Java String compareTo() Method
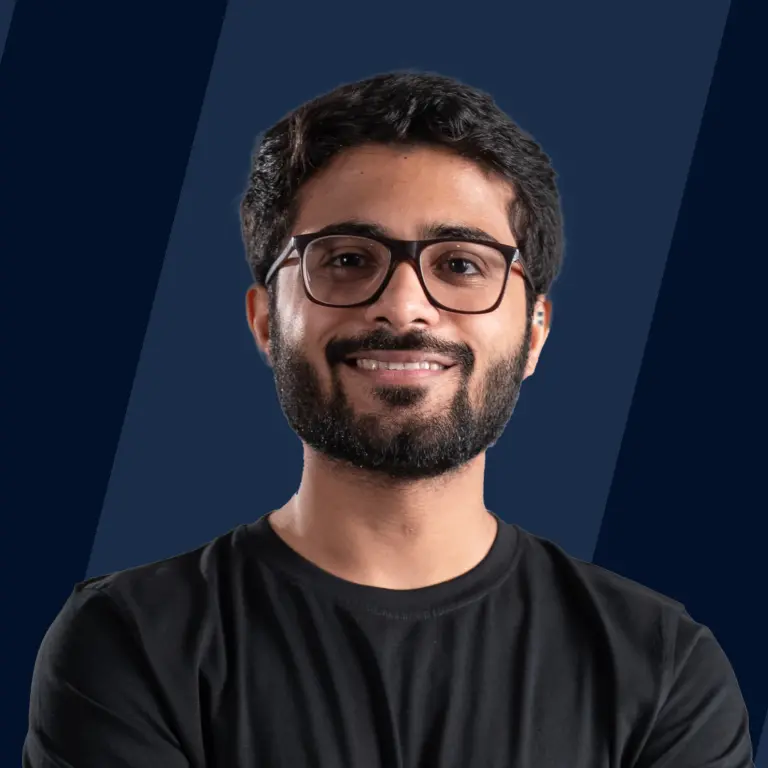
Overview
Java has an interface called Comparable, which contains the compareTo() method. Any class implementing the Comparable interface must override the compareTo() method.
Java.lang.String class which implements the Comparable interface defines the implementation of compareTo() method. This method is used for the lexicographical comparison of two strings. It compares two Strings A and B and returns:
- A negative number if A is less than B.
- A positive number of A is greater than B.
- Zero if both strings are equal.
Introduction to compareTo Method in Java
Syntax of compareTo()
compareTo() method of the String class in Java is called using an Object and takes another Object of the same type as an argument. It returns a positive integer, negative integer, or zero. It has different implementations for different classes. The signature for the method which accepts a parameter of type String and compares it with the current string is given below:
And it's syntax will be:
We will discuss other implementations of compareTo() later in this article.
compareTo() Parameters
compareTo() in Java takes only a single Object as a parameter which is used for comparison.
Note:
obj1 and obj2 must belong to the same class, and their class must implement the Comparable interface.
compareTo() Return Values
Return Type: Integer
compareTo() in java returns an integer value. It returns a positive integer if string1 is lexicographically greater than string2, negative if string2 is greater than string1, and zero if both are equal.
Exceptions
The compareTo() method in Java throws two Exceptions. These two exceptions are ClassCastException and NullPointerException.
ClassCastException
If we try to compare two Objects which are of different data types, then ClassCastException is thrown.
For example: we try to compare String and ArrayList, so we get ClassCastException.
Let us take an example where we try to compare two objects of different data types.
Explanation:
- In the above example, we are comparing objects of StringBuffer and String classes using compareTo() method. compareTo() method throws ClassCastException in such a case.
- But we can convert StringBuffer to String using the toString() method. After the conversion, we can compare two strings without any exceptions.
NullPointerException
compareTo() method in java throws NullPointerException if we try to compare an object with another null object.
Look at the example below, we are initializing one of the String as null, and we try to compare two strings.
Output:
Explanation:
When any of the strings under comparison is null, NullPointerException is thrown.
Example
Output:
As we know character comes after in the dictionary, it returns a positive number. Thus, we get as output.
Java String CompareTo() Case Sensitivity
Case sensitive is where uppercase characters and lowercase characters are different. For example, 'a' and 'A' are not the same in the case-sensitive scenario. compareTo() method uses the Unicode of each character in both the strings for comparison. Also, the Unicode of lowercase and uppercase characters are different. Hence, compareTo() method is case sensitive.
Example:
Output:
Explanation:
- str2 is equal to str1 except that str2 is in lowercase. When we compare them we get output as -32.
- Unicode of J is 74 and j is 106. Thus, the difference of 74-106 (J-j) is -32 and so is the output.
Note:
- If string1 > string2, it returns positive number.
- If string1 < string2, it returns negative number.
- If string1 == string2, it returns 0.
Three variants of compareTo() method in Java
int compareTo(Object anotherObj)
All the classes that implement the Comparable interface must override the compareTo() method as it is a abstract method of the interface. The compareTo() method in Java takes an object of this class as a parameter, and we can define this method to compare two objects according to the sorting order.
Let us define a class Book that implements Comparable interface, stores details like the name of the book, author, etc. and overrides the compareTo() method.
- We compare two books by their publishing year.
- If a book is published after another book, it should return 1.
- If the book is released before another book it should return -1.
- If both books are released in the same year, it should return 0.
Output:
Explanation:
- The above class Book, implements the Comparable interface and overrides the compareTo() method.
- Book has three parameters, book_name, author, and year (year of book publication).
- Constructor method takes these three parameters to create an instance of Book class.
- In the main method, we create three Book objects namely, Java, Kotlin, and Python and we specify its parameters.
- Now we try to compare two Book objects, Java and Python. As we know Java's publication year(2001) is prior to Python's (2005), it returns -1.
- Similarly, since the publication year of Kotlin book is greater than Python's, 1 is returned as output.
int compareTo(String anotherString)
String class in Java defines the compareTo() method to compare two strings lexicographically i.e. in dictionary order. If a string1 comes before string2 it returns a positive number, if string1 comes after string2 it returns a negative number, and if both the strings are equal, it returns 0.
int compareToIgnoreCase(String str)
compareToIgnoreCase() method is the same as the compareTo method; it compares strings irrespective of the uppercase or lowercase, i.e., treats small letters and capital letters equally. This method is case insensitive, unlike the compareTo method.
Consider an example of two same strings with different cases.
Output:
Explanation:
Unicode of H is 74 and h is 106 so compareTo() method returns its difference i.e. -32 whereas compareToIgnoreCase() method returns 0 as h and H as equal for it.
How to find length of a string using String compareTo() method
The length of a string can be found out by comparing that string with an empty string.
Let us take an example to understand this.
Output:
Explanation:
When we compare the non-empty string with the empty one, we get a positive number that is the length of the non-empty string.
Thus, using the compareTo() in Java we can find the length of the string by comparing with an empty string as it returns the length of the non-empty string with positive and negative signs as per the position of the non-empty string.
Important Examples of CompareTo in Java
Example 1: Java String compareTo()
Output:
Example 2: empty string
An empty string and null are not equivalent.
- An empty string doesn't throw an exception and points to a string with length 0 or no characters, whereas
- null string points to nothing (garbage value) and throws an exception. So, the compareTo method also must have a different output for null and empty strings.
Let us take an example to understand what happens when we compare an empty string with another non-empty string. We will take three strings, out of which one will be empty and the rest of the two with different lengths.
Output:
Explanation:
In the example above, when we compare an empty string with a non-empty string it returns a negative number which is nothing but the length of the non-empty string.
Vice-versa, when we compare the non-empty string with the empty one, we get a positive number that is again the length of the non-empty string.
Thus, using the compareTo() in Java we can find the length of the string by comparing with an empty string as it returns the length of the non-empty string with positive and negative signs as per the position of the non-empty string.
Example 3: Check if Two Strings are Equal
If two strings are equal, the compareTo() method returns 0. We can use this to check if two strings are equal. Let us take an example of a login form. If the username and password match, we say login successful.
Output:
Explanation:
- In the login form, we ask the user for username, comparing user-entered username and username string using the compareTo method, if they are equal it returns 0 and the condition becomes true.
- Then we ask for the password. Similarly if the password matches, we print the message of a successful login. Else, we print the message Incorrect Username or Password.
Conclusion
- Java.lang.String class contains** compareTo()** method. It is used to compare two strings lexicographically. It returns the difference of Unicode of the first unmatched character. It is a case sensitive method.
- In Java, any class implementing Comparable interface must override the compareTo() method and define a way to compare two objects of the class.
- compareTo() method in java throws two exceptions, namely NullPointerException and ClassCastException.
- When we try to compare the null object with any other object NullPointerException is thrown, and when we try to compare objects of two different data types, ClassCastException is thrown.
- compareTo() method of String class has many uses. It can be used to find the length of any string by comparing it with an empty String. It can be used to check if two Strings are equal. Additionally, it is always used to compare two strings.
- Java has another method called compareToIgnoreCase() method. This is just like the compareTo() method, the only difference is it treats uppercase and lowercase characters equally.
- Built-in sorting methods use compareTo() in java for sorting its objects.