Macros in C
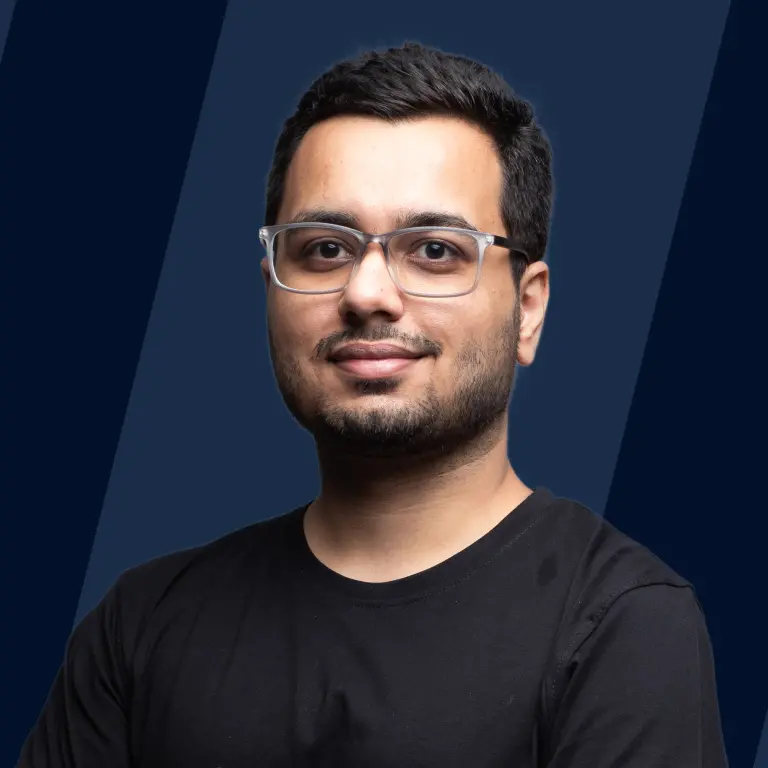
Overview
Macro in C programming is known as the piece of code defined with the help of the #define directive. Macros in C are very useful at multiple places to replace the piece of code with a single value of the macro. Macros have multiple types and there are some predefined macros as well.
Introduction to Macros in C Language
Suppose we are working on an application in C language and there is one value or an object or segment of code which we require so many times in our code then with the help of macros we can define it once and use it many times.
Macros are one of the convenient ways to write robust and scalable code.
In this article, we will explore the use of macros in C language, their different types and use cases.
What are Macros in C language?
The macro in C language is known as the piece of code which can be replaced by the macro value. The macro is defined with the help of #define preprocessor directive and the macro doesn’t end with a semicolon(;). Macro is just a name given to certain values or expressions it doesn't point to any memory location.
Whenever the compiler encounters the macro, it replaces the macro name with the macro value. Two macros could not have the same name.
The syntax of the macro is as shown in the following figure. Here, we will have the three components:
- #define - Preprocessor Directive
- PI - Macro Name
- 3.14 - Macro Value
We will take a deeper look at what preprocessor directive is in the later part of this article, but first, let's try to understand macro with the help of code example-
Code:
Output:
In the above code example, we wrote a program to calculate the area of a circle with the help of a given radius.
We defined the PI constant as a macro with the value 3.14.
Here macro name PI at the 11th line got replaced with the macro value 3.14 as we saw in the definition, and we got the area of a circle as the output.
Now, this replacement of value occurs due to the preprocessor and preprocessor directive #define.
What are Preprocessor and Preprocessor Directives in C Programming?
Whenever we write a certain code in C language, it goes through the process of compilation, where it gets converted from source code to machine-understandable code.
But before the compilation process, the source code goes through preprocessing, which is done by the preprocessor.
As the above image shows preprocessor checks for preprocessing directives in the C program.
If there are some preprocessing directives found, then certain actions are taken on them by the preprocessor.
There are multiple types of preprocessors such as #define, #if, #error, and #warning etc., They all start with the # symbol.
To define the macro, we use the #define preprocessing directive, which performs the action to replace the macro name with the macro value at the time of preprocessing.
Let's see what are the different types of macros present in the C language.
Types of Macros in C Language
The macro in C language is classified based on the different types of values that it replaces.
Let's see the different types of macros one by one.
Object like Macros in C
An object like macros in C programming is simply the macros that get replaced by certain values or segments of code.
In the above example, in the introduction, we saw the macro with the name PI and value 3.14 it is an example of an object like macros.
We can define macros with different datatypes as shown as follows but we can't use the same macro names for two different values -
Function Like Macros in C
In the function like macros are very similar to the actual function in C programming.
We can pass the arguments with the macro name and perform the actions in the code segment.
In macros, there is no type checking of arguments so we can use it as an advantage to pass different datatypes in same macros in C language.
Let's consider the following code example which is the modification of the previous code -
Code:
Output:
See in the above code, we added the function-like macro at line no. 7.
The macro name is Area which takes the argument r as a radius that we have passed at line no. 15 and line no. 22.
At the time of preprocessing the value Area(radius) gets replaced with the processed macro value and it is assigned to the area variable.
At line no. 15, we passed the value of radius as a float and at line no. 22 we passed the value of radius as a float of type integer. So macros gave us the advantage to use the same macro for different datatypes because there is no type checking of arguments.
Chain Like Macros in C
When we use one macro inside another macro, then it is known as the chain-like macro.
We already saw the example of a chain-like macro in the above code example where we used the PI in Area. Let's discuss it a little more -
In the above code snippet, we can see we used the object like macro PI inside the function like macro Area.
Here first parent macro gets expanded i.e. the functional macro Area and then the child macro gets expanded i.e. PI. This way when we use one macro inside another macro, it is called a chain macro.
Types of macros in Nutshell:
Sr. No. | Macro Name | Description |
---|---|---|
1 | Object Like | Value or code segment gets replaced with macro name |
2 | Function Like | The macro which takes an argument and acts as a function |
3 | Chain Like | The macro inside another macro |
Till now, we have seen how to define the macros, their types and how to use them. But some macros come with the C language itself and which we can directly use in the C program.
Predefined Macros in C Language
There are some predefined macros in C that we can directly use in the program and they aren't modifiable.
We can perform so many different tasks with predefined macros which isn't possible with the normal C programming.
These macros are as follows:
Predefined Macros and their features:
Sr. No. | Macro | Feature |
---|---|---|
1 | __LINE__ Macro | It contains the current line number on which this macro is used. |
2 | __FILE__ Macro | It contains the name of the file where the current program is present. |
3 | __DATE__ Macro | It contains the current date in MMM DD YYYY format. |
4 | __TIME__ Macro | It contains the current time in HH:MM |
5 | __STDC__ Macro | It is defined as 1 when there is a successful compilation. |
Let's see the working of all of these predefined macros with the help of a coding example-
Code:
Output
You can run and check your code in this Online C Compiler.
Conclusion
- Macro is a piece of code or value that is replaced with the macro name before the execution of the program.
- Preprocessor performs different actions on preprocessor directives and for the macro definition, we use the #define preprocessor directive.
- The work of #define is that it replaces the macro body with the macro value at the time of preprocessing.
- It is a good idea to use the macros in code as per the requirement and utilize the different types of macros.
- Predefined macros can do so many things which aren't possible with normal C programming.