Console in Java
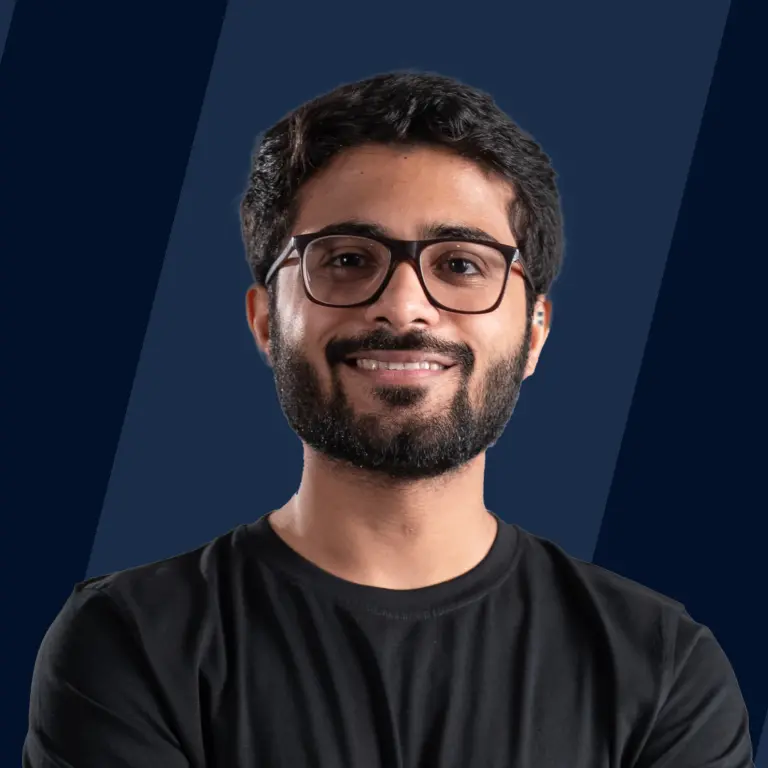
Overview
To get console input, we use the Java Console class. It offers ways to read text messages and passwords. If we read a password using the Console class, the user won't see it. Because System.in and System.out provide access to the majority of the Console's capabilities, it serves mostly as a convenience class. However, when reading strings from the console, its use can make some console interactions simpler.
Constructors are not provided by Console. Instead, using System.console() returns a Console object. A reference to the console is returned if one is present. Null is returned if not. In some circumstances, a console won't be accessible. Therefore, no console I/O is possible if null is returned.
Introduction to Console in Java
If there is a character-based console device connected to the active Java virtual machine, it can be accessed using methods provided by the java.io.Console class. The Console class was introduced in JDK 6, not JDK 1. JDK 6 added the Console class to the java.io API.
To obtain console input, you can use the methods provided by the Console class. It offers ways to read text messages and passwords. When reading a password using the Console class, the user's input remains hidden, ensuring that it is not displayed on the console.
The Console class provides an interface to the system console, allowing interaction with it. Since Java 1.5, the Console class has been available, not since JDK 1.
Console Class Declaration in Java
public final class Console extends Object implements Flushable
Java Console class extends the Object class which means it inherits all methods and attributes from the Object class, Object class is the superclass of the Console class. Console class implements Flushable Interface.
Different Methods of Console Class in Java
Method | Description |
---|---|
Reader reader() | Retrieves the Reader object associated with the console. |
String readLine() | Reads a single line of text from the console. |
String readLine(String fmt, Object... args) | Reads a single line of text from the console, displaying a formatted prompt before reading. |
char[] readPassword() | Reads a password from the console without displaying it. |
char[] readPassword(String fmt, Object... args) | Reads a password from the console, displaying a formatted prompt before reading. |
Console format(String fmt, Object... args) | Writes a formatted string to the console's output stream. |
Console printf(String format, Object... args) | Writes a string to the console's output stream. |
PrintWriter writer() | Retrieves the PrintWriter object connected to the console. |
void flush() | Flushes the console, ensuring any buffered data is written out. |
Reader reader()
The Reader reader() method in the Java Console class is used to retrieve the Reader object associated with the console. This Reader object allows you to read character-based input from the console, enabling you to interact with the user and obtain textual input.
Example:
Output:
Explanation:
In this example, we first obtain an instance of the Console class using System.console(). We then use the reader() method to retrieve a Reader object associated with the console. We read a sequence of characters from the console using the read(char[] cbuf) method, and then we convert the character array into a string and display it as the output.
String readLine()
A single line of text from the console is read using this method. In this example, we are going to see how to use readline() method in java.
Output
String readLine(String fmt, Object... args)
This method is used to read a single line from the console, providing a formatted prompt to the user.
Parameters:
- fmt: A format string used for the prompt text.
- args: The arguments referenced by the format specifiers in the format string.
Return: The method returns a string that is read from the console. If the stream is ended or closed, it returns null.
Exceptions:
- IllegalFormatException: This exception is thrown when the format string contains unsupported format specifiers or has illegal syntax for the provided arguments.
- IOException: This exception can be thrown if an I/O error occurs during the reading process.
In this code, we are going to use readline(String fmt,Object ... args) method of the console class to read a line from the console.
Output
Code Explanation
In the above code, we have given string format as %s %s, and "hello", and "world" are passed as arguments.
char[] readPassword()
This method is used to read a password or passphrase from the console, ensuring that it is not visible on the console while being typed.
Returns: The method returns a character array that represents the password or passphrase that was read from the console. The returned array does not include any line-termination characters. If the stream has come to a stop or is closed, it returns null.
Throws:
- IOError: This exception can be thrown if an I/O error occurs during the reading process.
In this example, we are going to use readPassword() method of the console class to read passwords from the console.
Output
Code Explanation
In the above code, we have used readPassword() method of the console class to read passwords from the console which will be stored in a char array. Notice, when you type the password, it is not visible in the console.
Console Format(String fmt, Object... args)
It is used to write a formatted string to the output stream of the console.
Parameters
fmt: A format string according to the Format string syntax definition.
args
In this example, we are going to use format() method of the console class to write a formatted string to the output stream of the console.
Output
Code Explanation
In the above code, we have written Items, Quantity, and Price details to the console with proper string format.
Console printf(String format, Object... args)
This method is used to write a formatted string to the output stream of the console.
Syntax
Parameters:
- format: The format string as defined in the format string syntax.
- args: The arguments referenced by the format specifiers in the format string.
Returns: The method returns the PrintStream object representing the output stream.
Exception:
- IllegalFormatException: This exception is thrown if the format string is invalid, such as containing unsupported format specifiers or having illegal syntax.
In this example, we are going to use printf() method of the console class to write to the console.
Output
Code Explanation
In the above code, we have used printf() method to write a formatted string to the console output stream.
Here %d is used to represent an integer.
PrintWriter writer()
The PrintWriter object connected to the console is retrieved using it.
Parameters: This method doesn't take any parameters.
Return value: Returns the PrintWriter object associated with the console.
Exceptions: This method does not throw any exceptions.
In this code, we are going to use writer() method of the console class to retrieve the unique PrintWriter object which is associated with the console.
Output
Code Explanation
In the above code, we have retrieved the unique PrintWriter object which is associated with the console object.
void flush()
The console is flushed using it.
- This method doesn't take any parameter
- This method does not return any value
- This method does not throw any exception
We are going to use flush() method to better understand it.
Code Explanation
In the above code, we have used readline() method to get string input from the user and printed that string. After that, we used flush() method to flush the console.
Ways to Get the Object of Console in Java
The static function console() of the System class returns a singleton instance of the Console class.
Syntax
Code
Output
Code Explanation
We have used System.console() to get the object of the console class in java.
Ways to Read Java Console Input
Reading user input from the command line using the Java console is becoming increasingly popular. Additionally, the format string syntax (like System.out.printf()) can be used to receive input that resembles a password without echoing the user's input.
Advantages
- Reading a password without repeating the characters that were typed.
- Reading techniques are coordinated.
- Syntax for format strings is available.
- Does not function in a non-interactive environment (such as in an IDE).
Example
Example 1
In this example, we are going to see how to take input from users using Console class. Code
Output
Example 2
In this example, we are going to use readPassword() method of the console class to read passwords from the console
Output
Conclusion
- The Java Console class provides functionality for obtaining console input and is capable of reading both text messages and passwords securely.
- When reading passwords using the Console class, the user's input remains hidden, ensuring privacy and security.
- The Console class serves mostly as a convenience class because most of its capabilities can be accessed through System.in and System.out. However, using the Console class can simplify interactions with the console when reading strings.
- The Console class does not have constructors. Instead, the System.console() method is used to obtain a reference to a Console object.
- When calling System.console(), it returns a reference to the console if one is available. If not, it returns null. In cases where a console is not accessible, console I/O operations are not possible if null is returned.