What is a Pointer in C?
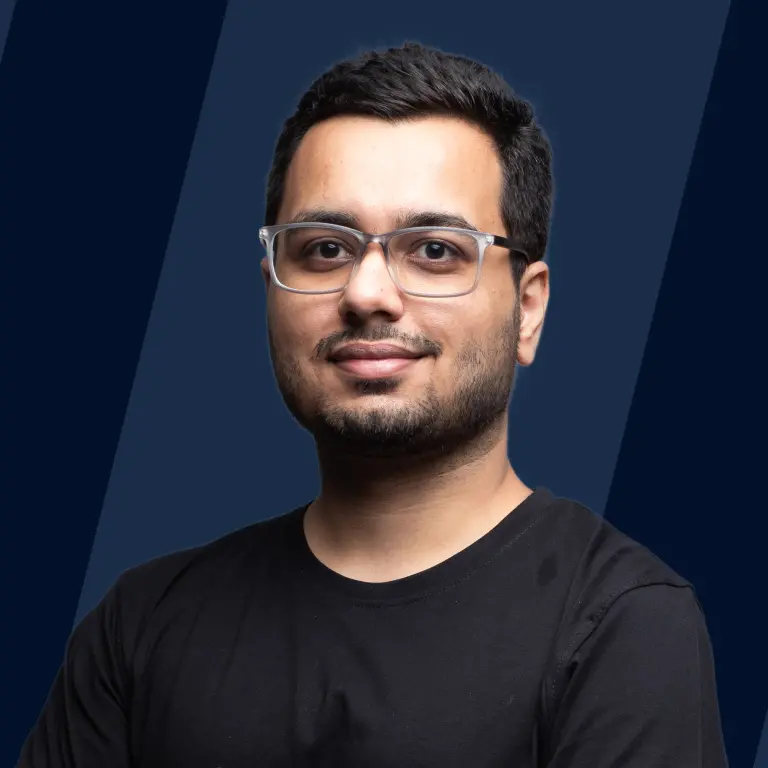
Overview
A pointer in C is a variable like any other variable but that holds the address of a variable. We use them when we want to allocate some memory on the heap which is also known as dynamic memory allocation.
What is a Pointer in C?
A Pointer is a variable that holds the memory address of another variable. When we declare a variable of a specific data type we assign some of the memory for the variable where it can store it's data. By defualt the memory allocation is in stack. Using different functions(malloc in C) or key-words(new in C++ , Java etc) we can allocate memory for a variable in the heap section(also called as dynamic memory allocation). One of the major advantages of allocating memory in heap is it allows you to access variables globally. Simply accessing the pointer that stores the address of the variable declared in heap we can directly manipulate the data of the variable in heap.
How to Use Pointers in C?
As of now we know what a pointer is. Now let's look how to use a pointer in C--
For using a pointer in C , we need to use a unary operator '*' before the name of the pointer variable to let the compiler know that this is a pointer variable.
Now to assign address of another variable we use another unary operator that is the '&' operator to assign the address of a variable to pointer variable.
Note that the '*' operator that we used to declare a variable as pointer is also used to get the value at that address that the pointer holds. This is also known as dereferrencing.
Output
Here in the printf function , we dereferrenced the pointer variable p to get the value at the address which the pointer variable p is holding.
Syntax of Pointers in C
To declare a pointer variabla we use "*" before the variable name.
Here p is a pointer variable.
When we declare p , an empty block is created in heap sections having value '0' i.e. it currently has no addressess in it to store.
Output
Now if we want our pointer to have an address we need to declare another variable of the same data type as of pointer and assign the address of the variable to a pointer using "&" operator.
If we try to print the value of p , we get a hexadecimal number on the output screen which is nothing but the address of the variable n.
Output
Now if you want to check whether that address contains the correct value or not(i.e. 10) , simply print the value at address which is done using "*" again and is called as dereferrencing.
Output
Types of Pointers in C
There are basically 8 types of pointers in C.
Null Pointer in C
If we don't initialize a pointer after declaration then by default the pointer is a Null pointer . We can also explicitly do this by assigning the NULL value at the time of pointer declaration. This method is useful when you do not assign any address to the pointer.
Output
Void Pointer in C
The void pointer is a generic pointer that does not have any data type assosiated with it. The datatype of void pointer can be of any type and can be typecast to any type.
Output
The output may vary depending upon the compiler.
Wild Pointer in C
Wild pointers are basically uninitialized pointers that points to arbitrary memory location and may cause a program to crash or behave badly.
Dangling Pointer in C
A dangling pointer is a pointer that is pointing to a memory location that has been deleted or released. Let's understand with an example---
When we create a pointer "ptr" it gets some memory in stack of the same size as its data type. Then we called malloc function to create a memory in heap and assign the address of that memory to the pointer variable. Now the magic happens, when we call "free" on "ptr" the memory address of the heap got deleted and now "ptr" is pointing to a location that has been deleted. This is called dangling pointer. Now when we aasign "NULL" to "ptr" it no more remains a dangling pointer & becomes a NULL pointer.
Complex Pointer in C
Complex pointers contains of () , [] , * , Identifier , Data type with different associativity and precedence. () & [] have the highest precedence & associativity from left to right followed by * & Identifier having precedence 2 & associativity from right to left and Data Type having the least precedence of all.
Example
- Here '()' is used to declare and define the function.
- '[]' is used as an array subscript operator.
- '*' is the pointer operator.
- 'Identifier' is the name of a pointer.
- 'Data type' is the type of variable.
Near Pointer in C
Near Pointer is a pointer that can access small size of data(usually 64kb) & can utilize bit address(generally up to 16 bits) in a given section of memory that is 16 bit enabled. Each address in a memory is of single byte i.e. eight bits of storage & for large data the data can be stored in sequence of addressess.
Output
Far Pointer in C
A far pointer is a pointer (generally 32 bit) that basically uses two registers for storing segment & offset addresses and can be used to access memory outside current segment where a register is able to hold 4 bytes(1 byte being equal to 8 bits).
Output
Huge Pointer in C
Both Huge and Far pointers are typically 32 bit and both of them can access memory outside current segment. The only difference is that for far pointer the segment part cannot be modified i.e. the segment part for far pointer is fixed while in huge pointer it can be changed easily without indulging in segment work round.
Examples of Pointers in C
In the above portions of this article we got know many things about pointers such as it's uses , syntax , how to assign addressess to a pointer and also to get the value of that location that the pointer is pointing to. Now let's see all of this working together---
Here we created a variable n & assigned its address to a pointer variable p and then print its value and addressess.
Output
We can see that the address of n & the location where the pointer variable is pointing have the same value i.e. p is holding the address of n. Now if we print both we can see that the value of n & value at the location that p is holding(also called dereferrencing) is same. From here we can conclude that p is perfectly pointing to the addresses of n.
To get a more clear understanding , if we change the value of n , you will see the value at the location p is pointing will also change & vice versa.
Output
Benefits Of Pointers in C
One of the major advantages of using pointers is dynamic memory allocation which acclerates the program execution when we have any task of updating the variable in different time frames as the variable is directly accessible.
With the help of pointers we create different data structures such as Linked List , Trees etc. It also helps to return more than one function value with the help of structures and classes.
Conclusion
- In this article we get to learn about pointers , its syntax and its types.
- We also learnt about benefits of pointers in C and some differences between stack & heap memory and different types of Pointers in C as well.