What is Compile-Time Polymorphism in Java?
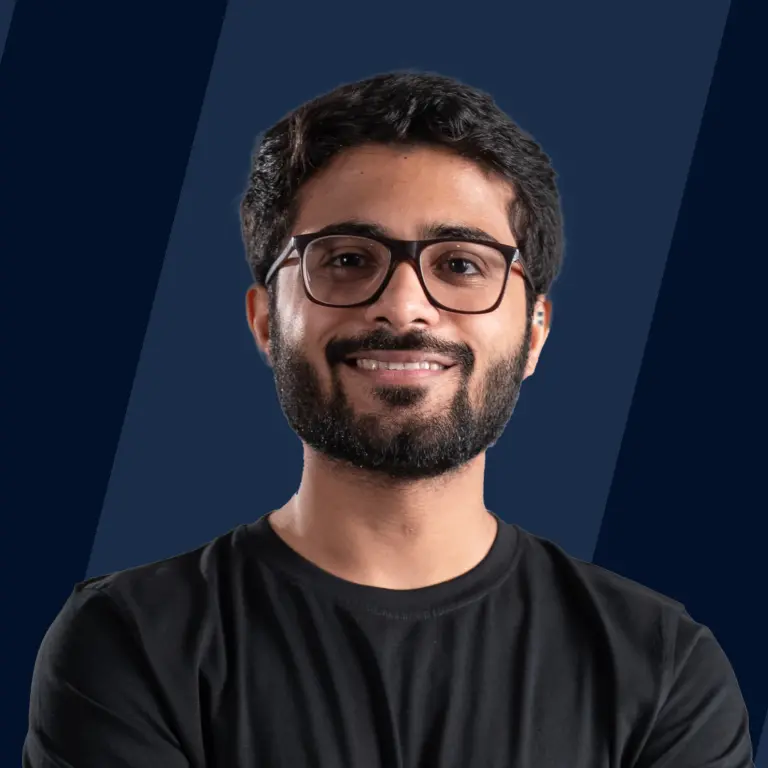
The term polymorphism comes from two Greek words, poly means many and morphs means forms. It allows us to perform various operations by using the same method.
Polymorphism is of two types: Static also known as Compile time polymorphism and Dynamic also known as Runtime polymorphism
Compile time polymorphism in Java is also known as early binding or static polymorphism. The binding is performed during compilation time and hence the name compile-time polymorphism. Binding refers to connecting the function call to the function body. It is achieved by overloading methods, operator overloading, and by changing the signature of the function. Overloading of methods is done by the reference variable of the class. Operator overloading is done by extending the functionality of an operator with different functionalities. The term signature of function refers to parameters and their types along with the return value and its type. It defines the input and output of a function.
Note: Reference variable is used to point objects/values in a given class or a function.
Example:
In this, the methods are overloaded by changing the number of parameters in the argument. The first add method includes two parameters (a and b) and it returns the addition of two numbers given in the argument whereas the second method of add includes three parameters (a, b, and c) and it returns the addition of three numbers a,b, and c given as parameters in the argument.
To sum it up:
- Overloading of main method (add) in the example is done by changing the parameters
- The first method takes two parameters and returns the addition of two number
- In the second method the addition of three numbers is returned
- In the main class, an object for reference is created
- This object is used to call add method of the SimpleCalculator class
- If the user calls the add method and passes two parameters in the argument then the first add method is called.
- If the parameters passed is 3 then the second add method is invoked.
Advantages of Compile-time Polymorphism in Java
There are various advantages of compile-time polymorphism in Java. The following are the key advantage of compile-time polymorphism:
- Debugging the code becomes easier
- It helps us to reuse the code.
- Binding of the code is performed during compilation time
- Overloading of the method makes the working of the code simpler and more efficient.
Note: Binding refers to connecting the function call to the function body.
Method overloading In Java, we can declare two or more methods, with the same function name in the class, provided their parameter declarations vary.
The Java compiler observes the signature of the methods (i.e method name, number of parameters, and type of parameters) and is able to differentiate amongst the methods of the same names by the difference in their method signatures.
The difference can be:
- In the number of parameters
- Sequence of the order of parameters
- Data types of parameters
The difference in method signatures helps the Java compiler to bind the appropriate method. Rules for Method Overloading:
- We Must change the argument list (i.e number of parameter types, sequence).
- We Can change the return type.
- We Can change the access modifier.
- We Can overload a method in the same class or subclass.
Syntax:
Here, the method overloaded is func(). The methods have the same name but the arguments are different.
There are different ways in which methods can be overloaded. The following are the types of overloading a method:
1. Overloading by changing the number of parameters in the argument
Output:
In the above example, it is seen that for displaying one argument, display(int a) method is called while for displaying two arguments, display(int a, int b) method is being called internally. The name of the method here is the same which is displayed but the argument list or the parameters are kept different.
2. By changing Datatype of parameter
Output
In this example, the data type of the parameter is changed while the method name and number of parameters are kept the same. For displaying an Int data, display(int a) method is called while in order to display String data, display(String a) method is invoked internally.
3. By changing the sequence of parameters
Output:
In the above example, the Student identity are displayed. If the parameters passed by the user is (name, id) then Method 1 is called else if the parameters are passed in reverse order i.e (id, name) Method 2 is invoked internally.
Operator Overloading
Operator overloading is the ability of an operator to redefine its functionality. It refers to using the same operator on different data types.
For example, the addition of two integers (1+3)=4 gives output as 4 whereas "+" operator when used with strings gives output as a concatenation of two strings ("a"+"b")= "ab".
Programming languages like C++ support operator overloading but in Java, operator overloading is not supported.
Operator overloading is functions with special names which is the name of the operator to be overloaded. Just like any other function, an overloaded operator has a list of parameters as well as the return type.
Below is the table which shows the type of object and its return type:
object | return type |
---|---|
String+String | String |
Int+Int | Int |
String+Int | String |
But the question arises as to why doesn’t Java support operator overloading.
Well, to understand this, here are the following reasons that justify why is operator overloading not supported in Java.
- Operator overloading make the code complex- In this case, the compiler, as well as the interpreter of Java which is JVM, needs to put extra effort in order to find the functionality of the operator used in the statement.
- Error in programming- This is because the custom definition for operators creates confusion for programmers which increases the rate of error.
- Easy to develop IDE tools- Without operator overloading, it is simple to develop and integrate IDEs and environments in Java. However, operator overloading can be achieved in Java with the help of method overloading in a clear and error-free manner. For instance, operator overloading is used for contention of string type in Java. Note that you cannot define your own operator overloads.
Example: String concat = "one" + "two";
The output of the above example is one-two which results in the concatenation of two strings.
Example: Int a=1, b=3;
Output: a+b= 4
Example: Consider a code snippet given below.
Output
This "+" operator, when used with Integer, gives output as 4 which is the addition of two numbers. Since "+" operator is providing two different use cases when used with different data types it results in operator overloading. In addition to this, operators like -, *, and /, are also used for overloading operators for int as well as floating points.
Learn More
If you want to learn more about polymorphism in Java, head over to Polymorphism in Java.
Conclusion
I hope you like this blog. To conclude what have we covered in this article:
- For Compile time polymorphism in Java also known as static polymorphism, early binding is performed.
- Early binding refers to the process where the compiler determines the type of object and the method is resolved during compilation time.
- Method overloading is declaring two or more methods with the same name as that of a class and varying the declaration of parameters.
- At Compile time polymorphism in Java, the declared type of reference is used to determine which method will be executed at the runtime.
- It is used for adding functionalities, behavior, and features in Java code.
- Operator overloading refers to using the same operator on different data types.
- For example: addition of two integer (1+3)=4 gives output as 4 whereas "+" operator when used with strings gives output as concatenation of two strings ("a"+"b")= "ab".
- Operator overloading is not supported in Java, however, it can be achieved by method overloading.
These are a few takeaways from this article. Hope you liked it.