Java Class loaders
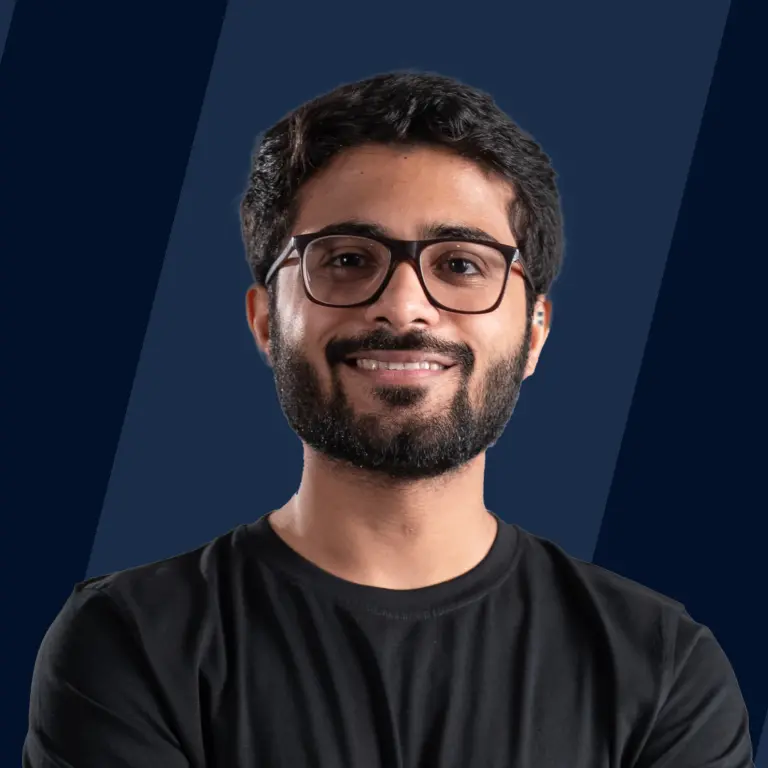
Overview
The Java class loader is an abstract class, belonging to java.lang package. It is a part of the Java run time environment that loads Java classes dynamically into the java virtual machine. It is used to load a class during run time. The java class loader is based on 3 principles, Delegation, Visibility, and Uniqueness. In this article, we will be diving deep into understanding class loaders in Java.
What is ClassLoader in Java?
Java class loader is an abstract class that belongs to java.lang package. It loads classes from the different resources at run time. The JVM (Java virtual machine) is used for performing the linking process at run time. The classes are dependent on other loaded classes and those are loaded into JVM according to the need. When we request a class to load, it delegates it to the parent class. By doing this, the uniqueness is maintained in the run time environment which is essential to execute a java program.
Types of the class loader in java In Java, there are three types of class loaders:
- Bootstrap class loader
- Extension class loader
- System class loader
- Bootstrap ClassLoader: It is a machine code that starts the operation when called by JVM. The bootstrap class loader is not a Java class, instead, its job is to only load a class from the location rt.jar. It doesn’t have any parent class loader and is also known as a Primordial class loader. It is a parent of all the class loaders. For example: When a String.class.getClassLoader() is called, it returns the output as null, and any code that uses it throws NullPointerException.
- Extension ClassLoader: It is a child of BootStrap ClassLoader. It is used for loading the extension of core classes of Java by using the respective extension library of JDK. This class loads the library from the directory, JRE/lib/ext or any other directory pointed java.ext.dirs by a system property.
- System ClassLoader: The application of ClassLoader is also known as System ClassLoader. It is used for loading application-type classes which can be found in the environment variable CLASSPATH, -classpath, or -cp command-line option. The ClassLoader Application is a child of Extension CLassLoader.
How Do Java ClassLoaders Work?
When JVM sends the request for a class, a loadClass() method of java.lang.ClassLoader is invoked internally and passes the name of the class. The loadClass() method then calls the findLoadedClass() method to check whether the class has been loaded successfully or not. This is helpful to avoid loading of class multiple times. If the class is loaded already, then it delegates the request to the parent ClassLoader of the class. In case, if the ClassLoader is unable to find the class then findClass() is invoked which looks for classes in the file system. The diagram shows how the class is loaded using ClassLoader. To understand this more efficiently, let’s take an example.
Compile and run the code given using the following command:
Note -verbose:class: is used for displaying the information about the classes that is been loaded by JVM.
In the example given, we have a class called Exp. class. The request of loading this class file is sent to the application class loader. It then delegates it to the extension class loader and then to the bootstrap class loader. Bootstrap class loader will first check it in rt.jar and if the class is not present in it, it will transfer the request to the extension class loader which searches for it in the directory jre/lib/ext. If the class is found in that directory then it loads the class. If it does not found in the given directory then the application class loader is used to find and load it from CLASSPATH in Java. To sum it up in the points:
- It will first check if the class is already loaded or not
- If not, then it will ask the parent class loader to load the class.
- If the parent class loader is unable to load the class then it tries to load it in the class loader.
Three Important Features of Class Loaders in Java
These are a set of rules on which the ClassLoader in Java works. The three functionality are:
- Delegation Model
- The JVM (java virtual machine) and the ClassLoader of java use the Delegation Hierarchy Algorithm to load the class into the java file. The working of ClassLoader is based on a set of operations as given by the delegation model. The following are the operations performed:
- Whenever the JVM comes across a class, it first checks whether the class is loaded or not.
- If it's already loaded, then the JVM proceeds with the execution of the code in the method area or heap.
- If in case a class is not present in the method area then ask the java ClassLoader Sub System to load that class after which it hands over the control to the application class loader.
- Application ClassLoader delegates the request to Extension ClassLoader which in turn delegates the request to Bootstrap ClassLoader.
- Bootstrap class loader will search it in classpath(JDK/JRE/LIB). If the class is found then loaded else it is delegated to Extension ClassLoader.
- Extension ClassLoader search for the class in Classpath(JDK/JRE/LIB/EXT), if found then loaded else it transfers it to the Application ClassLoader.
- The application class loader then searches for the class in Classpath, if found then loaded else ClassNotFoundException is generated at the output.
- Unique Classes- It ensures that the class is unique and there are no duplicate classes. It also ensures that the class is loaded only by its parent class loader and not the child class loader. If in case, the current parent class loader is unable to find the class then-current instance would attempt to do it.
- Visibility- This principle states that a class, loaded by the parent ClassLoader is visible only to the child ClassLoader and not to the classes that are loaded by the child ClassLoader. To understand this, let's take an example: Let’s say a class called Exp. class is loaded by Extension ClassLoader. The class Exp. class is then visible to only Extension and Application ClassLoader and not by the Bootstrap ClassLoader. If Exp. the class tries to load by using Bootstrap class loader then an exception java. lang.ClassNotFoundException is generated at the output.
Class Loading
When Classes are Loaded?
There are only two cases when the class is loaded:
- When the new byte code is executed.
- When static reference is made to a class by byte code, for instance, System. out
Static vs. Dynamic Class Loading
Static loading is the loading of a class statistically with a “new” operator whereas dynamic class loading invokes the class loader function at run time by using the method Class.forName().
Static Class Loading | Dynamic Class Loading |
---|---|
Classes are loaded using the "new" operator | programmatically invoke a function of the class loader at run time. |
Car car= new Car() | Class.forName(String className) |
No ClassDefFoundException is thrown if the classes are loaded statically but class is not found at run time. | ClassNotFoundException is thrown in dynamically loaded method. |
Methods in Loading a Class in Java
To load a class, a few steps are followed. The classes are loaded as per the delegation model.
- loadClass(String name, boolean resolve)- This method is used for loading a class that is referenced by JVM. It takes the parameter as the name of the class of type loadClass(String, boolean).
- defineClass()- This method cannot be overridden as it is final. It is used for defining an array of bytes as an instance of a class. The error ClassFormatError is thrown if it is invalid.
- findClass(String name)- This method is used for finding a specified class. It is only used for finding a class and not for loading it.
- findLoadedClass(String name)- This method is used for verifying whether the previous class referenced by JVM was loaded or not.
- Class.forName(String name, boolean initialize, ClassLoader loader)- This method is used for loading and initializing the class. It gives the option to choose any one ClassLoader. If the parameter of ClassLoader is null, the Bootstrap ClassLoader is used.
Building a SimpleClassLoader
To build a simple class loader, a file needs to be loaded using a custom class loader. For that, we need to override the findClass() method.
In this example, we have to define a simple class loader that extends the default class loader and loads an array of bytes from the specified array. This is how you create a custom class loader.
Example of Class Loader in Java
Before any class is loaded, this code snippet is executed. If the class is loaded already then it returns it. Else, it delegates the search for finding a new class to the parent class loader. If the class is not found by the parent class loader then loadClass() calls the method findClass() to find and load the class respectively. The method findClass() searches for class in the current ClassLoader if not found in the parent class.
Example:
Output:
Class loader of this class:sun.misc.Launcher$AppClassLoader@18b4aac2
Class loader of Logging:sun.misc.Launcher$ExtClassLoader@3caeaf62
Class loader of ArrayList:null
From the given example, we can see that there are three different class loaders here: application, extension, and bootstrap The application class loader loads the class where the method is contained. An application or system class loader loads files in the classpath. The extension class loader is used for loading the Logging class. Extension class loaders load classes that are an extension of the standard core Java classes. The bootstrap class loader loads the class that contains ArrayList. A bootstrap or primordial class loader is the parent of all the others.
Where to use ClassLoader in Java?
The ClassLoader is used for abstract classes. It is a part of the Java run time environment that loads Java classes dynamically into the java virtual machine. It is used to load a class during run time. It is responsible for loading java classes dynamically at run time to JVM. The JVM doesn’t need to know about the underlying file system to run java programs, all this is possible only because of ClassLoader.
Conclusion
- The Java class loader is an abstract class, belonging to java.lang package. It is a part of the Java run time environment that loads Java classes dynamically into the java virtual machine
- Types of the class loader in java
- Bootstrap Class Loader
- Extension Class Loader
- System Class Loader
- Three important features of class loaders in Java
- Delegation Model
- Unique Classes
- Visibility
- Methods for loading a Class in Java
- loadClass(String name, boolean resolve)
- defineClass()
- findClass(String name)
- findLoadedClass(String name)
- Class.forName(String name, boolean initialize, ClassLoader loader).
- Static loading is the loading of a class statistically with a “new” operator whereas dynamic class loading invokes the class loader function at run time by using the method Class.forName().