Conditional Operator in C
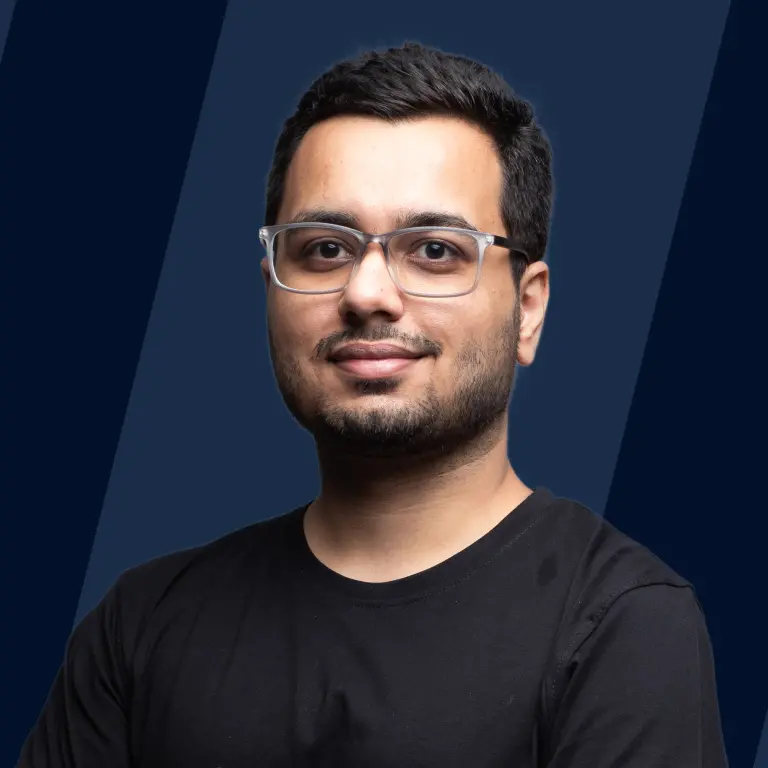
The conditional operator in C, often referred to as the ternary operator, offers a streamlined way to execute if-else statements in just one line. This powerful tool allows programmers to evaluate a condition and choose between two paths: one if the condition is true, and another if it's false. Its simplicity and efficiency make it a favorite for optimizing code readability and functionality.
Syntax of Conditional Operator in C
One common way to employ the conditional operator is as follows:
This structure is straightforward, where variable is assigned valueIfTrue if the condition evaluates to true, and valueIfFalse otherwise.
Alternatively, the syntax can be elaborated to explicitly encompass the condition within parentheses:
Additionally, there exists a variation that emphasizes the assignment within the branches of the conditional operator:
Each of these forms of the conditional operator syntax in C allows programmers to write more concise and readable code.
Working of Conditional Operator in C
Working of all versions of Conditional Operator are similar and here's how it operates:
- The initial step involves evaluating a specific condition, denoted as Condition.
- Depending on the outcome of this evaluation, the flow diverges:
- If Condition evaluates to true, the operation proceeds with Expression2. This part of the code is executed, leading to the execution of actions or the return of values specific to the true condition.
- Conversely, if Condition is found to be false, Expression3 is the path taken. In this scenario, the code block or value corresponding to the false condition is executed or returned.
- The final step culminates in the return of the result from the executed expression, effectively completing the conditional operation.
Flow Chart of Conditional Operators in C
The below diagram will help you remember the working of the Conditional Operator in C much more efficiently.
The below diagram will help you in remembering the working of another verison of the Conditional Operator in C much more efficiently
Examples of Conditional Operators in C
Here are examples demonstrating the use of the conditional operator in C to achieve different functionalities.
Example 1: Program to Store the Greatest of Two Numbers
Output:
Explanation: This program prompts the user to input two numbers. It then uses the conditional operator to compare these numbers and assigns the greater number to the variable greatest, which is then printed.
Example 2: Program to Check Whether a Year is a Leap Year
Output:
Explanation: This code checks if the entered year is a leap year. It uses the conditional operator to evaluate whether the year is divisible by 4 and not by 100, or it is divisible by 400. Based on this condition, it prints whether the year is a leap year or not.
Example 3: Program to find given Number is Odd or Even
Output:
Explanation: In this example, the user is prompted to enter a number. The program then uses the conditional operator to determine whether the number is even or odd by checking the remainder of the division of the number by 2. Depending on the result, it prints out the appropriate message.
Associativity of the Conditional Operator in C
The Associativity property defines the order in which the operands of the operator gets executed. When an expression has more than one operator in it, and more than one operators have the same precedence, then the order in which the operator gets executed is based on the associativity.
The Associativity of the conditional operator is from Right to Left.
Difference Between Conditional Operator in C and if-else statement in C
The conditional operator can be used in the case of an if-else statement if the if-else statement only has one statement to execute. The conditional operator reduces the number of lines of code in a program.
Some noteworthy differences between the conditional operator and the if-else statement of the C programming language are listed in the below tabular column:
Conditional operator in C | if-else statement in C |
---|---|
The conditional operator is a single programming statement and can only perform one operation. | The if-else statement is a block statement, you can group multiple statements using a parenthesis. |
The conditional operator can return a value and so can be used for performing assignment operations. | The if-else statement does not return any value and cannot be used for assignment purposes. |
The nested ternary operator is complex and hard to debug. | The nested if-else statement is easy to read and maintain. |
FAQs
Q. Is the conditional operator in C and the ternary operator of the C programming language the same?
A. yes, the conditional operator are also known as the ternary operator.
Q. Why is it called a ternary operator?
A. The conditional operator is called a ternary operator because it is the only operator in C that takes three operands. The term "ternary" comes from Latin, meaning "composed of three items".
Q. Why is it called a conditional operator in C?
A. The first operand for the conditional operator is always a condition, so it is called the conditional operator.
Q. Can I use conditional operator in C instead of if statement?
A. It cannot entirely replace if statements, especially in scenarios involving more complex logic or the need to execute multiple statements conditionally without explicitly returning or assigning a value.
Conclusion
- The conditional operator, or ternary operator, in C is a succinct alternative to if-else statements, enabling conditional logic in a single line for enhanced code readability and efficiency.
- Its syntax varies from a simple variable = condition ? valueIfTrue : valueIfFalse; to more explicit forms incorporating parentheses for clarity or emphasizing assignment within its branches.
- The operator works by evaluating a condition, then executing one of two expressions based on the condition's truthiness, culminating in the return of the resultant value.
- Examples in the article illustrate its use in practical scenarios, such as determining the greater of two numbers, checking for a leap year, and assessing if a number is odd or even.