JavaScript String charAt() Method
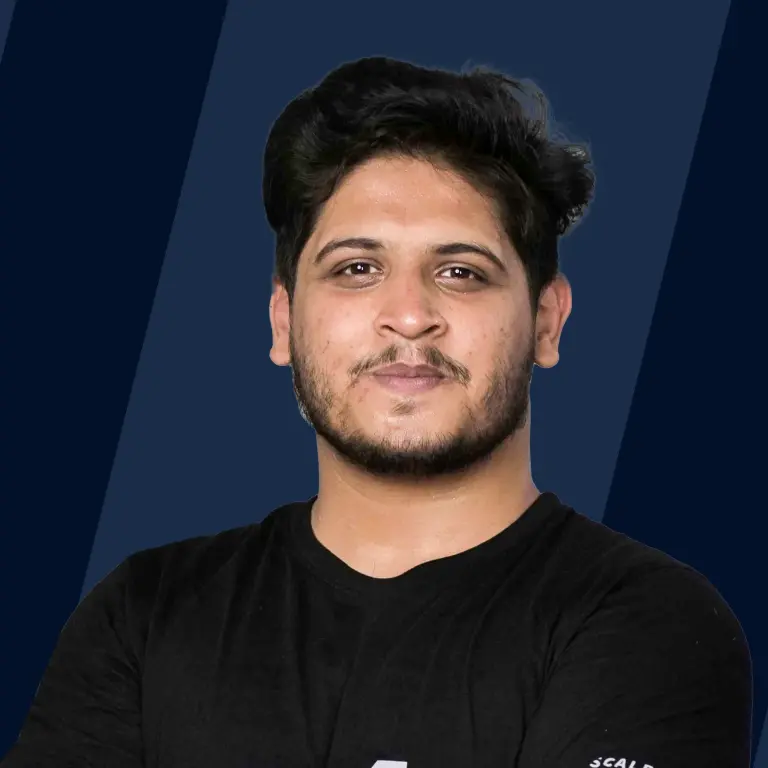
Overview
The charAt method of the String class in JavaScript is used to get the character at the specified index value of the String.
Syntax of String charAt() in JavaScript
The syntax of charAt in JavaScript is:
Just like other methods, we need to append this method with the desired String using a .(dot) as mentioned in the above Syntax.
Parameters of String charAt() in JavaScript
The charAt method has only one parameter:
- indexValue: It is the index of the desired character. It is of number data type.
Return Value of String charAt() in JavaScript
Return Type: string
The return value of charAt in JavaScript is a string that contains a single character.
Example
Getting the character present at the first index of the String.
Code:
Output:
What is charAt() in JavaScript?
While dealing with Strings in JavaScript, there can be situations when we want to get a specific character of the String. We can use the charAt method of Strings in JavaScript in such situations. This method accepts an index value and returns the character present at the passed index value.
In case the passed index is not there in the String (which is the case of index out of range), an empty string is returned. Also, if no index is passed in the charAt in JavaScript, the 0 index is automatically passed as it is assigned as the default index.
Important Points for the charAt method
- If the passed index is out of range of the String, an empty string is returned.
- If no index is passed, it returns the String's first character as the default index is 0.
- If a numeric string is passed, it is converted to its equivalent number.
- If a non-numeric string is passed, the default index is considered 0.
- If a boolean index is passed, then true is coerced to 1 and false to 0.
More Examples
Example 1: Getting the First Character of the String.
Code:
Output:
Explanation:
In this example, We used the charAt in JavaScript to get the first character present at the 0th index of the specified String. As the character 'H' is present at the 0th index position, we got 'H' in the Output.
Example 2: Getting the last Character of the String.
Code:
Output:
Explanation:
In this example, we used the charAt in JavaScript to get the character present at the last index of the specified String. We used the String.length property of the Strings to get the last index value, but as the indexing starts from zero, we subtracted one from the String length. As the character 'y' is present at the last index position, we got 'y' in the Output.
Example 3: Getting any Character of the String.
Code:
Output:
Explanation:
In this example, We used the charAt in JavaScript to get the character present at the 2nd index of the specified String. As the character 'v' is present at the 2nd index position, we got 'v' in the Output.
Example 4: Passing No Arguments to charAt Method
We should note that if we want the String's first character, it is not mandatory to pass the index value in charAt in JavaScript. By default, if no index value is passed in charAt in JavaScript, it returns the first character.
Code:
Output:
Explanation:
In this example, We used the charAt in JavaScript without passing any index value. You can see that we got the first character in the Output.
Example 5: Passing the Out of Range Index Value in charAt() Method
If we pass the index value in the charAt in JavaScript that does not exist in the specified String, it returns an empty string "".
Code:
Output:
Explanation:
In this example, We passed the index value that does not exist in the String, which is the value equal to its length. Then we added an if-else block to check if the variable myChar contains an empty string or not. As the passed index is out of range, an empty string got stored inside myChar, and as a result, we got "The string is empty" in the Output.
Example 6: Passing a Non-Integer as Index Value in charAt Method
There can be different cases depending upon the different types of non-integer values that we pass in the charAt method like
- a numeric string: '4'
- a non-numeric string: 'string'
- a boolean: true or false
- an array: [1, 2, 3]
- an object: {one: 1, two: 2}
Let us see each of these cases in detail.
Case 1: Passing a numeric string as an index value in the charAt method
Suppose the passed Non-integer index value in the charAt method is a numeric string. In that case, that numeric String is converted(type coercion) into its equivalent number data type, and it gets executed the same as it gets for number values.
Code:
Output:
Explanation:
We passed a numeric string index value '4' in this example. This value got converted(or type coerced) into its numeric equivalent, 4. Hence we got the character l, present at the 4th index of the String Google.
Case 2: Passing a non-numeric string as an index value in the charAt method
If the passed Non-integer index value in the charAt method is a non-numeric string, it returns the String's first character.
Code:
Output:
Explanation:
We passed a non-numeric string index value 'string' in this example. Hence, we got the first character 'G' of the String Google in the Output.
Case 3: Passing a boolean as an index value in the charAt method
If the passed Non-integer index value in charAt method is a boolean(true or false), true is coerced to 1 while false is coerced to 0. Hence if it's true, the character at the 1st index position is returned, and if it's false, the character at the 0th index position is returned.
Code:
Output:
Explanation:
In this example, we passed true in the charAt method, we got the character present at the 1st index position that is G, and when we passed false, we got the character present at the 0th index position that is o.
Case 4: Passing an array as an index value in the charAt method
If the passed Non-integer index value in the charAt method is an array, then also it returns the first character of the String.
Code:
Output:
Explanation:
We passed an array index value [1, 2, 3] in this example. Hence, we got the first character 'G' of the String "Google" in the Output.
Case 5: Passing an object as an index value in the charAt method
If the passed Non-integer index value in the charAt method is an object, then also it returns the first character of the String.
Code:
Output:
Explanation:
In this example, we passed an object index value {one: 1, two: 2}. Hence, we got the first character 'G' of the String "Google" in the Output.
Browser compatibility
The charAt method in javascript is supported by all the browsers such as Chrome, Edge, Safari, Opera, etc.
Conclusion
- The charAt in JavaScript is used to find the character at the specified index value of the String.
- The index value can be a number or a numeric string. For other data types, the first value of the String is returned, but for the boolean true, the second value is returned.
- The return value of charAt in JavaScript is a string having one character only.
- By default, if no index value is passed in charAt in JavaScript, it returns the first character.