What is Character Array in C?
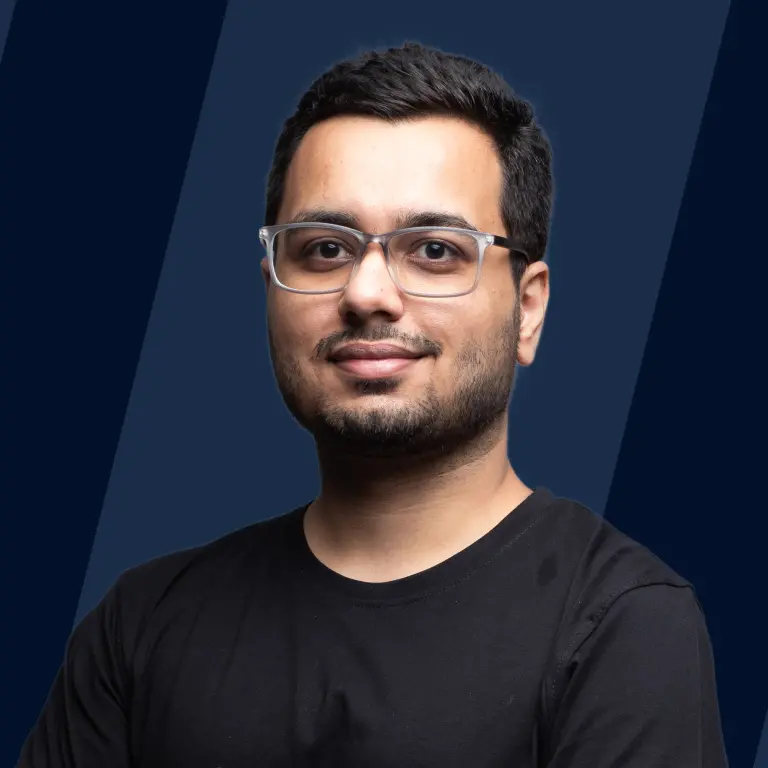
- A Character array is a derived data type in C that is used to store a collection of characters or strings.
- A char data type takes 1 byte of memory, so a character array has the memory of the number of elements in the array. (1* number_of_elements_in_array).
- Each character in a character array has an index that shows the position of the character in the string.
- The first character will be indexed 0 and the successive characters are indexed 1,2,3 etc...
- The null character \0 is used to find the end of characters in the array and is always stored in the index after the last character or in the last index.
Consider a string "character", it is indexed as the following image in a character array.
Syntax
There are many syntaxes for creating a character array in c. The most basic syntax is,
- The name depicts the name of the character array and size is the length of the character array.
- The size can be greater than the length of the string but can not be lesser. If it is lesser then the full string can't be stored and if it is greater the remaining spaces are just left unused.
Example
Before seeing the example, let us understand the method of dynamic memory allocation which is used to create the dynamic array.
- Dynamic memory allocation is an efficient way to allocate memory for a variable in c.
- The memory is allocated in the runtime after getting the number of characters from the user.
- It uses pointers, which are structures that point to an address where the real value of the variable is stored.
- The malloc() function is used for dynamic memory allocation. Its syntax is,
- The malloc function returns a void pointer which is cast (converted) to the required data type.
- The sizeof() function gives the size of data types and has the syntax,
- The malloc method is present in the header stdlib.h.
Now, let us see the example of how we can create a dynamic single-dimension character array by getting input from the user.
Output:
Anyone may find this line in code curious,
There is no & in scanf. This is because the variable arr is a pointer that points to an address and the & symbol is used to represent the address at which the variable is stored.
The space before %c is left intentionally and by doing this the scanf() function skips reading white spaces.
The arr+i is used to store the values in consecutive addresses with a difference of 4 bytes(size of a pointer) which is diagrammatically expressed below,
Initialization of Character Array in C
There are different ways to initialize a character array in c. Let us understand them with examples.
Using {} Curly Braces
- We can use {} brackets to initialize a character array by specifying the characters in the array.
- The advantage of using this method is we don't have to specify the length of the array as the compiler can find it for us.
Let us see a example where we initialize an array using {} and print it.
Output
The puts() function is used to print a character array or string to the output. It has the following syntax
The const char* is a character pointer that points to the character array. The puts function returns a positive integer on success and the EOF(End of file) error on failure.
The strlen() function is present in the string.h header and is used to find the length of a string. It has the following syntax,
This function returns the length of the string.
Using String Assignment
- A easy way to initialize a character array is using string assignment.
- We don't need to specify the length of the array in this method too.
Consider the following example,
Output
Using {{ }} Double Curly Braces(2D Char Array)
- This method is used to store two or more strings together in a single array.
- As a character array can only store characters, to store different strings we use a 2-dimensional array with syntax arr[i][j].
- The i denotes the string and j denote the position of the character in the string at position i.
- The i can be left empty as it is automatically computed by the compiler but the value of j must be provided.
Output
Examples of Character Array in C
Let us see more examples to better understand character arrays.
Convert Integers to Characters
- In computers, characters are represented as numbers according to the ASCII values.
- The ASCII value can represent numbers from 0-9, alphabets(both lower and upper case), and some special characters. The ASCII values are predefined for each letter or character.
- To convert an integer to a character we add the integer with 0, which has an ASCII value of 48. For example, If we want the letter A which has an ASCII value of 65, we just need to add 0 and 17.
- From the input number, we take the last digit and convert it to a character then store it, then follow the same for the rest of the digits by removing the digit as it is converted to a character and stored.
Output
Convert Multiple Arrays to Character Array
- We can concatenate multiple arrays to a single array using loops to iterate till \0 to find the last element of the first array.
- Then add the elements of the second array to the end of the first array.
- Then we mark the end of the array with \0.
- In this example we have used %s which is the data type for a string in c.
Output
Convert Strings to Character Array
- We can concat many strings to a character vector using the strcat() method in c.
- The syntax of strcat() method is ,
- This method concatenates two strings and stores the result in string1.
- This method is present in the header string.h.
- The strlen() method returns the length of the string and has the syntax,
- This method is also present in the string.h header.
Output
In this example, we are concating two arrays using the strcat function and printing the concatenated string.
Convert Duration Array to Character Array
- The scanf() not only reads input but also returns how many inputs are read.
- It has some special syntax to perform special functions such as the %n representing that only n numbers are read.
- The [^symbol] represents that the symbol is excluded when reading the input.
Output
We first read 2 digits for hours and 2 digits for minutes while excluding the character :. We store the values in character arrays h and m.
What is the Use of Char Array in C?
- A string is stored and represented using a character array.
- Every string operation such as copying two strings or concatenating two strings is done only using the character array.
- We can individually access every character in an array using the character array.
- Writing and reading from files also use character arrays.
Importance of Char Array
- Character array is used to store and manipulate characters.
- File systems and text editors use character arrays to manipulate strings.
- NoSQL(Not only SQL) databases that store data in JSON also use character arrays.
- Character array is used in language processing software and speech-to-text software.
- It is used in DNA matching and genome matching as pattern matching can be easily done using characters.
Program for Char Array in C
Display a given string through the use of char array in C Programming
- The gets() function can be used to get the string as input from the user.It receives input from the user until the Enter key is pressed. It has the following syntax,
The gets function returns the arr on successfully getting input from user or returns EOF(error on failure).
In this program, we are getting the string from the user and storing it in the array arr. Then we check if the array is empty by checking the first position of the array for \0. If the array is not empty, then we print the elements of the array.
Program in C to Calculate the Length and Size of a Given String
- The string length and size can be easily computed by the strlen() method and using the sizeof() operator.
- The size of the string is the same as the length of the string because the size of char is 1. The total size of the string is 1*length_of_string.
- The string length can also be calculated without using the strlen() method. We will look into both methods with the following example,
Output
We have created a for loop which will increment the value of i till the end of the string is reached. Finally, the value of i will be the length of the string.
Similarities & Differences between Character Array and Character Pointer in C
The differences between the character array and pointer are listed below,
- A character pointer is a pointer that stores the address of the character array. It represents the address of the character array.
- A character array is used to store the character and represent the values in the character array. The way of defining the character pointer and the array is,
- In character pointer the values at each index can be accessed by adding the pointer and the location at which the value is present.
- In the character array the values at each index can be accessed by the index.
Output
The * used here is called the dereferencing operator and is used to get the value from the address stored in ptr+2.
- The value of the character array can't be replaced as the old address can't be changed.
- The value of the address pointer can be changed as it can change its address and point to the new array.
- Dynamic memory allocation can't be used in the character array.
- Dynamic memory allocation can be used in character pointers.
The similarity between character array and pointer is,
- Both the character array and character pointer have a data type of char or char* which makes them similar to be handled by any function.
Difference Between Character Array and String
- Character array represents a series of characters that are indexed separately and stored consecutively in memory.
- String are characters written together, they can also be represented as a character array.
- A string should be assigned to a character array at the time of initialization or else an error will occur.
- The above example proves that strings are not the same as characters but both string and character array are interchangeable.
Conclusion
- Character arrays in C are used to store characters and represent strings.
- There are three ways to initialize a character array.
- Character array can be manipulated by using functions such as strlen() and strcat() etc..
- Character arrays are very useful and have important use cases.
- There are many similarities and differences between the character Array and the character Pointer in C.
- There are a few aspects in which a Character Array differs from a String.