Constant Pointer in C
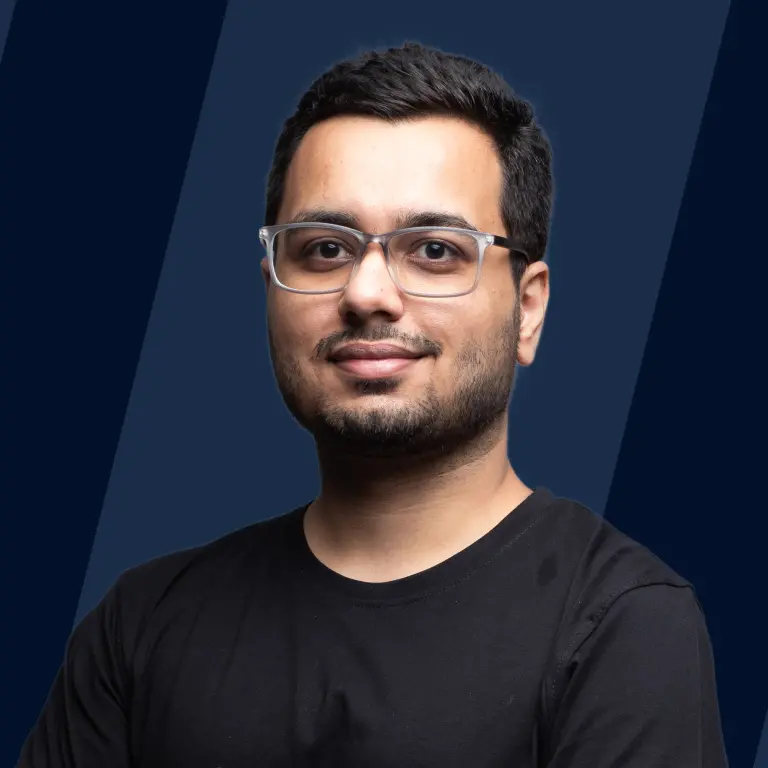
Introduction
Whenever there is a requirement to make a variable immutable in C, we can resort to the const keyword in C. By doing so, the variable cannot be modified as long as it exists in memory. Similarly, we can use the const keyword with pointers as well. There are multiple usages of pointers with the const keyword, such as
- We can create a constant pointer in C, which means that the value of the pointer variable wouldn't change.
- We can create a pointer to a constant in C, which means that the pointer would point to a constant variable (created using const).
- We can also create a constant pointer to a constant in C, which means that neither the value of the pointer nor the value of the variable pointed to by the pointer would change.
How const Pointer Works in C?
As described earlier, a constant pointer in C is one whose value cannot be changed in the program. It is quite similar to a constant variable in C. The only difference here is that, by definition, pointers store memory addresses. So, a constant pointer will keep pointing to the same memory location to which it is initially assigned.
Note: It is necessary to initialize the constant pointer during the declaration itself, unlike a normal pointer which can be left uninitialized.
Syntax
The syntax for declaring a const pointer in C is
Note: Here, the const keyword must appear after the * in the declaration.
Examples
Let's look at a few examples of correct and incorrect usages of a constant pointer in C:
-
The following code demonstrates the proper way of using a constant pointer in C.
Output
-
The following code produces an error because the constant pointer was not initialized at the time of declaration.
Output
-
As discussed previously, the value of the constant pointer variable cannot be changed as shown in the code below.
Output
-
Similar to a non-const pointer, we can use a constant pointer to alter the value stored at the memory location to which it is pointing. We can also verify that the address stored in the constant pointer remains the same after the change.
Output
Pointer to a Constant in C
Unlike the constant pointer discussed previously, a pointer to a constant in C refers to an ordinary pointer variable that can only store the address of a constant variable, i.e., a variable defined using the const keyword.
Note: Unlike a constant pointer, it is not necessary to initialize the value of a pointer to a constant at the time of declaration.
Syntax
The syntax for declaring a pointer to a constant in C is
Note: Although there are two syntaxes, as shown above, notice that the const keyword should appear before the *. This is the difference in the syntax of a constant pointer and a pointer to a constant in C.
Why Do We Need a Special Pointer Type for const Variables?
Let's understand why it is not advisable to use an ordinary pointer to store the address of a const variable. Consider the following example:
Output
As we can see from the output above, although the compiler generates a warning, the value of a has changed from 10 to 50, although a is declared as a const variable. This happens because when an ordinary pointer like ptr points to a const variable, the compiler discards the constant attribute given to the variable temporarily whenever the pointer tries to modify the value of that variable. This is not recommended as it can lead to security flaws and defeats the purpose of making the variable constant.
Note: Even though the value of a can be changed by ptr in the above example, we cannot directly alter the value of a. The following code will produce an error.
Output
Thus, we need to use a different pointer syntax to point to constant variables in C.
Examples
Let's look at a few examples of pointers to a constant in C:
- Here is an example of correct usage of a pointer to a constant in C.
Output
- The following code throws an error because we are attempting to change the value of a constant variable.
Output
- We can also use such pointers to store the address of a non-const variable.
Output
- We can change the value stored in the pointer and make it point to another constant variable.
Output
As we can see in the output above, the address stored in ptr changes and now it points to variable b.
Constant Pointer to a Constant in C
This type of pointer is used when we want a pointer to a constant variable, as well as keep the address stored in the pointer as constant (unlike the example above). In other words, a constant pointer to a constant in C will always point to a specific constant variable and cannot be reassigned to another address. This type of pointer is essentially a combination of the two types discussed previously, i.e., a combination of constant pointer and a pointer to a constant.
Note: It is necessary to initialize these types of pointers during the declaration itself.
Syntax
The syntax for declaring a pointer to a constant in C is
Here, we have two const keywords in the syntax, one before and one after the *.
Examples
Let's look at a few usages of a constant pointer to a constant in C.
-
The following code shows the correct usage of a constant pointer to a constant.
Output
-
These type of pointers can also point to non-const variables.
Output
-
The following code throws an error when we try to change the value of the pointer or the value of the constant variable using the pointer.
Output
Conclusion
There are multiple benefits of using pointers with const in C.
- When a constant pointer is declared in C, the compiler can make some optimizations when converting the C source code to assembly-level instructions.
- If it is not intended for a pointer variable that is passed as a parameter to a function to change its value, then declaring the pointer as constant acts as a check against accidental modifications.
- As described in this article, it is not advisable to use an ordinary pointer with a const variable in C due to loss of const property. So, we must use the "pointer to a constant" syntax.