / NO
For this, Boolean in JavaScript has two values, i.e. true or false.
The Boolean() Function
JavaScript's Boolean() function converts a value to its corresponding Boolean representation. JavaScript provides the Boolean() function to convert any value into a boolean primitive: true or false.
Boolean primitives and Boolean objects
- In JavaScript, there are two boolean primitives:
- True
- False
- Boolean objects are instances of the Boolean constructor.
- The Boolean() Constructor is used to create a new Boolean Object in JavaScript using new keyword.
Syntax
Example:
In most cases, it's preferable to use boolean primitives (true or false) instead of Boolean objects for better performance and consistency.
Boolean coercion
- Boolean coercion refers to the automatic conversion of non-boolean values to boolean values in JavaScript.
- This process occurs implicitly in contexts where a boolean value is expected, such as in conditional statements (if, while, etc.) or logical operations (&&, ||, !).
JavaScript follows certain rules for boolean coercion:
- Falsy Values: Values that are considered "falsy" (such as false, 0, '', null, undefined, and NaN) are coerced to false.
- Truthy Values: All other values, known as "truthy" values, are coerced to true.
Example:
In this example, the number 0 is considered falsy, so it's coerced to false. Therefore, the output will be "Number is falsy".
Constructor
- The Boolean constructor in JavaScript creates Boolean objects in Javascript.
- It can be invoked with or without the new keyword.
Instance Properties
- Boolean objects have properties inherited from their prototype, including prototype, constructor, and others.
- These properties provide metadata and methods associated with Boolean objects.
Instance Methods
- Boolean objects in Javascript have methods defined in their prototype
- It enables manipulation and retrieval of boolean values.
Boolean.prototype.toString()
- The toString() method returns a string representation of the Boolean object. It converts the boolean value (true or false) to a string ("true" or "false").
Boolean.prototype.valueOf()
- The valueOf() method returns the primitive boolean value of the Boolean object.
Comparisons and Conditions
Operator | Description | Example | Result |
---|---|---|---|
== | Equal to (loose equality) | 5 == 5 | true |
=== | Equal to (strict equality) | 5 === '5' | false |
!= | Not equal to (loose inequality) | 5 != 10 | true |
> | Greater than | 10 > 5 | true |
< | Less than | 5 < 10 | true |
These examples explain how the comparison operator works and the resulting value of boolean in javascript. You can check out this article to learn more about comparison operators.
As Comparison operators return a boolean value, they are also widely used with conditional javascript statements like if-else statements .
JavaScript Booleans as Objects
- In JavaScript, booleans can be represented as objects using the Boolean constructor.
- These Boolean objects have additional properties and methods compared to boolean primitives.
Examples
a) Creating Boolean objects with an initial value of false
Output:
b) Creating Boolean objects with an initial value of true
Output:
Browser Compatibility
Boolean objects are supported in all major browsers, including Chrome, Firefox, Safari, Edge, and Internet Explorer. However, they may behave differently in some older versions of Internet Explorer.
Conclusion
- Boolean in JavaScript serves as a logical data type with values of either true or false.
- The Boolean() function converts values to their corresponding boolean representation.
- JavaScript has boolean primitives (true and false).
- Boolean coercion automatically converts non-boolean values to boolean values.