Declaration of Variables in C
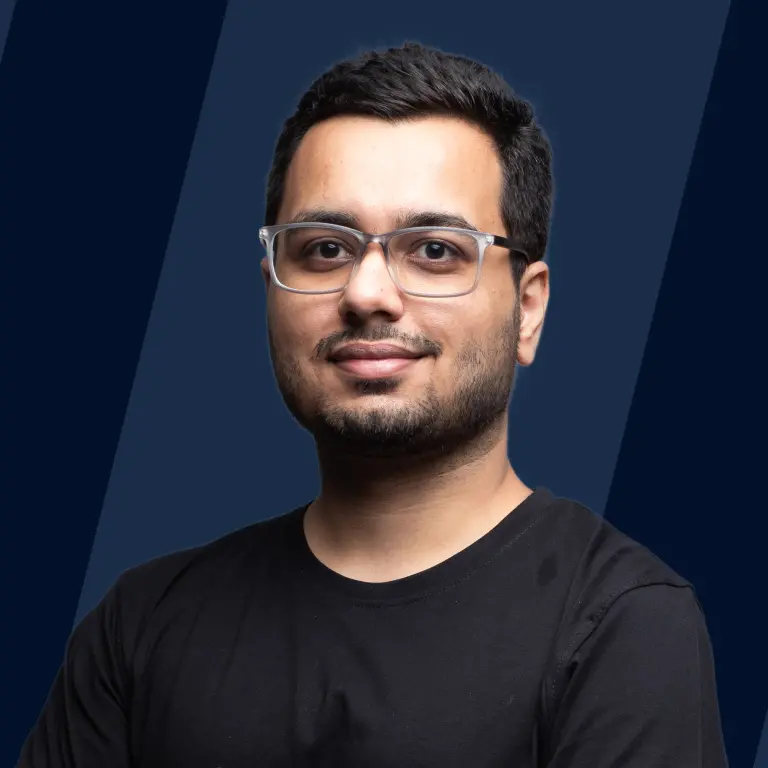
Overview
In any programming language, We can refer to anything with the help of variables. They are the most essential part, from writing a normal program to writing advanced software. Variable allows us to access the particular element and assign them with some value. With great powers comes great responsibilities, So variables are bounded by some declaration and assignment rules which we will look into.
Introduction to Variable Declaration in C
Variables are the most essential part of any programming language.
Let's say we need to calculate the area of a rectangle. To make this arithmetic calculation, we need to store the length and width of the rectangle. To store the length and width of the rectangle, we need to allocate some space in a memory location for the data, and the name given to that memory location is called Variable.
For each different data, we give different variable names to it for later use in the program.
For better understanding, let's look at the following image. It shows the memory location where the data is stored with a variable name as myvar and value 22 to it.
a) General syntax for declaring a variable
In variable declarations, we can declare variables in two ways:
- Declaration of variable without initializing any value to it
data_type variable_name;
Eg:- char Final_Grade; // Final_Grade is a variable of type char, and no value is assigned to it.
- Declaration of variable with initializing some value to it
data_type variable_name = val;
Eg:- int age = 22; // age is a variable of type int and holds the value 22.
Here, data_type specifies the type of variable like int, char, etc.
variable_name specifies the name of the variable. val is the value for which we are initializing the variable.
Program to Illustrate the Declaration of Variables in C
To use some data in the program, we need to declare a variable with the corresponding data type and assign some value to it. And then use that variable name to access the data.
While declaring a variable, memory space is not allocated to it. It happens only on initializing the variable.
- So what happens when we declare a variable without initializing it? When we declare a variable without initializing it, then it just stores some garbage value or zero value. But if we assign some value to it, then it will be overwritten with the new value.
Let's see an example to understand the above concept.
Output:
From the above program, we can see that the initial value of c is 0. And when we reassign the new value to C variable, it will be overwritten with the new value.
Types of Declaration of Variables in C
Variables in C need to be declared by storing any data type and any variable name before using it.
There are two types of declaration of variables in C:
- Primary Type Declaration
- User-Defined Type Declaration
a) Primary Type Declaration
Primary type declaration is used to declare a variable with primitive data types, which are also called as built-in data types.
Most commonly used primary data types are int, float, char, boolean, double, long etc.
- Single primary type declaration
Eg:- char Grade = 'A';
-
Multiple primary type declarations in the same line
When multiple variables are declared in the same line, we need to use a comma to separate the variables, as shown below.
Eg:- int Length= 12, Width = 13, Depth = 14;
- Multiple primary type declaration in different lines When multiple variables are declared in different lines, we need to use semi-colons to separate the variables, as shown below.
Eg:-
b) User-Defined Type Declaration
User-Defined Type Declaration is a type of declaration where the data type is defined by the user.
Some of the most commonly used data types are struct, Union, enum, typedef etc.
-
Structure Structures are used to group data items of different types into a single user-defined data type.
-
Union Unions are user-defined data types in which members share a common memory location, so any one of them is accessible at a time. When we want to access only one member, then we use Union.
-
Typedef We need to use the keyword typedef to define the data type. Now we can use those new data types in our program, as shown below.
For example,
Here, we have defined a new data type called person_name, person_age and salary. Now we can use these data types to declare variables as follows.
Here, Akhil, Bhanu, and Chaitanya are declared as char type variables and , , are declared as int type variables and , , are declared as float type variables.
-
By using user-defined data types, we can create our own data types. For example, we can create a new data type called person_info which can store the name, age and salary of a person. And also increases the readability of the program.
-
The main difference between primary type declaration and user-defined type declaration is that in the primary type declaration, we can use any variable name for any data type. But in the user-defined type declaration, we can use any identifier for any data type.
Why do We Need to Declare a Variable in C?
Basically, we need to declare a variable to store various types of data in the program. So to perform some operations or tasks with the data, we need to store them in the computer's memory location. But we can't remember the address of the memory location where the data is stored. So to access the data, we name the memory location with a variable name and size depending on the data type.
In the program, by declaring a variable, we have to tell the compiler the type of data and the variable name to access the data.
Purpose of Variable Declarations
The main purpose of variable declaration is to store the required data in the memory location in the form of variables so that we can use them in our program to perform any operation or task.
By declaring a variable, we can use that variable in our program by using the variable name and its respective data type.
Let's take an example to understand this concept.
For example, We need to calculate the total marks of a student in all the subjects. So to calculate the total marks, we need to give the individual marks of each subject to the computer so that it will add them up. To use the data, we name the memory location with variable names and assign the value to the variable. So, we can use the variable name to access the data.
Rules to Declare Variable in C
In C language, we need to declare a variable with suitable data type and variable name.
Here are some of the rules we need to follow while declaring a variable in C:
- Variables should not be declared with the same name in the same scope.
- A variable name can start with anything like the alphabet and underscore. But the variable name should not start with a number.
- A variable name must not be a reserved keyword in C. For example, if you declare a variable name as label, int, float, char, function, else etc., then it will not be able to be used as a variable name.
- A variable name can contain any combination of alphabets, numbers and underscores.
- All the declaration statements must end with a semi-colon. (;)
- It is suggested to declare the variables of same data type in the same line.
- It will be better if we declare variable names with some meaningful names and then it clearly describes the purpose of the variable.
For example,
In the above-shown example, we declared variable names with Person_name, age, and weight instead of a, b, c etc., so that we can easily understand that variable name is used to store the age of a person.
Conclusion
- We can declare the variable either along with the initialization or without initializing it. If we do not initialize the variable, then it will take in the garbage value.
- In Primary Type, we use built-in data types such as int, float, char, boolean, double, long etc. and in User Defined Type, we use user-defined data types such as struct, Union, enum, typedef etc.
- The basic variable functionality provided by the C language is intuitive and straightforward. Still, quite a few details can help you make an embedded application more reliable and efficient.