Concrete Class in Java
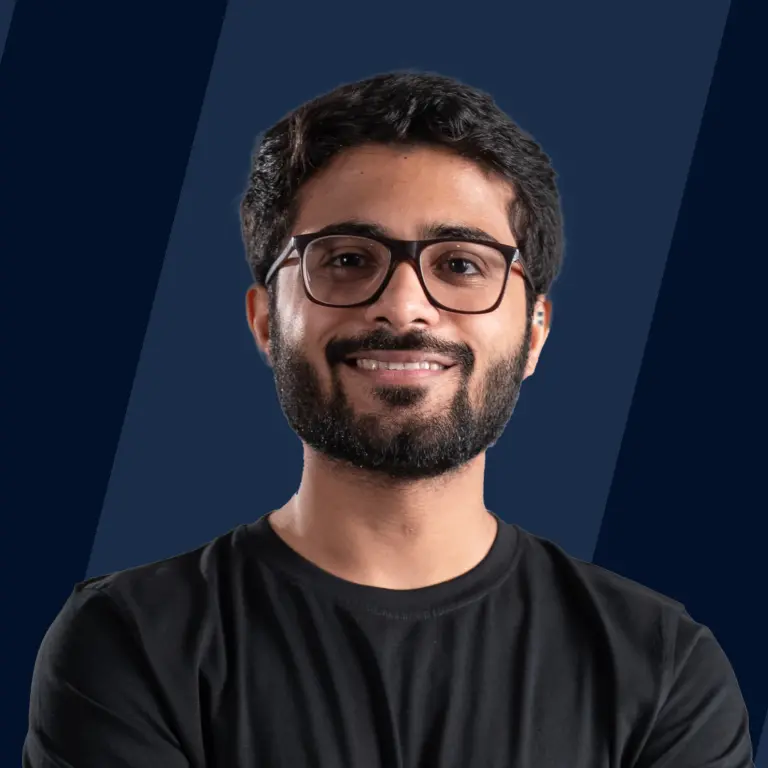
Overview
Concrete classes are Java classes that have code that implements all their methods, and method bodies are complete. Concrete classes in Java are known as 100% implemented classes. A concrete class can be instantiated as an object (like any other Java class).
What is a Concrete Class?
- A concrete class in java is a class that has all its methods implemented. For any class to be classified as concrete, it cannot have any unimplemented methods.
- Concrete classes can extend abstract class in Java or implement interfaces, but they must implement all the methods of the abstract class or interface they inherit.
- We can create an object of concrete class using the new keyword.
- A concrete class is an abstract class if it has even one abstract method, i.e. an unimplemented method because it doesn't meet the condition of being a concrete class.
- Concrete classes implement all methods and, in most cases, they inherit an abstract class or implement an interface. Java's final class is a class that cannot be inherited, so it's possible to declare concrete classes as final classes.
- As a concrete class inherits or implements all the methods from an abstract class or an interface, it can also be called a 100% implemented class in Java. Java methods from concrete classes are inherited or completely implemented from abstract classes or interfaces, which helps achieve 100% abstraction.
Syntax of Concrete Class in Java
A concrete class in Java is any such class that has the implementation of all of its inherited members either from an interface or abstract class Here is the basic syntax of the Concrete class:
Here, C is a concrete class that extends abstract class A and implements interface C. Class C has implemented methods from both class A and interface B.
Simple Concrete Class
Now, let's see how we can implement a simple concrete class using java code.
Output:
In the above code, the ConcreteCalculator class has 4 methods that perform arithmetic operations. All four methods add, subtract, multiply, divide are implemented and return the result. As all the methods of the class have been implemented ConcreteCalculator is a concrete class.
Example
The image below shows 4 classes Shape, Circle, Triangle, and Square. The shape is an abstract class with two unimplemented methods area(), perimeter(). The other three classes extend class Shape and implement both the methods area() and perimeter() of the abstract class. Hence, the classes Circle, Triangle, and Square are concrete classes.
Concrete Class Which Extends an Abstract Class
Before seeing the code implementation of a concrete class that extends an Abstract class let's understand what an abstract class means.
Abstraction in Java:
Abstraction is a process of hiding implementation and showing only essential details to the user. Abstraction is used in the case of banking applications to hide crucial details from end-users.
Abstract Class in Java:
- Abstract class in java is a class that is declared using the abstract keyword.
- It contains abstract methods which means it has incomplete or unimplemented methods.
- Abstract classes can have abstract as well as non-abstract methods.
- We cannot create objects of an abstract class to use abstract class methods abstract class needs to be inherited.
Program:
Let's consider a scenario. The shape is an abstract class with two unimplemented methods area(), perimeter(). The other three classes extend class Shape and implement both the methods area() and perimeter() of the abstract class. Hence, the classes Circle, Triangle, and Square are concrete classes.
Let's implement the above example of concrete classes using the java programming language.
Output:
In the above code, Shape is a abstract class with abstract methods area() and perimeter(). Classes Circle, Triangle, and Square inherit the abstract class shape and implement both abstract methods. As the classes Circle, Triangle and Square have all the methods implemented they are Concrete Classes.
Concrete Class Which Implements an Interface
First, let's understand the Interface in java. Interface:
- Interface in Java is a mechanism used to achieve abstraction.
- Interface is said to be a blueprint of a class. It contains only abstract methods and variables.
- Interface doesn't have a method body.
- Interface is used to achieve abstraction. It can also be used to implement multiple inheritances.
Program:
Output:
In the above code DemoInterface is an interface implemented by the abstract class DemoAbstract. The concrete class DemoConcrete implements the methods inherited from both the interface and the abstract class.
Java Abstraction vs. Concrete Classes
Abstract class in Java | Concrete class in Java |
---|---|
Abstract class can have unimplemented methods. | Concrete class cannot have unimplemented methods. |
Abstract class cannot be instantiated i.e. we cannot create an object of an abstract class. | Concrete class in java can be instantiated i.e. we can create an object of an abstract class using the new keyword. |
We can declare an abstract class using the abstract keyword. | Declaration of a concrete class is the same as of any normal class in java using the class keyword. |
It is impossible to create an abstract class without incomplete methods as an abstract class without any abstract method will be a normal java class. | On the other hand, concrete class in java should not have a single incomplete method. |
Abstract class cannot be declared as a final class. The reason is final class cannot be inherited and abstract classes are meant to be inherited and then used. | Concrete class can be declared as a final class as they have implementation for all the methods and need not be inherited. |
Abstract class should have abstract methods. | A concrete class implements all the abstract(unimplemented) methods of its parent abstract class. |
Conclusion
- Concrete classes in Java are fully implemented classes that implement all the methods.
- Every method that a concrete class contains must be implemented. A concrete class may extend an abstract class or implement an interface.
- To instantiate a concrete class in Java, the new keyword is used.
- A concrete class can also be declared as a final class.