Constructor Chaining in Java
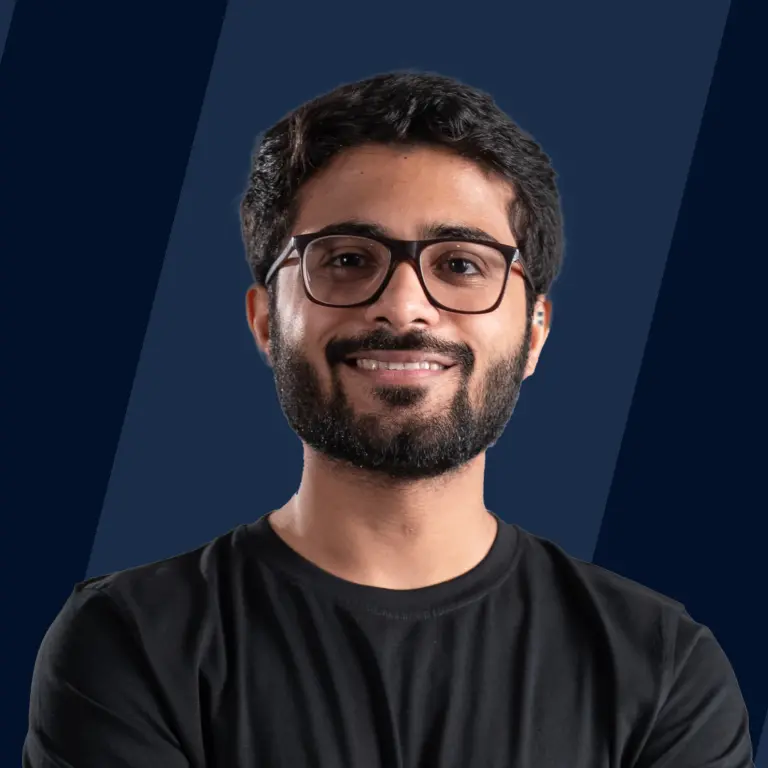
A constructor in Java is used to initialize an instance of a class. Also, a constructor can call other constructors of the same class or super-class, while the constructor call must be the very first call from the other constructors, and such a series of invocation of constructors is known as constructor chaining.
What is Constructor Chaining in Java?
Constructor chaining is the process of invoking a series of constructors within the same class or by the child class's constructors. In Java programming, constructor chaining generally happens as a result of the Inheritance process. When we are chaining constructors in a Java program, the first line of a constructor should always start with either this() or super() method according to the type of constructor chaining we want to implement. We use this() method to call a constructor from another overloaded constructor in the same class, and the super() method to invoke an immediate parent class constructor.
Now, let's see how we can implement the chaining of constructors in Java.
Ways to Implement Java Constructor Chaining
There are two ways in which constructor chaining is performed in Java, let's see the two ways below:
1. Constructor Chaining Within the Same Class:
We make several constructors with a different number of parameters (or with the different data types of parameters) within the same class and make a chain with these constructors using the this() method, we use this() method to call a constructor from another overloaded constructor in the same class. Let's see a Java example to understand it better:
Example Java Program:
Output:
Explanation:
- We can understand from the above image that when we created an object employee of the Employee class, it invoked a constructor with zero parameters as we have not passed any parameters while instantiating the employee object.
- In the first constructor, we have used this("NULL"); method as the initial statement, which invoked the second constructor with one parameter.
- In the second constructor, we have used this(name, 0); (name contains NULL string from the first constructor) as the initial statement, which invoked the third constructor with two parameters.
- The third constructor initialized the employee object with name containing NULL and empID containing 0.
- This series of invoking constructors from other constructors is known as constructor chaining within the same class.
2. Constructor Chaining from Parent/Base Class:
The objective of a sub-class constructor is to invoke the parent class's constructor first. This ensures that the initialization of the parent class's fields or member variables is performed as the first step in the creation of the sub-class's object. In Java, inheritance chains can contain any number of classes, every sub-class constructor calls up the constructor of the immediate parent class (using the super() method) in a chain until it reaches the top-level class. Let's see a Java example to understand it better:
Example Java Program:
Output:
Explanation:
- We have created a WagonR class that extends the Car class which also extends the Vehicle class, having certain fields defined in each class.
- We can understand from the above image that when we created an object car1 of WagonR class, it invoked the WagonR class constructor.
- In the WagonR class constructor, we have used super(name, type, doors, wheels); method (doors and wheels contains 4 passed from the WagonR constructor instantiation) as the initial statement, which invoked the Car class constructor initializing doors and wheels fields,
- In the Car class constructor, we have used super(name, type); method (name contains WagonR 2022 string and type contains Automobile string passed from the Car constructor), which invoked the Vehicle class constructor initializing name and type fields.
- The Vehicle class (Top-level) constructor is the first fully executed constructor and after that rest of the code in Car and WagonR constructors is executed respectively. This type of constructor chaining helps in initializing all the fields related to an object in one go.
Why do We Need Constructor Chaining?
- If we want to instantiate objects of a class with different types or numbers of parameters, we require different constructor definitions in the class. These different constructors can be made efficiently using constructor chaining.
- Constructor chaining in Java enables us to define various constructors for each task and connect them with links or chains to improve the quality of the code.
- If we don't chain constructors together and one of them requires a certain parameter, we'll have to initialize that parameter twice in each constructor.
Rules for Constructor Chaining
If we wish to use constructor chaining in Java, we have to follow the below guidelines:
- The constructor's definition should always start with the this() or super() method as the initial statement.
- There should be at least one constructor in the class that does not include this() method.
- The constructor chaining can be performed in any sequence.
Working of Constructor Chaining in Java
In Java, constructor chaining occurs as a result of the inheritance process. When dealing with the constructor of the parent class, the constructor of the subclass will call the constructor of the super-class first. This ensures that the parent class's members are initialized as the first step when a subclass object is instantiated. Every sub-class constructor calls up the constructor of the immediate parent class in a chain until it reaches the top-level class. However, a single constructor cannot invoke more than one constructor.
To understand how constructor chaining works, consider the following diagram:
Constructor Calling from Another Constructor
There are two methods for invoking the constructors:
-
Using this() method:
- The this() method is used to call one constructor from another constructor within the same class.
- The this() method can only be used inside a constructor.
- It must be the first statement with appropriate arguments in a constructor.
-
Using super() method:
- The super() method is used to call the immediate parent constructor from the child class constructor.
- The super() method can only be used inside a constructor.
- super() should also be the first statement with appropriate arguments in the child class's constructor.
Note: A constructor can have a call to super() or this() but never both.
Examples
Let us look at some more constructor chaining examples through Java programs:
Calling Current Class Constructor
If we wish to invoke the current class constructor, we utilize this() method in Java. this() method must be used since JVM never adds it automatically like the super() method. The constructor's first line must start with a this() method but inside a class, there should be at least one constructor that does not contain the this() method. Let's look at the syntax and an example to understand it better:
Syntax:
Example Java Program:
Output:
Explanation:
- We can understand from the above image that when we created an object acc1 of BankAccount class, it invoked a constructor with zero parameters as we have not passed any parameters while instantiating the acc1 object.
- In the first constructor, we have used this("2872", 0); method as the initial statement, which invoked the second constructor with two parameters.
- In the second constructor, we have used this(accountNumber, balance, "NULL", "NULL", "NULL"); as the initial statement, which invoked the third constructor with five parameters.
- The third constructor initialized the acc1 object with accountNumber containing 2872, balance containing 0, customerName, email, and phoneNumber containing NULL in each field.
- This series of invoking constructors from other constructors is known as constructor chaining within the same class.
Calling Super Class Constructor
Syntax:
Example Java Program:
Output:
Explanation:
- We have created a Dog class that extends the Animal class having certain fields defined in each class.
- We can understand from the above image that when we created an object dog of the Dog class, it invoked the Dog class constructor with four parameters.
- In the Dog class constructor, we have used the super(name, weight); method as the initial statement, which invoked the Animal class constructor initializing name and weight fields,
- The Animal class (Top-level) constructor is the first fully executed and after that rest of the code in the Dog constructor is executed and the remaining fields are initialized.
If We Change the Order of Constructors
We can achieve constructor chaining in any order, even if we change the order of calling the constructors in a java program, it will still achieve a constructor chain. Let us look at a Java example to understand the same:
Example Java Program:
Output:
Explanation:
- In this example, we have only changed the order of constructors in which they are invoked, it may not be meaningful or efficient sometimes, but this is a way to show that constructors can be chained in any sequence.
- We can understand from the above image that when we created an object s1 of the Student class, it invoked a constructor with two parameters as we have passed two parameters while instantiating the s1 object.
- The third constructor first calls the second constructor which calls the first constructor.
- In the first constructor, we have only printed a message in the output console. Now, the control shifts to the second constructor.
- In the second constructor also, we have only printed a message in the output console, and the control shifts to the third constructor.
- In the third constructor, we initialized the name and rollNumber field for object s1 and printed a message on the screen.
An Alternative Method of Constructor Chaining Using the Init Block
We can include some specific code in the init block to make sure that this code is executed with constructors. Whenever we create an instance of a class, the init block always runs before the constructor code.
Syntax:
Example Java Program:
Output:
Explanation:
- We have made an init block in the Demo class which is invoked before the constructor whenever we instantiate an object of the Demo class.
- We can understand from the output that the init block is invoked two times, first when the zero parameter constructor is called and second when a single parameter constructor is called.
Conclusion
- A series of invocation of constructors within the same class or by base class is known as constructor chaining. Constructor chaining generally happens because of the inheritance property in Java.
- this() or super() methods should always be the very first statements in a constructor definition to perform constructor chaining.
- Constructor chaining in Java can be performed in any sequence and it helps in reducing duplicate code.
- A constructor can have a call to super() or this() but never both.