Nested If else Statement in C
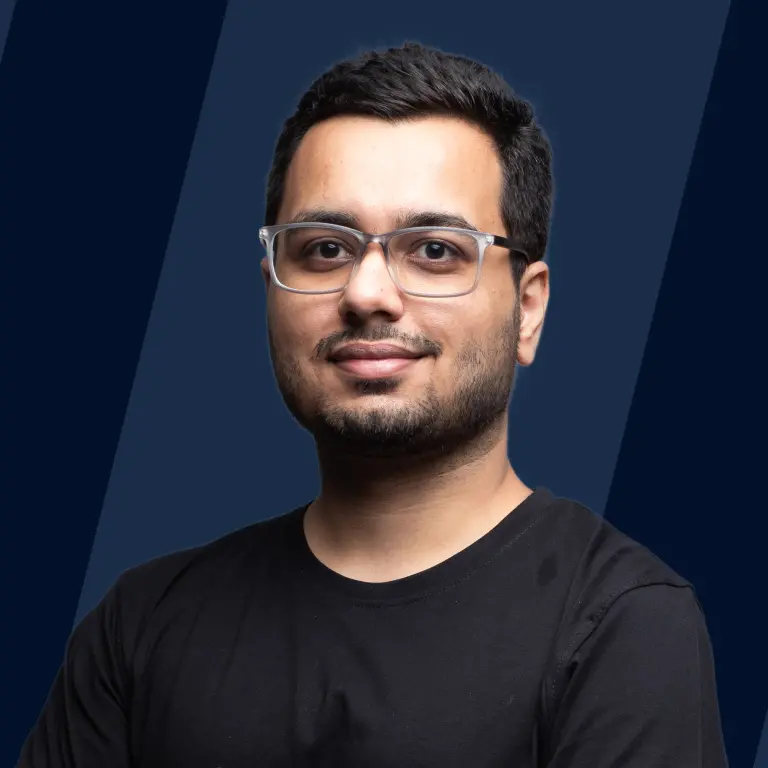
Overview
If else statements are used for decision making by specifying which block of code is to be executed when a certain condition is met. Nested if-else statements are just if-else statements inside other if-else statements to provide better decision making.
To understand this topic, you should have some knowledge of the following C Programming topics:
Introduction to Nested if else Statement in C
Whenever we need to make a decision about something, we ask ourselves questions, and based on the conditions, we evaluate which path to take. This is majorly the case when we have many options, and only one of them is to be picked.
In programming languages, to implement this very case, we use if-else statements. Let's look at a quick recap of if and else statements before introducing nested if else statement in C.
Recap of If Else Statements
Whenever we need to make different decisions based on specific conditions being satisfied in programming, we use if-else statements. These statements are called decision making statements due to this very reason. They help in making decisions based on conditions and hence contribute to deciding the flow of the code.
If the condition inside if is true, the statements inside the if bracket is executed. If the condition turns out to be false, the if block is skipped, and the statements inside the else block are executed.
For example, given a number we need to check if it is even or not. That means we need to make a decision of printing even or odd based on the parity of the number given. For this, we will use if and else statement.
The actual code in C would be :
Input
Output
You can run and check your code in this Online C Compiler.
Nested If Else Statement in C
We already saw how useful if and else statements are, but what if we need to check for more conditions even when one condition is satisfied?
For example, if we need to analyse if the number is even or odd, and then if it is even, whether it is divisible by 4 or not, and if it is odd, whether it is divisible by 3 or not. In such a case, only one if and else statement wouldn’t suffice.
First, we will check with one if-else statement whether the number is even or odd. Then in the if block, that means if the number was even, we would have to include another if and else statement checking if it is divisible by 4 or not, and similarly in the else block, we would have to include another if and else statement checking if the number is divisible by 3 or not.
Including numerous if-else statements inside an if and else statement is called nesting. The second if and else statements are said to be nested inside the first if and else statement.
This is why C language allows nesting of if and else statements. These are called nested if else statements and provide clearer decision making when some extra conditions are needed to be checked inside the initial conditions like in the previous example.
Syntax of Nested If Else Statement in C
The syntax for Nested If Else Statement in C will be :
The code in C for the above described example where we need to analyse if the number is even or odd, and then if it is even, whether it is divisible by 4 or not, and if it is odd, whether it is divisible by 3 or not will be :
Input
Output
Flowchart of Nested If Else Statement in C
The flowchart for nested if-else statements is shown below in the diagram.
Working of Nested If Statement in C
How does the nested if statement provides flow control and decision making in programming? Let's look at the working of Nested If Statement in C to understand this better.
Taking the previous example, we have the first IF statement which evaluates if n is even. If this n is even, that means the expression n % 2 == 0 evaluates to true, we enter the if block. Here we have our nested if statement which evaluates if n is divisible by 4. If the expression n % 4 == 0evaluates to true, we enter the nested if statement block. Here we print that the number is even and divisible by 4. If the expression n % 4 == 0 was evaluated to be false, we enter the nested else statement and print that the number is even but not divisible by 4.
Similarly, if the expression n % 2 == 0 evaluates to false, we enter the first else block, skipping the if part as the condition is false, and we check the condition of the nested if statement. If the expression n % 3 == 0 evaluates to true, we enter the nested if statement block. Here we print that the number is odd and divisible by 3. If the expression n % 3 == 0 was evaluated to be false, we enter the nested else statement and print that the number is even but not divisible by 3.
The thing to note here is when we enter the if block, the else block is ignored and if we enter the else block, the if block is ignored, and this is exactly how if else statements help in decision making. Only a particular block of code is executed based on what conditions are being satisfied.
Nested if statement provides better decision making when other conditions are to be analyzed inside the first condition, and so we can include more than one nested if statement inside an if statement to get the required result.
Examples for Nested If Else Statement in C
We have taken a look at the syntax and working of nested if-else statements in C. Let us go through some examples to get a better idea.
Example 1 : Check if three numbers are equal
Given three numbers, we need to check if all of them are equal in value or not.
We will use nested if else statement to check this. First, we check that out of the three numbers, whether the first two are equal. If they are, then we go inside the nested if to check whether the third is equal to them. If yes, then all are equal else they are not equal. And if the first two numbers themselves are not equal to each other, no need to check further, we can simply output No.
The code in C is given below.
Input 1
Output 1
Input 2
Output 2
Example 2 : Which number is greatest among three numbers
Given three numbers, find the greatest among them and output its value.
We will use nested if else statement in C to check this. First, we check if the first number is greater than the second number. If this is true, it means that maybe the first number might be the greatest among all three. To check whether this might be the case, we go into the nested if else loop. We again check if the first number is greater than the third number, then it is the largest of them all, else the third number is the largest of them.
If the first number is smaller than the second number, we enter the else block. In this case, the second number might be the greatest of them all. To check whether this might be the case, we go into the nested if else loop. We again check if the second number is greater than the third number, then it is the largest of them all, else the third number is the largest of them.
The code in C is given below.
Input 1
Output 1
Example 3 : Grade of a student based on marks
Given the grade of a student, find whether he/she passes or fails and if he/she passes, output the grade achieved.
First, according to the marks of the student, we need to check whether the student is pass or fail. So, the first if statement analyses whether the student passed or not. If the student passes, we next need to check what grade the student received based on his marks, for this we will use nested if-else statements. If the student fails, there is no other case possible, so we just output the F grade.
The code in C is given below.
Input 1
Output 1
Input 2
Output 2
Input 3
Output 3
Input 4
Output 4
Example 4 : Check if a year is a leap year
Given a year, check if it is a leap year or not.
A leap year is a year that has 366 days, instead of 365 days. It has one day extra in the month of February. Leap years occur once in 4 years, so any year that is completely divisible by four should be a leap year. But this is not always true. If there is some year and it is divisible by hundred, it will be a leap year only if it is also divisible by four hundred.
This means that the first if statement will check if the given year is divisible by 4. If it is not, then it cannot be a leap year. But, if it is, then we need to check if the year is divisible by 100. If it is not divisible by 100, then it is surely a leap year. But, if it is divisible by 100, then we need to check if it is also divisible by 400. If it is, then it is a leap year, else it is not a leap year.
Looking closely at the explanation, we would have to use two nested if-else statements in this code for the desired correct solution.
The code in C is given below.
Input 1
Output 1
Input 2
Output 2
Input 3
Output 3
Input 4
Output 4
Conclusion
- In programming languages, if else statements are used for decision making. They determine the flow of code by specifying different operations in different cases.
- Including numerous if-else statements inside an if and else statement is called nesting. The second if and else statements are said to be nested inside the first if and else statement.
- When we enter the if block, the else block is ignored and if we enter the else block, the if block is ignored. Nested if else follow the same working.
- C language allows nesting of if-else statements to facilitate better decision making.