Function Pointer in C
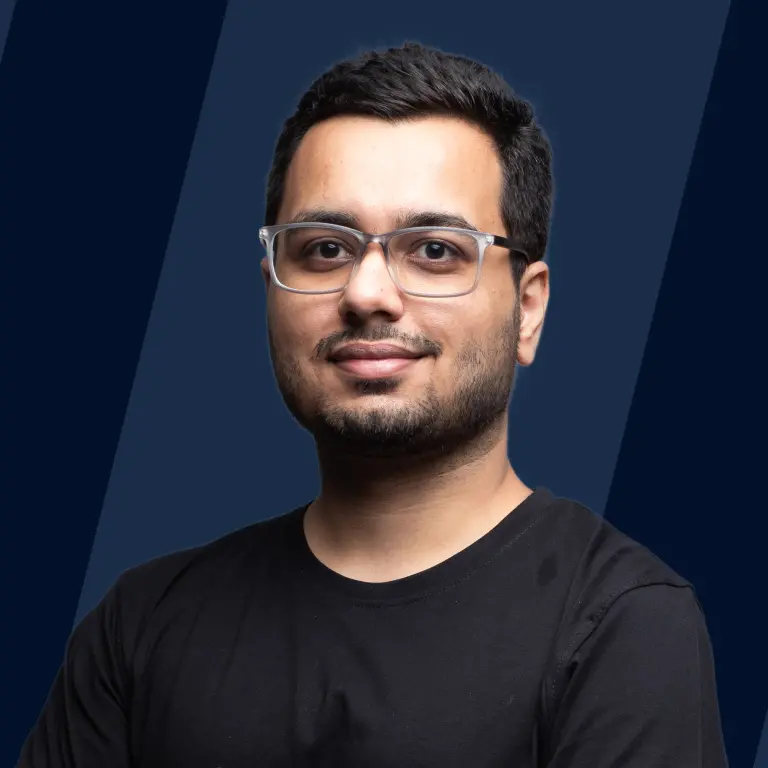
Function pointers in C hold function addresses, allowing direct calls. Declared with an asterisk and function parameters, they enable callbacks and array integrations. This article covers their declaration, use, and the concept of functions as memory-resident entities.
Declaring a Function Pointer in C
Now that we know that functions have a unique memory address, we can use function pointers in C that can point to the first executable code inside a function body.
For example, in the above figure we have a function add() to add two integer numbers. Here, the function name points to the address of the function itself so we are using a function pointer fptr that stores the address of beginning of the function add(a, b) that in 1001 in this case.
Before using a function pointer we need to declare them to tell the compiler the type of function a pointer can point to. The general syntax of a function pointer is,
Syntax of Function Pointer in C
Declaring a function pointer in C is comparable to declaring a function except that when a function pointer is declared, we prefix its name which is an Asterisk * symbol.
For example, if a function has the declaration
Declaration of a function pointer in C for the function foo will be
Here, pointer *foo_pointer is a function pointer and stores the memory address of a function foo that takes two arguments of type int and returns a value of data type float.
Tricky example
At first glance, this example seems complex but the trick to understanding such declarations is to read them inside out. Here, (*fp) is a function pointer just like normal pointer in C like int *ptr. This function pointer (*fp) can point to functions with two int * type arguments and has a return type of void * as we can see from its declaration where (int *, int *) explains the type and number of arguments ans void * is the return type from the pointed function.
Note: It is important to declare a function before assigning its address to a function pointer in C.
Calling a Function Through a Function Pointer in C
Calling a function using a pointer is similar to calling a function in the usual way using the name of the function.
Suppose we declare a function and its pointer as given below.
To call the function areaSquare, we can create a function call using any of the three ways
The effect of calling functions using pointers or using their name is the same. It is not compulsory to call the function with the indirection operator (*) as shown in the second case but it is good practice to use the indirection operator to clear out that function is called using a pointer as (*pointer)() is more readable when compared to calling function from pointers with parentheses pointer().
Example for Function Pointer in C
Now that we know the syntax of how function pointers in C are declared and used to create a function call. Let us see an example where we are creating a function pointer to call the function that returns the area of a rectangle.
Output
Here, we have defined a function areaRectangle() that takes two integer inputs and returns the area of the rectangle. To store the reference of the function, we are using function pointer (*fp) that has a similar declaration to the function it points to. To point the function address to the pointer we don't need to use & symbol as the function name areaRectangle also represents the function's address. To call the function, we pass parameters inside the parenthesis ((*fp)(length, breadth)), and the return value is stored in the variable area.
Example: Array of function pointers
Arrays are data structure that stores collection of identical data types. Like any other data types we can create an array to store function pointers in C. Function pointers can be accessed from their indexes like we access normal array values arr[i]. This way we are creating an array of function pointers, where each array element stores a function pointer pointing to different functions.
This approach is useful when we do not know in advance which function is called, as shown in the example.
Output
Here, we have stored the addresses of four functions in an array of function pointers. We used this array to call the required function using the function pointer stored in this array.
Functions Using Pointer Variables
C allows pointers to be passed in as function arguments and also returns pointers from the function. To pass pointers in the function, we simply declare the function parameter as pointer type. When functions have their pointer type arguments, the changes made on them inside the function persist even after program exists function scope because the changes are made on the actual address pointed by the pointer. This approach to pass arguments to a function is called as pass by reference because as shown in the figure below reference of the variable is passed to the function instead of the value stored in the address.
Extra care should be taken when function is used to return pointers because local variables don't live outside the function scope and if they are returned as pointers from function then that pointer will point to nothing when function terminates.
For example,
In this case, the compiler throws segmentation fault error because you are returning a copy of a pointer to a local variable. However, that local variable is de-allocated when the function increment finishes, so when we try to access it afterwards compiler is not able to reference the pointer.
Safe ways to return a pointer from a function
- Return variables are either created using the keyword static or created dynamically at run time because such variables exist in memory beyond the scope of the called function.
- Use arguments that are passed by their reference because such functions exist in the calling function scope.
Example: Passing and returning values from functions using pointer in C
Output
Referencing and Dereferencing of Function Pointer in C
Suppose we want to create a sorting function. It makes more sense to allow the function’s caller to decide the order in which values are sorted (ascending, descending, etc). One way is to provide a flag in the function argument to decide what to do, but this is not flexible.
Another way is to provide user flexibility to pass a function in our sort function. This function can take two values as input and perform a comparison between them. A syntax for our new function will look like.
For example, if we want to sort an array in decreasing order a comparison function can be used as shown in figure and the function can be passed to the sorting function using function pointers.
As shown in the example we can pass functions in another function as an argument using function pointers. This way we can pass reference of function pointer in a function and dereference it later inside the function's body to create a function call.
Let us look at some examples to understand the uses of function pointers.
Example: Function pointer passed as an argument
Another way to use function pointers is by passing them to other functions as a function argument. We also call such functions as callback functions because receiving function calls them back.
Output
Here, we have created a function conditionalSum that sums two integer numbers after modifying their value based on the callback function. When the function is called in the first case, fp was pointing to function square because of which the output of function is sum of squares of the arguments. In the second function call function pointer fp points to the function cube and the sum is returned in cubic sum of the numbers.
Program to pass pointers with functions in c
Following is a program where we passed pointer in function argument and, changes made on it inside the function are reflected in the calling function.
Output
Here, because we have passed current salary with a pointer the changes made on the address pointed by the pointer are reflected when we leave the scope of the function incrementSalary. This will not happen if a normal variable is passed to the function instead of a pointer variable.
We can also pass the array to functions using pointers as shown in the example
Output
Compile these codes online with this Free Online C Compiler.
Conclusion
- Instructions inside function also live in memory and have a memory address. We can use pointers to reference functions in C. Such pointers which hold a reference to a function are called function pointers.
- Function pointers in C are required to be declared before referencing them to a function. Function pointer declaration includes function return type and data types of function arguments, which it will point to.
- Functions can both accept and return values as pointers. When pointers are passed as function argument changes made on them persist outside the function scope. This way of passing pointers to function arguments is called pass by reference.
- When functions return a pointer, extra care should be taken not to return any address whose value might not exist outside the function scope. In such cases you such return pointer to variables that are passed to the function by reference or have global scope or are created dynamically inside the function.
- Function pointers in C can be used to create function calls to which they point. This allows programmers to pass them to functions as arguments. Such functions passed as an argument to other functions are also called callback functions.