Calculator in JavaScript
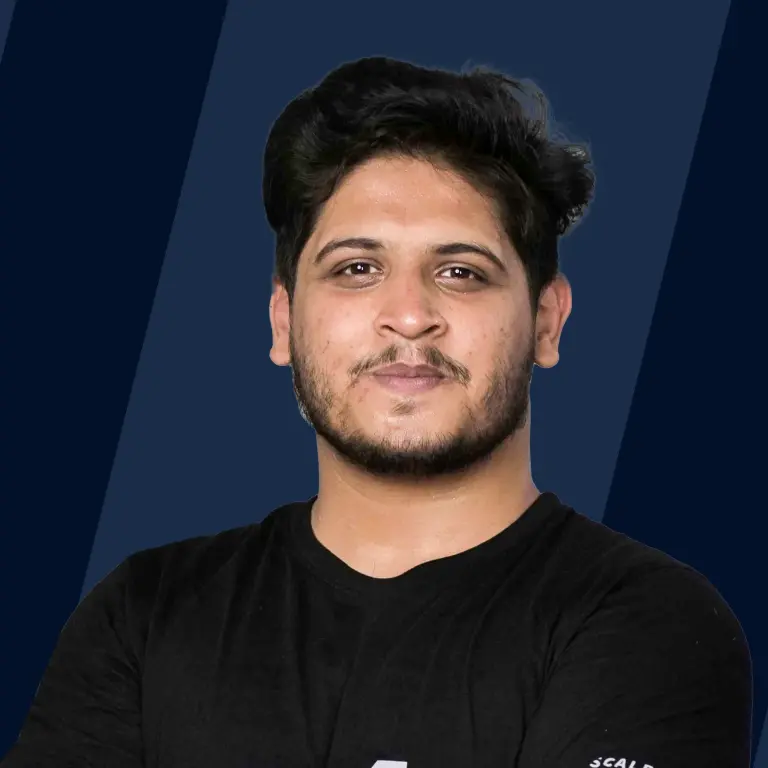
Creating a calculator in JavaScript is a fantastic way to learn and apply basic to advanced programming concepts. This project reinforces your understanding of JavaScript fundamentals, such as variables, functions, and event handling, and introduces you to more complex topics like DOM manipulation and UI design.
Approach to Create JavaScript Calculator
Building a calculator in javascript involves several key steps that combine fundamental programming concepts with practical application development. Here's a streamlined approach to get you started:
-
Begin by designing the calculator's interface. This can be completed using HTML and CSS. Create buttons for digits (0-9), operations (add, subtract, multiply, divide), and other functionalities like clear (C) or equals (=).
-
Implement the Calculator's Logic with JavaScript:
- Start by declaring variables to store the current input, the result, and the operation chosen by the user.
- Attach event listeners to the calculator buttons. The corresponding value or operation should be stored or executed, when a user clicks a button.
- Write functions to perform arithmetic operations. These functions will take input values, perform the selected operation, and return the result.
- Ensure that the input values and results are displayed to the user. This involves updating the text content of an element designated for displaying numbers and results on the calculator's interface.
-
Handle User Inputs: It's crucial to handle different types of user inputs correctly, such as:
- Allow users to enter numbers more than a single digit by concatenating digit inputs until an operation button is pressed.
- Implement logic to handle decimal points, ensuring users can perform operations with decimal numbers.
- Allow users to chain operations together, where the result of the first operation is automatically used as the first operand of the next operation.
-
Implement error handling to manage cases such as division by zero, invalid inputs, or syntax errors.
-
Once the basic functionality is in place, you can add additional features like keyboard support, advanced mathematical operations, and memory functions.
Create a Simple HTML, CSS, JavaScript Calculator
Below, you'll find simple JavaScript calculator code.
Output:
[IMAGE 1 {Add scaler topics logo into it} START SAMPLE]
[IMAGE 1 FINISH SAMPLE]
Create a JavaScript Calculator using If... Else...If statement
We can make a calculator in javascript with the help of if else statements without using HTML and CSS, by running it on the console in the Developer tools / Inspect element.
Let's see how this works:
Calculator made using if else statements:
Dynamic JavaScript Calculator using the HTML, CSS and JavaScript
This is the Dynamic Calculator created using HTML, CSS, and Javascript (all code at one place):
HTML
CSS
Javascript
The HTML is used for structuring the calculator, CSS is used for styling the calculator, and Javascript is used to add functionality to it.
Our resulting Simple calculator looks like this:
[IMAGE 2 {Add scaler topics logo into it} START SAMPLE]
[IMAGE 2 FINISH SAMPLE]
This calculator in javascript looks pretty and functional, but there are many spaces for improvement in it, like adding parenthesis, exponents, roots, logarithms, and other mathematical functions. The styling of the calculator should be in your hands only; let the creativity flow! Feel free to share your ideas with us.
Conclusion
- Creating calculator in JavaScript is a fantastic way to learn and apply basic to advanced programming concepts.
- We used CSS flexbox, CSS grid, and Vanilla Javascript to build our dynamic calculator.
- We also built Simple calculators using If else in Javascript.