Checked and Unchecked Exception in Java
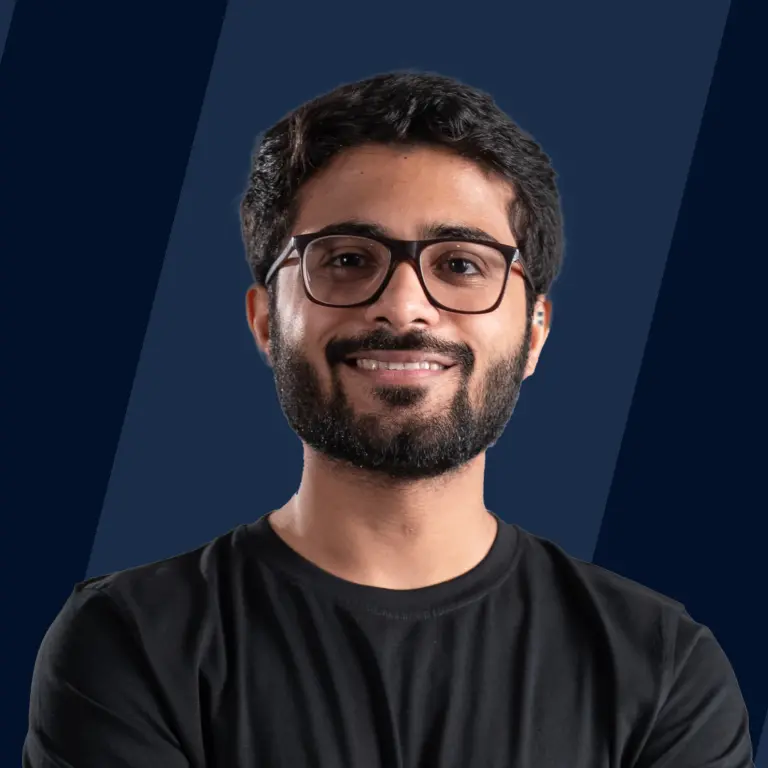
Overview
An exception is a disruptive occurrence that takes place at run time, or during the execution of a program, which interrupts the regular flow of the program's instructions. There are two categories of exceptions in Java:
- Checked exceptions
- Unchecked exceptions
Certain Java exceptions need to be handled in the code of the developer. Without using any exception handling semantics, above exceptions may occur.
Prerequisites
What are Checked Exceptions in Java?
In Java, Checked Exceptions are exceptions that are checked by the compiler during the compilation of the program. An example of a Checked Exception is the FileNotFoundException, which occurs during file handling in a Java program when the path of the file is invalid. This is a common cause of FileNotFoundException during compilation of a Java program.
Java Compiler is very smart :). It knows all the details of the checked exception. For example, it knows when and how checked exceptions can occur in the java program. It checks wherein the program checked Exceptions can occur and when they occur it provides ways to handle them.
When the FileNotFoundException occurs during the compilation of the java program. Compiler suggests that the exception must be caught or declared to be thrown to the caller method so the caller method can handle it. Even the different IDEs suggest these ways when there is the possibility of occurence of checked exceptions in the java program.
Types of checked Exception
There are various types of the checked exception, let’s see a few of them:
IO Exception
IO Exception generally occurs during the read and write operations on a particular file. We have already seen an IO Exception at the starting of an article i.e FileNotFoundException is an IO Exception that occurs due to the invalid path of the file.
SQL Exception
SQL Exception is also a type of checked exception which occurs both in the driver and the database. It provides information of database access errors and other database connection errors. Consider below exception:
In the above program, we are creating a connection between the MySQL database and the java application. If we give anything wrong information like the wrong database password or wrong host, then there will be a database connection error and this will cause SQLException.
ParseException
The ParseException is also a checked exception. This exception occurs when we fail to parse a string for a specific format of type. This type of exception is generally seen during the formatting of the specific data. Consider the example given below.
Output:
When we try to compile the above code, the java compiler will throw a ParseException which occurs during the compilation time of the java program. The reason behind this is that we used the wrong format of date which causes an error and throws parse Exception.
What are Unchecked Exceptions in Java?
Runtime Exceptions are known as unchecked exceptions. These are exceptions that occur during the runtime of the java program. These exceptions are not checked at compile time which means even if your program is giving an unchecked exception and even if you are not handling it, the program will not give a compilation error. These exceptions occur mainly from the programmer’s side during the run time of the program.
Let’s take a real-time example to understand the unchecked exception in java:
- A real-life example of an unchecked exception in Java is when you try to divide a number by zero. This will result in an ArithmeticException at runtime, which is an unchecked exception.
- For example, imagine you are writing a program to calculate the average of some numbers, and you accidentally divide by zero because you forgot to check for zero values.
- The program will compile without any errors, but when you run it, it will throw an ArithmeticException because dividing by zero is not allowed.
This is an example of an unchecked exception because it occurs at runtime and is not checked by the Java compiler.
Now comes the point of the programming language. Java compiler compiled the valid instructions which are totally valid but to the wrong instruction from the programmer, side Exceptions occur which cannot be handled during the runtime of the program and they are known as Unchecked Exceptions in java.
Types of Unchecked Exception
There are various types of the unchecked exceptions, let’s see a few of them:
ArrayIndexOutOfBoundsException
ArrayIndexOutOfBoundsException is an unchecked exception in Java that occurs when an attempt is made to access an array element at an invalid index. The index can be either negative or greater than or equal to the size of the array. For example, if an array has 5 elements, trying to access the 6th element will result in an ArrayIndexOutOfBoundsException.
Let’s try to understand ArrayIndexOutOfBoundException with the help of an example:
Output:
Explanation:
- The program initializes an integer array of size 4 and assigns a value to its first element.
- It then tries to assign a value to an index outside the range of the array, which causes an ArrayIndexOutOfBoundsException at runtime.
- The exception is not caught or handled in the program, so the program terminates with an error message.
InputMismatchException
InputMismatchException is a type of unchecked exception in Java, which is thrown when a program tries to read data of a different type than what is expected. For example, if the program expects an integer input but the user enters a string, this exception can be thrown. This exception is commonly encountered when using the Scanner class to read input from the user.
Let’s understand this exception using an example:
Output:
Explanation:
In the above program, the compiler was expecting input value as an integer, but at runtime, we gave a String as input that causes the JVM to throw InputMismatchException.
Now let’s see the differences between Checked and Unchecked Exceptions in Java.
Difference between Checked and Unchecked Exceptions in Java
Basis of Comparison | Checked Exceptions | Unchecked Exceptions |
---|---|---|
Compilation | These Exceptions occur during the compilation time of the java programs. | These Exceptions occur at runtime of the java programs. |
Compiler Checking | Checked Exceptions are checked by the java compiler. | Unchecked Exceptions are not checked by the java compiler. |
Exceptions Handling | These Exceptions can be handled during the compilation time. | These Exceptions cannot be handled at compilation time. |
Examples: | IOException FileNotFoundException InterruptedException | ArithmeticException InputMismatchException NullPointerException |
Conclusion
- Java has two types of exceptions: Checked Exceptions and Unchecked Exceptions.
- Checked Exceptions are exceptions that must be declared in a method or caught with a try-catch block. They are checked by the compiler at compile time.
- Unchecked Exceptions, on the other hand, are not required to be declared or caught. They are checked by the Java Virtual Machine at runtime.
- Some common examples of Checked Exceptions include IOException, ParseException, and ClassNotFoundException, while examples of Unchecked Exceptions include NullPointerException, ArrayIndexOutOfBoundsException, and IllegalArgumentException.
- Properly handling exceptions in Java is important to ensure that your program runs smoothly and doesn't crash unexpectedly.