Constructor Overloading in C++
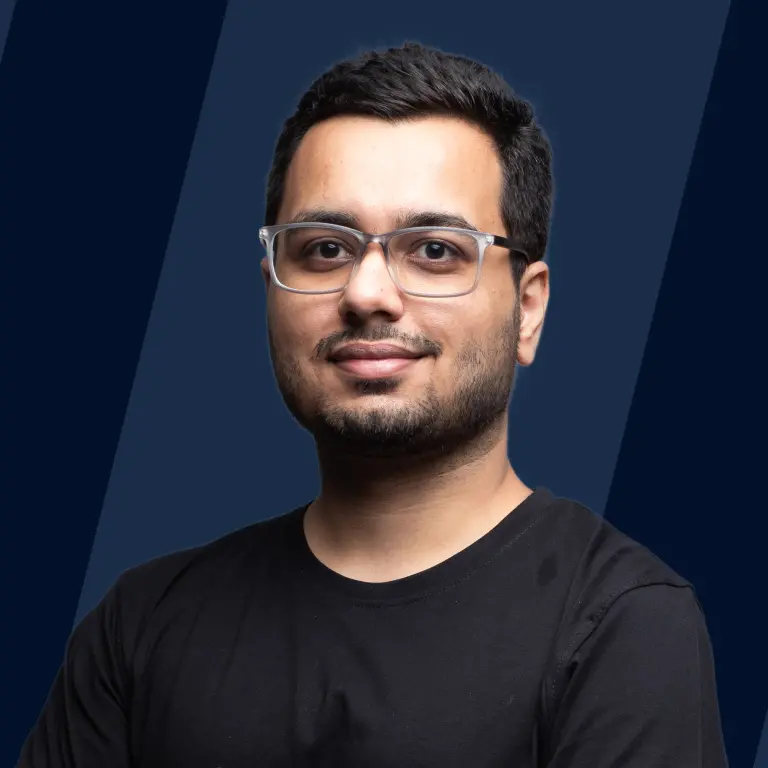
The use of multiple constructors in the same class is known as Constructor Overloading.
The constructor must follow one or both of the two rules below.
- All the constructors in the class should have a different number of parameters.
- It is also allowed in a class to have constructors with the same number of parameters and different data types.
Declaration
Here is the code syntax for the declaration of constructor overloading in C++:
Examples of Constructor Overloading in C++
Here are some examples of Constructor Overloading in C++:
Example 1
Here is a program to overload two constructors, one to set the name and age of a student with no parameters, and the second to set the name and age of a student with a two-parameter.
Output:
Explanation
In the above C++ program, we have created a class Student with two variables, Name and Age. We have also defined two constructors, Student() and Student(string str, int x). The first constructor is called when the object stu1 is created because we have not passed any argument. The second constructor is called when the object stu2 is created because we have passed two arguments with it. The get_Name() and get_Age() functions return the Name and Age, which we use to print the name and age of stu1 and stu2.
Example 2:
Here is a program to overload three constructors, one to set the area with no parameters, the second to set the area with one parameter, and the third to set the area with two parameters.
Output
Explanation
In the above C++ program, we have created a class Area with one variable, area. We have also defined three constructors, Area(), Area(int side), and Area(int length, int width).
- The first constructor, Area(), is called when the object obj1 is created because we have not passed any argument.
- The second constructor, Area(int side), is called when the object obj2 is created because we have passed one argument.
- The third constructor, Area(int length, int width), is called when the object obj3 is created because we have passed two arguments with it.
How Does Constructor Overloading Work in C++?
Here we are taking an example to understand the working of constructor overloading in C++:
Output
Explanation
Here in the above program, there are two constructors of class Calculate:
- A default constructor (with no parameters)
- The constructor with three-parameter
Two objects are created in the main() function.
- obj1: The obj1 will automatically call the default constructor without any parameters when it is created. This is due to the reason that no parameters are passed when creating the object. Thus, it complies with the definition of first(Default constructor).
- obj2: The obj2 automatically invokes the constructor with three parameters when it is created. This is because only three parameters are passed when creating the object. So, it matches the definition of the second constructor.
Conclusion
- Constructor overloading can be defined as having multiple constructors with different parameters so that every constructor can perform a different task.
- The constructor that does not take any argument is called the default constructor.
- A constructor that has parameters is known as a parameterized constructor.
- A copy constructor is a member function that initializes an object using another object of the same class.
- The advantage of constructer overloading is it provides flexibility in creating multiple types of objects for a class.