Check If Key Exists in Dictionary Python
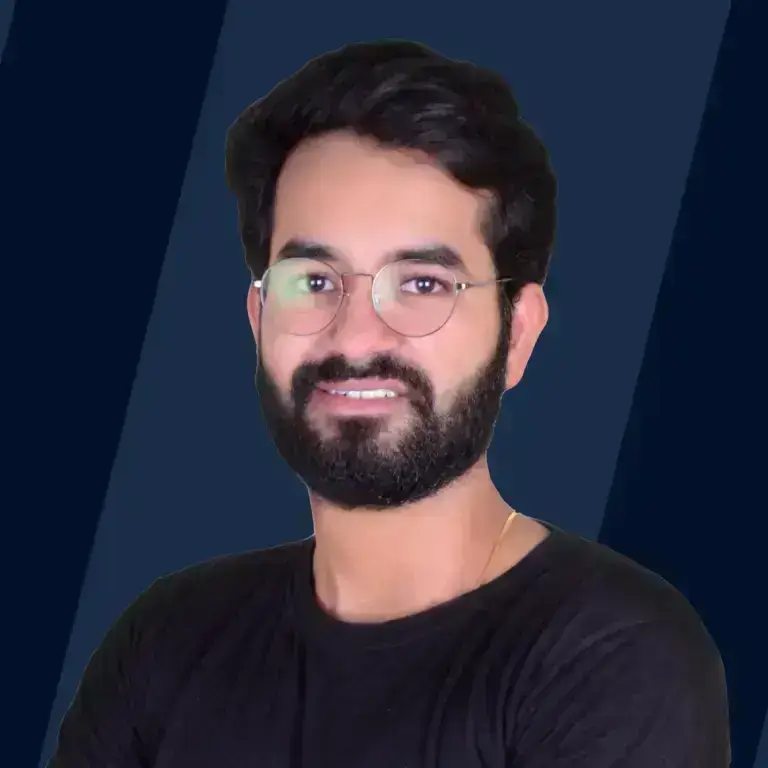
Overview
Dictionary in Python is a data structure that helps store key-value pairs. Before accessing the value associated with a key, we should check whether the key exists in the dictionary. Otherwise, unwanted errors may be encountered while executing our program.
Why Do We Check If a Key Exists in a Python Dictionary?
Suppose you have a dictionary in Python (that is essentially a collection of key-value pair mappings) that you use to store the names of the students in your class using their roll numbers as keys.
For example:
Now, imagine that the strength of the students in your class is very dynamic for the first month since some students drop the subject, and some take it. This is because of the relaxed course facility at your university. For attendance purposes, you want to ensure that the roll number you search for is in the dictionary. If you try to access a key in a dictionary that does not exist, your Python program will throw a KeyError exception. Therefore, checking whether the key exists before accessing it is always good. This prevents any unwanted behavior in your Python program.
Method 1 - Using if-in statement / in Operator
You can check if a key exists in a dictionary in Python using Python in keyword. This operator returns True if the key is present in the Python dictionary. Otherwise, it returns False.
Syntax
Code Example 1:
Output 1:
Explanation 1: Since 1602 was a valid key in dictionary dict, the in operator returned true. Since 1600 was absent in dict, 1600 in dict returned false.
Note: Since the return value of the in operator is a boolean, it can be used in conditional statements in Python. See the example below:
Code Example 2:
Output 2:
Explanation 2: Since 1602 in dict returned true, the if branch was executed by the program, alternatively, if the key had not existed in the given dictionary, the else part would have been executed.
Method 2 - Using get() Method
You can also use the get() method to check if key exists in the dictionary in Python. If the key exists, the get() method will return the associated value from the dictionary. Otherwise, it will return None (by default). You may specify a custom value to be returned (in case the key is not found) as an optional second parameter to the get() method.
Syntax:
Please see the examples below to understand the syntax usage better.
Code Example 1:
Output:
Explanation: Since the key 1601 exists in the dictionary dict, the retVal is not None, and instead is equal to the value mapped to 1601, that is, Aman.
Let us see another code example where we use the second parameter to check if the key exists in dictionary in Python.
Code Example 2:
Output:
Explanation: Since the key 1600 does not exist, the value returned by the get() method equals the keyNotFound variable, which we passed as the second parameter to the get() method.
Method 3 - Using keys() method
You can check if a key exists in dictionary in Python using the keys() method of the dictionary class. The keys() method returns a view object of keys (something similar to a collection) in which you can search the required key using the in operator we saw earlier.
Syntax:
See the code example below for a better understanding of how to use the keys() method to check if a key exists in the dictionary in Python.
Code Example 1:
Output:
Explanation: Since 1602 exists in the dictionary, it will also be in the returned view allKeys. Therefore, 1602 in allKeys evaluates to True, and the if branch gets executed. The else branch would have been executed if the key had not been present.
Method 4 - Using [] Operator With try-except Blocks
You can check if a key exists in the dictionary in Python using [] (index access operator) along with the try-except block.
If you try to access a key that does not exist in Python dictionary using the [] operator, the program will raise a KeyError exception. But we can manage that situation using the try-except block.
Syntax:
See the code examples below to check if key exists in dictionary in Python using the [] operator.
Code Example 1:
Output:
Explanation: Since the key we try to access using the [] operator, 1601, exists in the dictionary, the program throws no exception. See the example below for a case when the key does not exist.
Code Example 2:
Output:
Explanation: In this program, we try to access a key that does not exist in the dictionary. Therefore, the program throws a KeyError exception that is caught by the except block.
Method 5 - Using setdefault() method
You can check if a key exists in dictionary in Python using setdefault() method of dictionary class.
The setdefault() method returns the value associated with the key searched if the key exists. If the key does not exist, it will insert the key in the dictionary with a provided value (which, by default, is None) and returns that value.
Syntax:
Code Example 1:
Output:
Explanation:
- Since the key 1601 is present in the dictionary, setdefault() returns the associated value, that is "Aman".
- Since the key 1600 does not exist, setdefault() inserts the key into the dictionary with the default value None and returns it too!
- Since the key 1600 is now present in the dictionary (because of point 2 above), the setdefault() returns the associated value, None.
- Since the key 1605 is not present in the dictionary, the setdefault() method inserts it into the dictionary with the specified value, "Studnet Not Found!" and returns the value too.
Don't miss the opportunity to become a certified Python specialist. Enroll in our Python certification course and level up your career.
Performance Comparison
- [] and in operators are the fastest and most efficient way to check if a key exists in dictionary in Python. [] operator, of course, needs to be associated with the try-except block.
- get() and setdefault() methods lag behind the [] and in operators, since they also perform attribute/value lookup (along with insertion in case of setdefault()), while the other 2 only perform membership check.
- Using the keys() method is the least efficient method to check if key exists in dictionary in Python, since it involves returning the key-set as well as checking whether the key exists in that set. This entire operation is linear in time, while the ones discussed above are constant in time.
Conclusion
- Dictionary in Python is a collection of key-value pair mappings.
- in operator can be used to check if key exists in dictionary in Python or not. If the key exists, in operator returns true and false otherwise.
- Index operator ([]) can be used to access a dictionary key. If the key exists, it returns the associated value. If the key does not exist, it raises a KeyError exception. Therefore, it should be surrounded by try-except blocks to handle the exception.
- get() and setdefault() methods return the associated value when queried for a key that exists in the dictionary. If the key does not exist, these methods return a specified value, which by default, equals None. The setdefault() method, in addition, also inserts the key in the dictionary.
- The keys() method also helps us check for key membership in Python dictionary, but it is highly inefficient for a key-value pair lookup and should be avoided as such.