Structure of a C Program
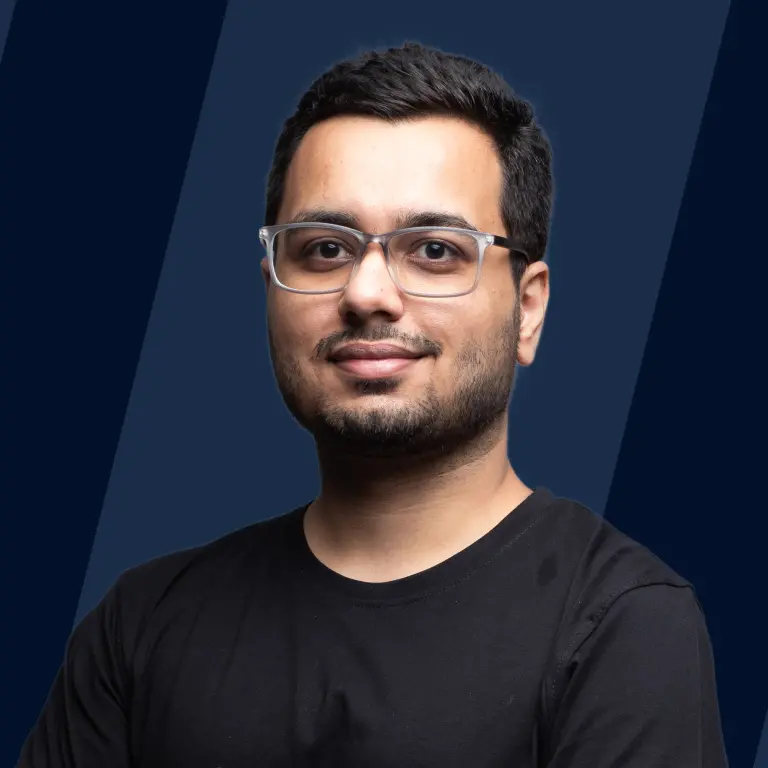
The structure of a C program, foundational to the basic structure of C programming, is categorized into six key sections: Documentation, Link, Definition, Global Declaration, Main() Function, and Subprograms. The Main() function is essential in every C program, highlighting what is required in each C program. This organization facilitates readability, modification, and documentation, providing a clear structure of C language with examples to illustrate the concepts.
Sections of the C Program
Documentation
In a C program, single-line comments can be written using two forward slashes i.e., //, and we can create multi-line comments using /* */. Here, we've used multi-line comments.
Preprocessor Section
All header files are included in this section.
A header file is a file that consists of C declarations that can be used between different files. It helps us in using others' code in our files. A copy of these header files is inserted into your code before compilation.
Definition
A preprocessor directive in C is any statement that begins with the "#" symbol. The #define is a preprocessor compiler directive used to create constants. In simple terms, #define basically allows the macro definition, which allows the use of constants in our code.
We've created a constant BORN which is assigned a value of 2000. Generally, uppercase letters are preferred for defining the constants. The above constant BORN will be replaced by 2000 throughout our code wherever used.
#define is typically used to make a source program easy to modify and compile in different execution environments.
The define statement does not end with a semicolon.
Global Declaration
This section includes all global variables, function declarations, and static variables. The variables declared in this section can be used anywhere in the program. They're accessible to all the functions of the program. Hence, they are called global variables.
We've declared our age function, which takes one integer argument and returns an integer.
Main Function
In the structure of a C program, this section contains the main function of the code. The C compiler starts execution from the main() function. It can use global variables, static variables, inbuilt functions, and user-defined functions. The return type of the main() function can be void and also not necessarily int.
Here, we've declared a variable named current and assigned the value as 2021. Then we've called the printf() function, with calls the age() function, which takes only one parameter.
Sub programs
This includes the user-defined functions called in the main() function. User-defined functions are generally written after the main() function irrespective of their order.
When the user-defined function is called from the main() function, the control of the program shifts to the called function, and when it encounters a return statement, it returns to the main() function. In this case, we've defined the age() function, which takes one parameter, i.e., the current year.
This function is called in the main function. It returns an integer to the main function.
Structure of C Program with Example
Below is the simple structure of c program with example code to calculate our age.
Code:
Output
Explanation of the Example
Sections | Description |
---|---|
/** <br>* file: age.c<br>* author: you<br>* description: program to find our age.<br>*/ | It is the comment section and part of the documentation of the code, providing a brief overview including the file name, author, and purpose of the program. |
#include <stdio.h> | This header file is included for standard input-output operations. It is part of the preprocessor directive section. |
#define BORN 2000 | This definition section defines constants to be used throughout the code, here defining the year of birth. |
int age(int current) | This global declaration section includes the declaration of the age function, which can be used anywhere within the program. |
int main(void) | main() is the entry point of the C program and is the first function to be executed. |
{...} | These curly braces denote the beginning and end of the main function and also enclose the body of the age function. |
printf("Age: %d", age(current)); | The printf() function within the main function is used to display the calculated age on the screen. |
return 0; | This statement is used to indicate that the program has executed successfully without any errors. It marks the exit point of the main function. |
int age(int current) { return current - BORN; } | This is the subprogram section where the age function is defined. It calculates the age by subtracting the defined birth year from the current year passed as an argument. |
Steps Involved in the Compilation Process
- Create the C program using a text editor.
- Compile the program to check for errors and convert to machine code.
- Execute the compiled program to carry out the specified tasks.
- Observe the output generated by the program.
FAQs
Q. What are the six main sections of a C program?
A. The six sections are Documentation, Preprocessor Section, Definition, Global Declaration, Main() Function, and Subprograms.
Q. Is the main() function mandatory in a C program?
A. Yes, the main() function is compulsory in every C program as it is the entry point for execution.
Q. Can constants be defined in C, and how?
A. Yes, constants can be defined using the #define preprocessor directive, like #define BORN 2000, to make the code more modular and easy to modify.
Q. What is the purpose of the documentation section in a C program?
A. The documentation section provides an overview of the program, including file name, author, and a brief description of its purpose, aiding in code readability and maintenance.
Conclusion
- To conclude, the basic structure of C program can be divided into six sections, namely - Documentation, Link, Definition, Global Declaration, Main() Function, and Subprograms.
- The main() function is compulsory to include in every C program, whereas the rest are optional.
- A well structure of C language makes debugging easier and increases the readability and modularity of the code.