sizeof() in C
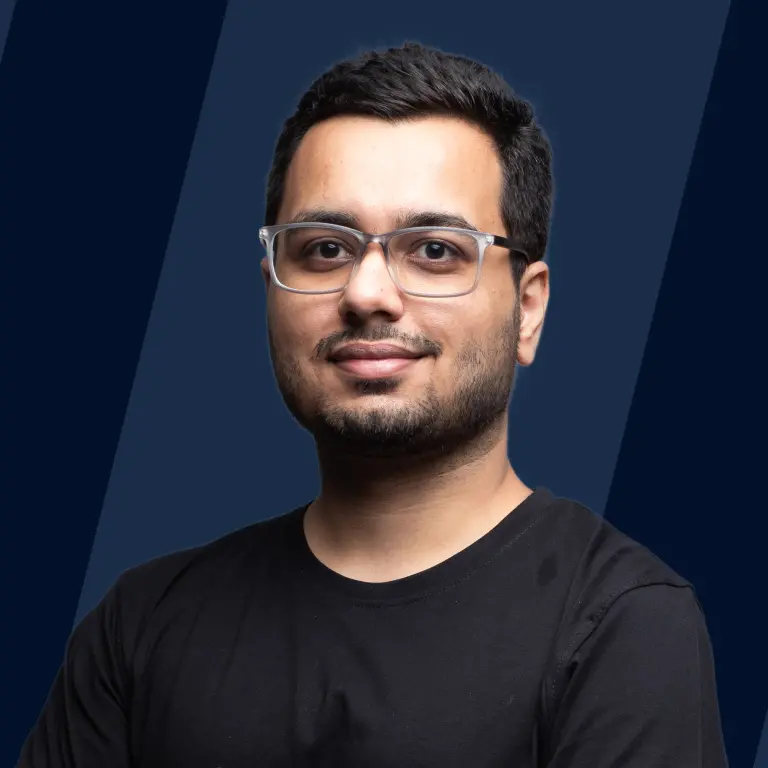
The sizeof() operator in C computes the memory size, in bytes, of variables, pointers, arrays, or expressions. It's essential for understanding the memory allocations. Let's delve into the workings of the sizeof() operator in C.
sizeof() Operator in C
The sizeof() operator is commonly used in C, it is a compile-time unary operator(which simply means that the function only executes at compile time and has only one operand). A compile time unary operator means that the sizeof() operator calculates the amount of memory space required by the data type or expression at compile time and as it operates on only one input, it is a unary operator as well. The sizeof() operator tells us the amount of memory space in bytes required of its operand when the code is compiled.
Mainly, programs know the storage size of the primitive data types, although when the same program is implemented on a different machine the storage size may vary. Let us look at an example where such a thing might happen:
In the above example, we utilize the sizeof() operator, which is applied to an int data typecast. We use the malloc() method to allocate memory dynamically and return the pointer referring to that memory. The memory space is then multiplied by 10, where the memory space originally represented the number of bytes held by the int data type.
Now the catch over here is that the output might alter depending on the computer, for example, a 32-bit operating system will produce different results, whereas a 64-bit operating system would produce different results for the same data types. This happens due to the fact that the 64-bit machines, can store int values greater than and upto . Hence in a 64-bit machine, the value does not overflow, unlike it may overflow in a 32-bit machine.
A 32-bit system can access different memory addresses whereas a 64-bit system can access different memory addresses
The sizeof() operator in C operates differently depending on the operand(data-type, variable, array, pointer or expression) which is passed to it, whose memory space in bytes is to be found:
- Operand is a data type
- Operand is a variable name
- Operand is an expression
- Operand is a pointer
Let us take a look at the syntax of the sizeof() operator in C.
Syntax of sizeof() in C
The sizeof() operator in C has various forms of representation, we can pass an array, data-type, variable, pointer, or an expression, lets now take a look at their syntax:
- When the parameter is a data-type:
datatype_variable: datatype_variable is a variable that is passed into the function to represent the data type to be used.
- When the parameter is a variable-name:
var_name: var_name is the name of the variable provided to the function to determine the number of bytes it occupies in memory.
- When the parameter is an expression:
exp: exp is the expression provided to the function to determine the number of bytes the output of the expression would occupy in the memory.
- When the parameter is an pointer:
ptr: ptr is the pointer provided to the function to determine the number of bytes the pointer would occupy in the memory.
Return Type of sizeof() Operator in C
The sizeof() operator returns an unsigned integer value which represents the amount of memory in bytes occupied by the operand. We can use the %lu access specifier to print this data type(unsigned integer) using the printf statement.
Example of sizeof in C
Output
As we can see here, the sizeof() operator prints the size of the memory that the variable x occupies and not the value it holds, which means that the variable x will occupy the same amount of memory no matter the value it holds.
What do we mean by sizeof() being a compile time operator?
We know that sizeof() is a compile time operator, it means that any code written within the parentheses of sizeof() operator is not evaluated/executed.
Let's look at an example to understand what this means:
Output
In the above program, if we try to decrement the value of the variable x, we can see that it remains the same. This happens because the decrement of x is done inside the parentheses of the sizeof() operator and since this operator is a compile-time operator, the decrement is not done during the run-time and the value of the x remains as it was before.
How Does the sizeof() Operator Work in C?
The sizeof() is a compiler built-in operator, that is there is no runtime logic (it does not have any logic such as + or - operator) behind the operator and it solely works during the compile time. The sizeof() operator is a powerful and adaptable operator for finding the storage size of an expression or a data type, measured in the number of char-sized (the amount of memory 1 char occupies which is simply 1 byte) units.
The sizeof() in C is a compiler-dependent function, since that the same expression or a complex datatype may consume different amounts of space on different machines though, the sizeof() operator will return the correct amount of space(in bytes) consumed no matter on which machine it is used. Also, if the sizeof() operator is given a parameter as an expression, it evaluates the representation size(in bytes) for the type that would result from the expression's evaluation, which is not done.
Furthermore, due to padding, it is frequently difficult to predict the sizes of complex data types such as a struct or union. Also, the use of sizeof() improves readability because it avoids unnamed numeric constants in our code.
Application of sizeof() Operator in C
The sizeof() operator is used in many different ways, let us take a look at those ways:
1. When the operand is a data type.
We can use the sizeof() operator to find the amount of memory allocated to the data types such as int, float, char, etc.
Output
Note: The sizeof() operator may return different values according to the machine, in this case, we have used it to run on a 32-bit GCC compiler.
In the above code we use the sizeof() operator inside the printf() function. The sizeof() operator returns the size of the data type which is then printed through the printf() function.
2. When the operand is a variable name.
We can use the sizeof() operator to find the amount of memory allocated to different variables.
Output
In the above code, we again use the sizeof() operator inside the printf() function. In this case, we pass a variable in the sizeof() operator which then assesses the type of data-type it is and then returns the size of the data-type which is then printed through the printf() function.
3. When the operand is an expression.
We can use the sizeof() operator to find the amount of memory that will be allocated to store the resultant of the expression.
Lets take an example:
Output
For the above code, we know that the size of int is 4 bytes and the size of double is 8 bytes. Now as num is an int type and dob is a double type, the resultant would be a double type. Therefore, the output of our code is 8 bytes.
4. When the operand is a pointer.
We can use the sizeof() operator to find the amount of memory that will be allocated to store the pointer to some variable.
Lets take an example:
Output
In the above code, we notice that the amount of memory occupied by a pointer pointing to anint type and a char type is both the same that is 8 bytes, but the memory occupied by int and char is not the same. This happens because the pointers store address of some other location and hence they require a constant amount of memory and do not depend on the type.
Note: The sizeof() operator may return the different amount of size(in bytes) occupied by a pointer on a different machine. As in a 64-bit machine, we can have different memory addresses whereas A 32-bit system can have different memory addresses. The difference in these amounts creates the difference in the amount of memory occupied by a pointer.
What is the Need for the sizeof() Operator in C?
Sizeof() operator can be applied in many instances, we will take a look at a few of them.
1. To find out memory during dynamic memory allocation.
We can use the sizeof() in C to dynamically allocate memory. Let’s say we want to allocate the memory to accommodate 10 doubles and we don’t know the size of the double in that machine, we can use the sizeof() operator in this case.
In the above code, we assign a memory block whose size is equivalent to 10 doubles. We use the malloc() function to assign that amount of memory.
2. To find the number of elements present in the array.
We can use the sizeof() operator to find the number of elements in an array. All the elements in an array are of the same data type, hence they occupy the same amount of space. Therefore, if we know the total amount of space(in bytes) occupied by an array and the amount of space(in bytes) by one of the elements, we can find the number of elements present in the array. Let us look at an example to see how this works out.
Output
In the above code, we have array arr and we are required to print the number of elements in the array. We use the sizeof() operator to find the amount of memory that the array is occupying and since all the elements will occupy the same amount of memory.
Total memory occupied by the array = (Number of elements in the array) * (Size of one element of the array)
Using the above formula we can find the number of elements in the array. We find the size of one element of the array and divide the size of the array by that. This gives us the number of elements present in the array.
Advantages of sizeof() Operator in C
Let us now discuss some of the advantages of using sizeof() in C:
- We can use the sizeof() to calculate and find the number of elements/ the size of the array.
- We can also use the sizeof() operator to find the amount of memory a user defined structure would take.
- The sizeof() operator can also be used to determine the type of system on which the program is being executed.
- We can also use the sizeof() to dynamically allocate memory to a section, it is a great tool as it helps automatically calculate and decides the amount of the memory to give on that particular machine.
Conclusion
- In this article, we learned about the sizeof() operator in C. It helps us in determining the amount of memory allocated depending on the data type or the expression passed to it.
- We discussed different syntaxes of the sizeof() operator:
- When the parameter is a data type.
- When the parameter is a variable name.
- When the parameter is an expression.
- We also discussed different ways where we can apply the sizeof() operator:
- To find the number of elements present in the array.
- To allocate a block of memory dynamically.
- In the end, we looked at some advantages of using the sizeof() operator.