Assignment Operators in C
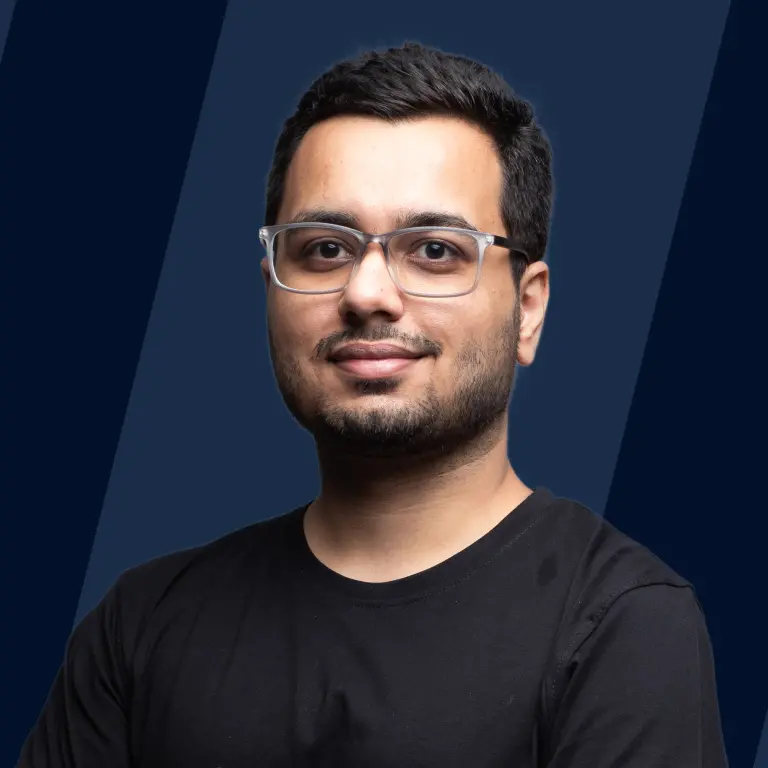
Operators are a fundamental part of all the computations that computers perform. Today we will learn about one of them known as Assignment Operators in C. Assignment Operators are used to assign values to variables. The most common assignment operator is =. Assignment Operators are Binary Operators.
Types of Assignment Operators in C
Assignment Operators help us to assign the value or result of an expression to a variable and the value on the right side must be of the same data type as the variable on the left side. They have the lowest precedence level among all the operators and it associates from right to left.
Here is a list of the assignment operators that you can find in the C language:
- basic assignment ( = )
- subtraction assignment ( -= )
- addition assignment ( += )
- division assignment ( /= )
- multiplication assignment ( *= )
- modulo assignment ( %= )
- bitwise XOR assignment ( ^= )
- bitwise OR assignment ( |= )
- bitwise AND assignment ( &= )
- bitwise right shift assignment ( >>= )
- bitwise left shift assignment ( <<= )
Working of Assignment Operators in C
This is the complete list of all assignment operators in C. To read the meaning of operator please keep in mind the above example.
Operator | Meaning Of Operator | Example | Same as |
---|---|---|---|
= | Simple assignment operator | x=y | x=y |
+= | Add left operand to right operand then assign result to left operand | x+=y | x=x+y |
-= | subtract right operand from left operand then assign result to left operand | x-=y | x=x-y |
*= | multiply left operand with right operand then assign result to left operand | x*=y | x=x*y |
/= | divide left operand with right operand then assign result to left operand | x/=y | x=x/y |
%= | take modulus left operand with right operand then assigned result in left operand | x%=y | x=x%y |
<<= | Left Shift Assignment Operator means the left operand is left shifted by right operand value and assigned value to left operand | x<<=y | x=x<<y |
>>= | Right shift Assignment Operator means the left operand is right shifted by right operand value and assigned value to left operand | x>>=y | x=x>>y |
&= | Bitwise AND Assignment Operator means does AND on every bit of left operand and right operand and assigned value to left operand | x&=y | x=x&y |
|= | Bitwise inclusive OR Assignment Operator means does OR on every bit of left operand and right operand and assigned value to left operand | x|=y | x=x|y |
^= | Bitwise exclusive OR Assignment Operator means does XOR on every bit of left operand and right operand and assigned value to left operand | x^=y | x=x^y |
Example for Assignment Operators in C
-
Basic assignment ( = ):
-
Subtraction assignment ( -= ):
-
Addition assignment ( += ):
-
Division assignment ( /= ):
-
Multiplication assignment ( *= ):
-
Modulo assignment ( %= ):
-
Bitwise XOR assignment ( ^= ):
-
Bitwise OR assignment ( |= ):
-
Bitwise AND assignment ( &= ):
-
Bitwise right shift assignment ( >>= ):
-
Bitwise left shift assignment ( <<= ):
This is the detailed explanation of all the assignment operators in C that we have. Hopefully, This is clear to you.
Practice Problems on Assignment Operators in C
1. What will be the value of a after the following code is executed?
A) 10 B) 11 C) 12 D) 15
Answer – C. 12 Explanation: a starts at 10, increases by 5 to 15, then decreases by 3 to 12. So, a is 12.
2. After executing the following code, what is the value of num?
A) 4 B) 8 C) 16 D) 32
Answer: C) 16 Explanation: After right-shifting 8 (binary 1000) by one and then left-shifting the result by two, the value becomes 16 (binary 10000).
FAQs
Q. How does the /= operator function? Is it a combination of two other operators?
A. The /= operator is a compound assignment operator in C++. It divides the left operand by the right operand and assigns the result to the left operand. It is equivalent to using the / operator and then the = operator separately.
Q. What is the most basic operator among all the assignment operators available in the C language?
A. The most basic assignment operator in the C language is the simple = operator, which is used for assigning a value to a variable.
Conclusion
- Assignment operators are used to assign the result of an expression to a variable.
- There are two types of assignment operators in C. Simple assignment operator and compound assignment operator.
- Compound Assignment operators are easy to use and the left operand of expression needs not to write again and again.
- They work the same way in C++ as in C.