Implicit Type Conversion in C
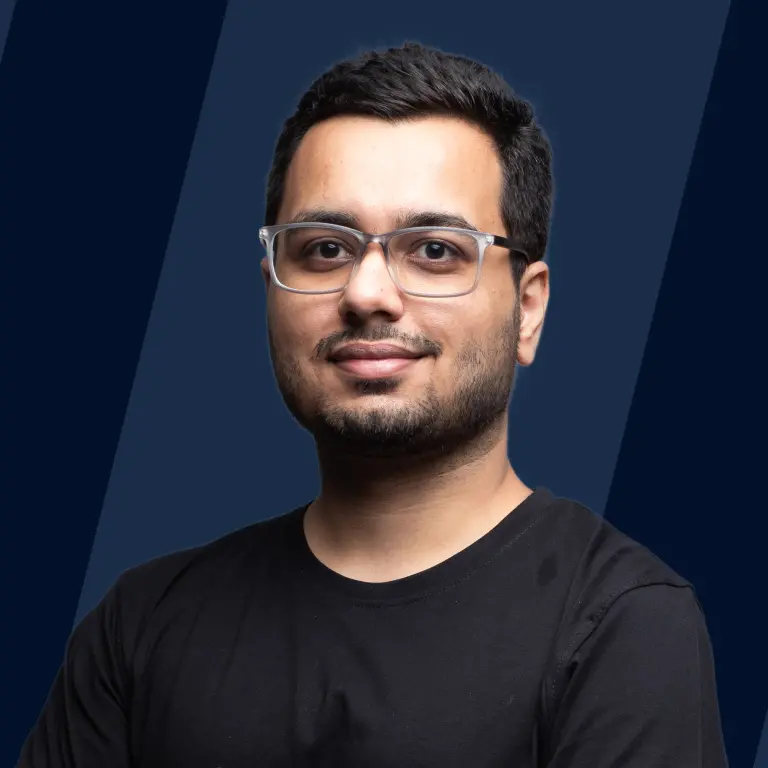
Overview
Implicit type conversion in C language is the conversion of one data type into another datatype by the compiler during the execution of the program. It is also called automatic type conversion. It generally takes place when more than one data types are present in an arithmetic expression and all the data types are converted to the data type of the highest rank to avoid the data loss in that expression according to the conversion hierarchy of the datatypes that are maintained by the C language.
What is Type Conversion in C?
Note : Before going through the type conversion the prerequisite knowledge of Datatypes in C is required
Type conversion is the method to convert one data type into another data type. When we write a C program, we declare some variables and constants, if we perform some operation or write some expression, the result of that expression may be of some other type. So to sustain the type we need to do typecasting or type conversion in C programming.
To understand this, let's take a look at a C program.
Code:
Output:
Here in this C program, variable 'a' is declared as float and 'b' is declared as int. To store the value of addition of float 'a' and integer '3' inside integer 'b' we have to perform typecasting on 'a' and convert it to integer for that particular expression.
Types of type conversion
There are two types of type conversion in the C language.
- Implicit type conversion.
- Explicit type conversion.
1. Implicit Type Conversion
Let's start with a real-life example. Let's say we have 10 mobile phones and 2 chargers, if we add them it will be 10 mobiles and 2 chargers it will not become 12 mobiles or 12 chargers because they both are different objects. Just like that in C language, different data types are different objects, so they cannot be added or any arithmetic operation is not possible until and unless we convert them to the same data type.
The implicit type conversion takes place when more than one data type is present in an expression. It is done by the compiler itself it is also called automatic type conversion. Here the automatic type conversion takes place in order to prevent data loss, as the datatypes are upgraded to the variable with datatype having the largest value.
Eg. If we add one integer and float so one of them has to become float because there is a conversion hierarchy according to which conversion happens.
The conversion hierarchy is as follows:
Here short is converted to int, int to unsigned int, and so on. But if we reverse the hierarchy, then it is not possible with the C compiler.
Let's take a C program for example.
Code:
Output:
The breakdown above C program according to the compiler is given below:
The output in the image shows the value stored in the variable 'd', which is declared as double. However, when the same expression is stored as an integer, the value will be ignored after the decimal point.
2. Explicit Type Conversion
Let's start with an example. If we perform an arithmetic operation on two same types of datatype variables so the output will be in the same data type. But there are some operations like the division that can give us output in float or double.
Eg.
Here the expected output was 6.66 but a and b were integers so the output came as 6 integer. But if we need 6.66 as output we need explicit type conversion.
Explicit type conversion refers to the type conversion performed by a programmer by modifying the data type of an expression using the type cast operator.
The explicit type conversion is also called type casting in other languages. It is done by the programmer, unlike implicit type conversion which is done by the compiler.
Syntax:
The explicit type conversion is possible because of the cast operator and it temporarily converts the variable's datatype into a different datatype. Here the data type could be of any type, even the user-defined data type and the expression could be constant, variable or an expression.
C program to explain explicit type conversion:
Code:
Output:
Now, if we break down the above example. It converts a into float temporarily in the second expression and now according to the implicit type conversion the output of int and float is float. The value of the expression becomes 6.6667 and gets assigned to x which is already declared as x.
But, there are a few points that should be noted.
-
The datatype conversion of an (int) into (float) is temporary, the integer variable will be treated as int if we use it in any other expression.
-
The value of x = b/(float)a is not equal to x = float(b/a) because in the second case the arithmetic operation happens before datatype conversion whose output is 6.000 and it will be assigned to x as a float with the value 6.000 and result will remain same.
Occurrences of Implicit Type Conversion in C.
We call implicit type conversion automatic type conversion, there are few occurrences of it in the C program. A few are mentioned below :
- Conversion Rank
- Conversions in Assignment Expressions
- Promotion
- Demotion
- Conversions in other Binary Expressions
1. Conversion Rank
There is a 1-9 scale of the datatype from lowest priority to highest i.e. their rank. The program follows the conversion according to the conversion rank as shown below.
Let's take an example to understand how the conversion rank works :
Code:
Output:
Here the variable 'a' is declared as char, while the variable 'b' is declared as boolean, which has a lower conversion rank than char. When we add the two, we get char and the result becomes ('S' + 1), which is T. So the output we get is T.
2. Conversions in Assignment Expressions
Starting with the assignment operator = there are two sides to it first is (left) of '=' and (right). So the occurrence of operands has the following conditions.
- If the operands are different, then with respect to the conversion rank the right-side operand data type will be converted to the left-hand side operand datatype.
Eg.
Here the operand on the left side has a higher rank, so the right-hand operand will be converted to left-hand type i.e. integer and it will take the ASCII value of x i.e. 120.
- If the right operand has a higher rank than the left side, then it will be demoted to the operand data type of the left side
Eg.
Here the right operand is int and left is float so the value 112.3 will be converted to 112 as an integer.
Let's take an example that explains it better :
Code:
Output:
Here, the char a is converted into int and the ASCII value of a which is 97, is assigned to int a. The 112.3 value is converted to int 112, where the digits after the decimals are excluded.
When we perform arithmetic operations on variables of different data types, then the declared variable can be demoted or promoted according to the conversion rank.
3. Promotion
In promotion, the datatype of lower rank is converted into a datatype of higher. If we take the example of char,int, and float so char is a subset of int and int is a subset to float so if we convert accordingly then the promotion generally does not create any problems and it is done to avoid data loss.
Let's take a real-life example, if we have one small box and one big box, when we put a small box into a big box, it will easily fit as here we are promoting but if we reverse the condition it will not fit because here we are demoting.
Let's take the C program to understand it better :
Code:
Output:
In this program, variable 'a' is declared as an integer, and the character 'x' is stored inside it. But can 'x' be stored inside an 'integer'? Therefore, the ASCII value of 'x' is stored inside variable 'a' as we can see in the output while we print the value of 'a' using C program.
4. Demotion
In demotion when the data type of the higher rank is converted into the data type of the lower rank. If we take the example of char,int and float the float is a superset of int and int is a superset of char. So demotion can create problems and give unpredictable results.
Let's take an example of char and int. when a char is converted to int it takes the value of char as ASCII value but the ASCII values are limited to 256 if we demote int 257 to char, it will overflow and the compiler will give warnings.
Let's take a C program to understand it better :
Code:
Output:
5. Conversions in other Binary Expressions.
When the two operands are separated by the one operator is called a binary operator and the expression is called a binary expression. There are different rules for binary expressions as they can also become complex like the points we covered in Demotion.
Let's take an example :
Code:
Output:
In this program, there are 3 binary expressions. At first, the variable ‘e’ is declared as a char, while the variable ‘a’ is declared as a boolean, which has a lower conversion rank than char. When we add the two, we get char and the result becomes (‘V’ + 1), which is W. So the output we get is W. In the second case, while multiplying integer and short integer, we get integer value as short integer has lower conversion rank than an integer. In the third case, while multiplying long double and char, the ASCII value of char is multiplied with the long double value and the output is long double.
Rules of implicit conversion
1. Short
Datatype 1 | Datatype 2 | Result |
---|---|---|
short | short | short |
short | int | int |
short | long | long |
short | float | float |
short | double | double |
short | long double | long double |
short | char | short |
2. int
Datatype 1 | Datatype 2 | Result |
---|---|---|
int | int | int |
int | long | long |
int | float | float |
int | double | double |
int | long double | long double |
int | char | int |
3. long
Datatype 1 | Datatype 2 | Result |
---|---|---|
long | long | long |
long | float | float |
long | double | double |
long | long double | long double |
long | char | long |
4. float
Datatype 1 | Datatype 2 | Result |
---|---|---|
float | float | float |
float | double | double |
float | long double | long double |
float | char | float |
5. double
Datatype 1 | Datatype 2 | Result |
---|---|---|
double | double | float |
double | long double | double |
double | char | long double |
6. long double
Datatype 1 | Datatype 2 | Result |
---|---|---|
long double | long double | long double |
long double | char | long double |
7. char
Datatype 1 | Datatype 2 | Result |
---|---|---|
char | char | char |
Important Points about Implicit Conversions.
- If one operand is of higher rank and the other operand is of the same rank or lower rank then the output of the operation will be that higher rank. (Refer to the rules in the above tables for conversion). It is also called promotion of type.
- When the datatype double is converted into float then the digits a rounded off.
- When any arithmetic operation between int and char happens then the char is converted to its ASCII value and then the operation is performed.
- When the int type is converted into to float type or the float type is converted to double type then there is no increase in the accuracy.
Advantages of Type Conversion
- If the output of any arithmetic operation between two similar operands is a different operand then to store it in proper datatype operand we use type conversion.
- There is more accuracy in the results when we use type conversion.
- The arithmetic operations with different data types can be computed easily.
- We can refer to the conversion rank hierarchy before performing operations for better results.
Conclusion.
- The implicit type conversion is also called automatic type conversion as it is automatically done by a compiler.
- The type conversion which is done by the programmer himself is called explicit type conversion.
- There is conversion rank according to which the implicit type conversion happens in C.
- There are many occurrences of implicit type conversion like in Assignment Expressions, Binary Expressions, etc.