Pointer Declaration in C
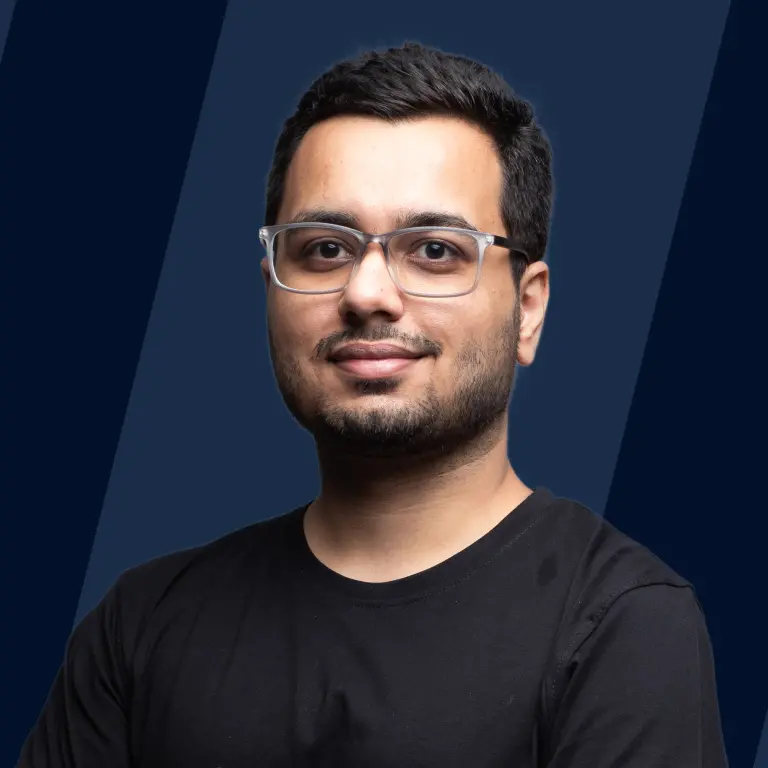
Pointers in C are crucial for direct memory manipulation, serving as variables that store memory addresses. They enable referencing specific memory locations, enhancing programming flexibility. This guide delves into declaring and initializing pointers with addresses of other variables, elucidating their fundamental role in C programming.
Look at the image below to understand what happens when a pointer is declared in C and how it relates to a variable.
How to declare a pointer in C?
Syntax:
Example:
Explanation:
For pointer declaration in C, you must make sure that the data type you're using is a valid C data type and that the pointer and the variable to which the pointer variable points must have the same data type.
For example, if you want a pointer to point to a variable of data type int, i.e. int var=5 then the pointer must also be of datatype 'int', i.e. int *ptr1=&var. The * symbol indicates that the variable is a pointer. To declare a variable as a pointer, you must prefix it with *.
In the example above, we have done a pointer declaration and named ptr1 with the data type integer.
How to initialize a pointer in C?
There are ways of initializing a pointer in C once the pointer declaration is done. Look at the example below to understand.
Example:
Method 1
We make use of the reference operator, i.e. '&' to get the memory address of a variable. It is also known as the address-of-operator. Look at the figure below to understand what happens:
Method 2
Let us consider the case when we want another pointer to point to the same variable, then, in that case, we can make use of this method to do the same instead of doing method 1 all over again i.e. we simply assign the old pointer to the new pointer. Look at the figure below to understand what happens:
Explanation:
& is a reference operator, which means it is used to get the memory location of the variable. So 'a' is an integer variable and by doing &a, we are getting the location where a is stored in the memory and then letting the pointer point to that location. This is the first method of initializing a pointer in C.
The second method is to initialize a pointer and assign the old pointer to this new pointer.
How to Access a Pointer in C?
You can access both the address in memory where the pointer points to and the value it points to as well. To do this, let us first understand what a dereference operator is i.e. '*'.
The process of getting a value from a memory address pointed by a pointer is known as dereferencing. To get the value pointed by a memory address, we utilize the unary operator, *.
Let us now see an example:
Example:
Output
See the image below to understand this further:
Note: When printf("%p\n",ptr1); is called the output is as that is the memory address stored by the pointer ptr1.
Explanation:
Simply printing ptr1 gets you the location in memory that the pointer points to, but to get the value it points to, you need to make use of a dereference operator(*). The process of getting a value from a memory address pointed by a pointer is known as dereferencing.
C program to Create, Initialize and Access a Pointer
Let us combine everything that we have learnt and write a combined code for declaring, initializing and accessing a pointer.
Code:
Output:
Look at the figure below to get a detailed explanation of the above output:
Points to Remember While Using Pointers
Here are a few points to remember for your next program involving pointers:
- The * indicates that the variable is a pointer when declaring or initializing it.
- The address of any variable is specified by using the & symbol before the variable name.
- The address of a variable is stored in the pointer variable.
- It is important to remember that the data type of the variable and the pointer should be the same during pointer declaration in C.
- * is also used to access the value of a specific address.
- There are multiple levels of a pointer. The level of the pointer is determined by the number of asterisks preceding the pointer variable at the time of pointer declaration in C. Level 1(int *ptr1=&a) would be a pointer to a variable. Level 2(int **ptr2=&a) would be a pointer to a pointer to a variable, and so on.
Conclusion
Pointers are widely used by C programmers due to their numerous advantages. Some of these include:
- When dealing with arrays and structures, pointers are more efficient.
- Multiple values are returned from a function using pointers.
- To gain a reference to a variable or function, we utilize pointers.
- In C, pointers enable dynamic memory allocation (variable creation at runtime). That is, without question, the most significant advantage of pointers.
- The use of pointers speeds up the execution of a program because of the direct access to memory location.
Pointers have several applications. Some of them include accessing array elements, dynamic memory allocation, and implementing data structures. It is thus easy to conclude that pointers are the heart of C programming!