Arithmetic Operators in C
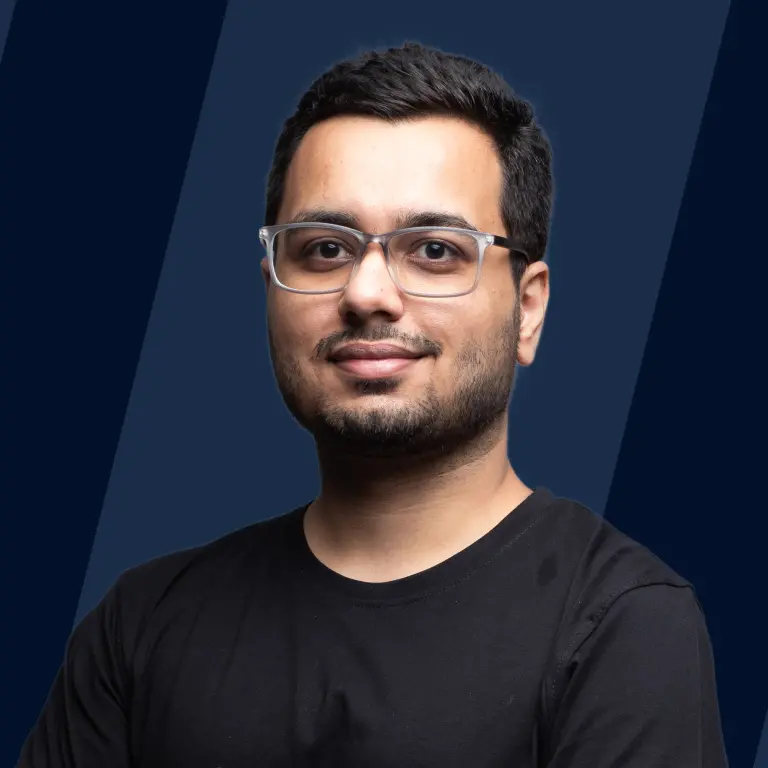
Overview
Arithmetic expressions are a combination of operands interjoined with arithmetic operators. They ultimately evaluate to a single value. We, humans, use a technique called BODMAS to evaluate arithmetic expressions in our real life, but the computers and the C programming language make use of a similar but different technique. They use precedence and associativity.
What are C Arithmetic Operators?
C arithmetic operators are the symbols that are used to perform mathematical operations on operands. Arithmetic operators can also be used to perform operations on any numeric data type, such as int, float, and double.
Types of Arithmetic Operators in C
The C Arithmetic Operators are of two types based on the number of operands they work. These are as follows:
- Binary Arithmetic Operators
- Unary Arithmetic Operators
Binary Arithmetic Operators
A Binary arithmetic operator is used to perform arithmetic/mathematical operations on two operands. C provides 5 Binary Arithmetic Operators for performing arithmetic functions which are as follows:
Operator | Name of Operator | What it does | How it is used |
---|---|---|---|
+ | Plus | Add two Operands | a+b |
- | Minus | Subtracts the second operand from the first. | a-b |
* | Multiplication | Multiplies both operands. | a*b |
/ | Division | Divides numerator by de-numerator. | a/b |
% | Modulus | return remainder, after an integer division. | a%b |
Example of Binary Arithmetic Operator in C
Output:
This example shows how to use the four basic arithmetic operators (+, -, *, and /) in C. The % operator is used to find the remainder of a division operation.
Unary Arithmetic Operators
The Unary arithmetic operators are mathematical operators that operate on a single operand. In C, we have four unary arithmetic operators which are as follows:
Operator | Symbol | Operation | Implementation |
---|---|---|---|
Decrement Operator | -- | Decreases the integer value of the variable by one. | - - h or h - - |
Increment Operator | ++ | Increases the integer value of the variable by one. | ++ h or h ++ |
Unary Plus Operator | + | Returns the value of its operand. | +h |
Unary Minus Operator | - | Returns the negative of the value of its operand. | -h |
Example of Unary Arithmetic Operator in C
Output:
Explanation:
The unary minus operator (-) negates the value of its operand. In this example, the operand is the variable a, which has a value of 10. Therefore, the result of the expression -a is -10.
Unary operators can be used to perform a variety of tasks, such as:
- Changing the sign of a number
- Incrementing or decrementing a variable by 1
- Getting the complement of a number
- Getting the address of a variable
Multiple Operators in a Single Expression
Output:
Explanation:
The expression in the result variable is evaluated from left to right, according to the operator precedence rules in C. The parentheses around the expression 2 * b ensure that it is evaluated first. This results in the following steps:
- 2 * b is evaluated, which gives 10.
- a + b is evaluated, which gives 15.
- c / 10.0 is evaluated, which gives 2.0.
- 15.0 * 2.0 is evaluated, which gives 30.0.
- The final result is assigned to the result variable.
Therefore, the output of the program is 25.000000.
Examples of C Arithmetic Operators
Example 1: C Program to find the area of a rectangle and triangle.
Output:
FAQ
Q. List all the arithmetic operators in C. A. Arithmetic operators in C are:
- ( + ) Addition Operator
- ( – ) Subtraction Operator
- ( * ) Multiplication Operator
- ( / ) Division Operator
- ( % ) Modulo Operator
- ( ++ ) Increment Operator
- ( — ) Decrement Operator
- ( + ) Unary Plus Operator
- ( – ) Unary Minus Operator
Q. What is the difference between the unary minus and subtraction operators?
A.
Aspect | Unary Minus | Subtraction Operator |
---|---|---|
Operands | Single operand | Two operands |
Functionality | Returns the negative of the operand's value | Returns the difference between the operands |
Conclusion
- C arithmetic operators are used to perform mathematical operations on operands.
- C has two types of arithmetic operators: binary and unary.
- Binary arithmetic operators operate on two operands, while unary arithmetic operators operate on a single operand.
- Some common binary arithmetic operators include addition (+), subtraction (-), multiplication (*), division (/), and modulo (%) operators.
- Some common unary arithmetic operators include increment (++), decrement (--), unary plus (+), and unary minus (-) operators.
- Operators can be combined to form complex expressions.
- The order in which operators are evaluated is determined by operator precedence rules.