C Pass Addresses and Pointers
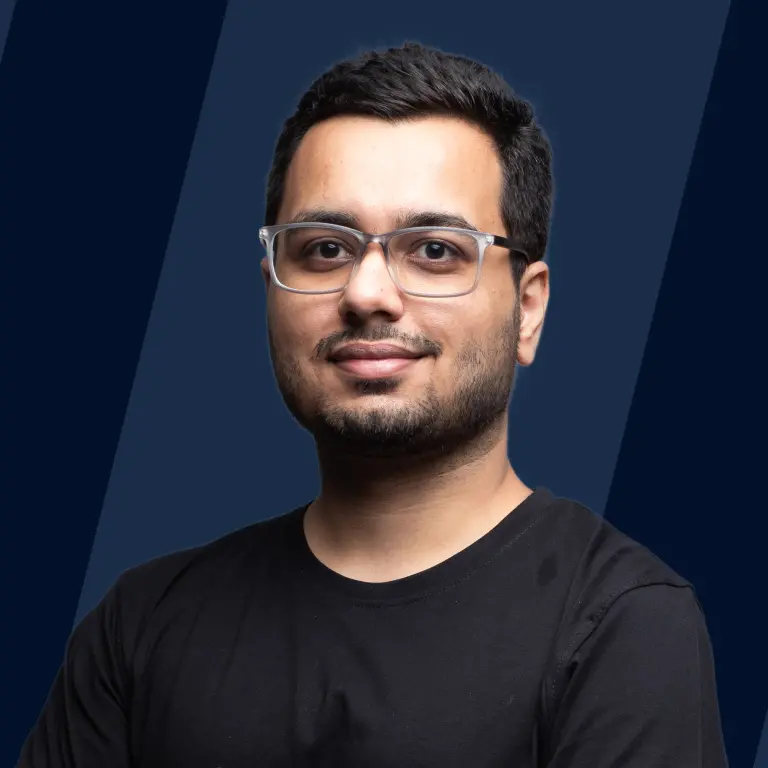
Overview
Passing addresses and pointers is a fundamental technique in C programming that plays a critical role in effectively managing data. When we send an address or a pointer to a function, we effectively provide it with direct access to the original data stored in memory rather than making a copy. This saves memory and enables us to directly edit the original data, making our programs more efficient.
Passing Addresses to Functions in C
The capacity to efficiently transmit data between functions is a key idea in programming. Passing addresses to functions is a typical approach in the C programming language for accomplishing this. This method allows functions to manipulate the original data directly rather than dealing with copies, which can save memory and time.
Before giving addresses to functions, we must first understand the idea of memory addresses. Each variable in memory in C has a unique address - a position in the computer's memory where its data is stored. These addresses are written in hexadecimal format, making them easy to identify.
Passing by Value vs. Passing by Reference
In C, you may provide a variable to a function in one of two ways: by a value or a reference. A copy of the variable's value is sent to the function when passing by value. Any modifications within the function do not affect the original variable outside of it. On the other hand, giving via reference entails giving the variable's memory location to the function. This enables the function to operate with the original data directly.
To pass an address to a function, you need to use pointers. A pointer is a variable that stores the memory address of another variable. Here's a simple example:
Explanation
In this example, modifyValue takes an integer pointer as its argument, allowing it to change the value stored at the address pointed to by ptr.
Benefits and Use Cases
Passing addresses to functions is very handy when dealing with big data structures or when you want to avoid making memory-intensive data copies. It is typically employed in settings where data alteration happens frequently, such as sorting algorithms.
Passing Pointers to Functions in C
Pointers are like secret agents in C programming; they allow you to manipulate data more efficiently and powerfully. But what if we told you that you could also send function pointers? Yes, you read that correctly! In this post, we'll look at the technical aspects of sending pointers to functions in C.
Before we get started, let's quickly review what pointers are. A pointer is a variable in C that contains the memory address of another variable. Consider it a guidepost directing you to the location of your data. As a result, pointers are extremely beneficial for tasks such as dynamic memory allocation and efficient data processing.
Passing Function Pointers: Now, let's get to the interesting part – passing pointers to functions. Why would you want to do this? It allows you to modify variables outside the function's scope, making your code more versatile.
Example
Explanation
In this example, we define a function modifyValue that takes a pointer to an integer as an argument. Inside the function, we modify the value pointed to by ptr, effectively changing the value of num in the main function.
Benefits of Passing Pointers to Functions:
- Efficient Memory Usage: Passing pointers instead of the entire data to functions conserves memory and execution time, especially when dealing with large datasets.
- Data Modification: Functions can alter the original data using pointers, making managing and updating information easier.
- Shared Data: Multiple functions can access and modify the same data through pointers, promoting code reusability.
- Improved Performance: Passing pointers can be faster than passing data directly, as it reduces the need for copying large amounts of data.
While sending pointers to functions has many advantages, it also has some drawbacks. To avoid difficulties such as segmentation faults, you must ensure that the pointers you send are valid and that you handle memory appropriately.
Important Concepts
Pointer-to-Function:
Passing function pointers involves sending the memory address of a function as an argument to another function. This technique is especially useful in scenarios like callback mechanisms, where a function needs to call different functions based on certain conditions. It allows for dynamic behavior, making code more modular and flexible.
Pointer Casting:
Pointer casting refers to converting a pointer from one data type to another. It is useful when working with polymorphic data or interchanging data between different data types. However, it should be done cautiously to prevent memory corruption or undefined behavior. Safe casting depends on the compatibility of the source and target data types.
Pointer Arithmetic:
Pointer arithmetic involves manipulating pointers to navigate and manipulate data efficiently. Incrementing a pointer moves it to the next memory location of its data type, while decrementing moves it backward. This is especially useful in array traversal and dynamic memory management. Care must be taken not to go beyond the bounds of allocated memory to prevent memory errors like buffer overflows.
Conclusion
- Utilizing pointers to pass addresses in C enables efficient memory management. It allows functions to access and manipulate data in memory directly, reducing the overhead of copying large data structures.
- Pointers provide fine-grained control over data, allowing functions to modify variables at their original memory locations. This precision is crucial for implementing data structures and algorithms effectively.
- By passing addresses rather than values, functions can alter variables outside their immediate scope. This is similar to passing variables by reference, allowing two-way communication between functions.
- Pointers are fundamental for dynamic memory allocation with functions like malloc and free. This dynamic memory management is essential for handling data structures like linked lists and trees.
- Passing addresses uses less memory than passing huge data structures. It improves resource utilization and is especially useful in embedded devices and low-level programming.