Command Line Arguments in C
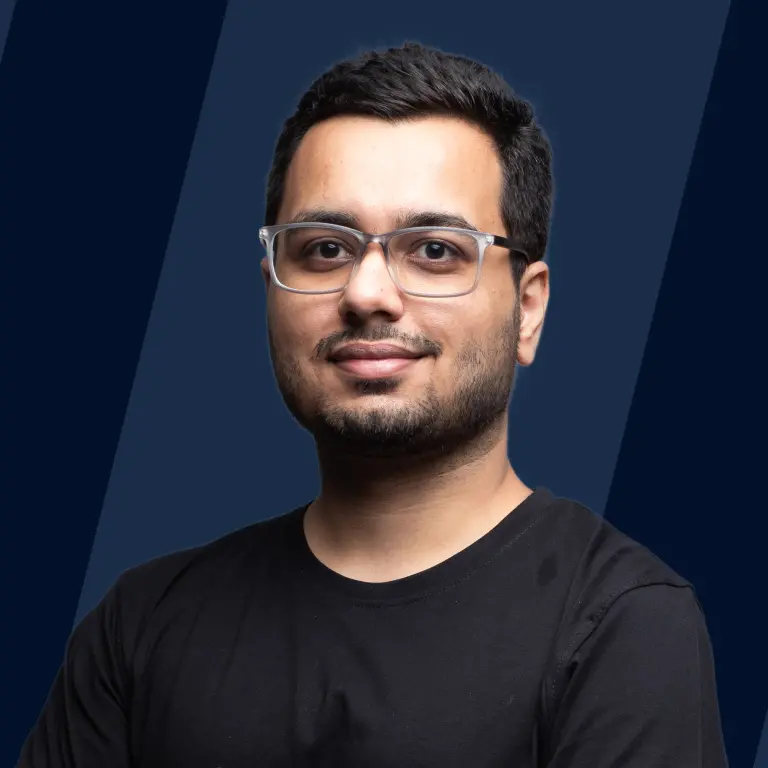
Overview
Command line argument is an important concept in C programming. It is mostly used when you need to control your program from outside. In C, command line arguments are passed to the main() method.
Introduction to Command Line Arguments in C
The command line is a text interface for your computer that allows you to enter commands for immediate execution. A command-line can perform almost everything that a graphical user interface can. Many tasks can be performed more rapidly and are easier to automate.
Let's say we have a weather forecasting application developed in any language. When we run the program, it will display a graphical user interface (GUI) where you can enter the city name and hit the ENTER button to know about the current weather. But if you don't have that GUI, which means you can't click on any buttons, that is where command-line arguments come into play, where we pass the parameters in the terminal box to do any actions.
For example:
weather "Delhi" and hit the ENTER key, this will show you the current weather.
Before we go any further, let us define certain terms that will be used in this article, such as Command-Line and Command-Line Arguments.
For example:
- You can navigate around your computer's files and directories using the command line.
- The command line can be scripted to automate complex tasks, like the example given below:
If a user wants to put 50+ files' data into a file, this is a highly time-consuming task. Copying data from 50+ files, on the other hand, can be done in less than a minute with a single command at the command line. And many more..
Syntax:
What are Command-Line Arguments?
Command-line arguments are simple parameters that are given on the system's command line, and the values of these arguments are passed on to your program during program execution. When a program starts execution without user interaction, command-line arguments are used to pass values or files to it.
What are Command-Line Arguments in C?
- When the main function of a program contains arguments, then these arguments are known as Command Line Arguments.
- The main function can be created with two methods: first with no parameters (void) and second with two parameters. The parameters are argc and argv, where argc is an integer and the argv is a list of command line arguments.
- argc denotes the number of arguments given, while argv[] is a pointer array pointing to each parameter passed to the program. If no argument is given, the value of argc will be 1.
- The value of argc should be non-negative.
Syntax:
Properties of Command Line Arguments in C
- Command line arguments in C are passed to the main function as argc and argv.
- Command line arguments are used to control the program from the outside.
- argv[argc] is a Null pointer.
- The name of the program is stored in argv[0], the first command-line parameter in argv[1], and the last argument in argv[n].
- Command-line arguments are useful when you want to control your program from outside rather than hard coding the values inside the code.
- To allow the usage of standard input and output so that we can utilize the shell to chain commands.
- To override defaults and have more direct control over the application. This is helpful in testing since it allows test scripts to run the application.
Output in Different Scenarios
Now, we will pass various types of arguments in our code, and see the output our code generates.
- Without Argument: When we run the above code and don’t pass any argument, let’s see the output our code generates.
- Pass single argument: When we pass the single argument separated by space but inside the double quotes or single quotes. Let’s see the output
- Pass more than single argument: When we run the program by passing more than single argument, say three arguments. Let’s see the output:
Usage of Command-Line Arguments in C
- It enables standard input and output to be utilized, enabling commands to be linked using a shell.
- It serves to make the installation and usage of basic programs easier.
Example of Command Line Argument in C
Now, we will pass various types of arguments in our code, and see the output our code generates.
- Without Argument: When we run the above code and don’t pass any argument, let’s see the output our code generates.
- Pass single argument: When we pass the single argument separated by space but inside the double quotes or single quotes. Let’s see the output.
- Pass more than single argument: When we run the program by passing more than single argument, say three arguments. Let’s see the output:
You can run your code here in this Online C Compiler.
Advantages of Command-Line Arguments in C
- A command-line argument allows us to provide an unlimited number of arguments.
- The data is passed as strings as arguments, so we can easily convert it to numeric or other formats.
- It is useful for configuration information while launching our application.
Conclusion
So far, we've gone through the command line in depth, now conclude the topic:
- Every programmer should use command-line arguments because they increase interaction with the operating system and help you understand how things operate.
- Through Command Line Interface (CLI) , a user can interact with the operating system or application by typing the commands. After that, the system responds to the command, and the user can type the next command for performing the next operation.
- Every programming language contains command-line tools that improve the attractiveness of the language and allow users to interact with their system more simply.