Constructor Overloading in Java
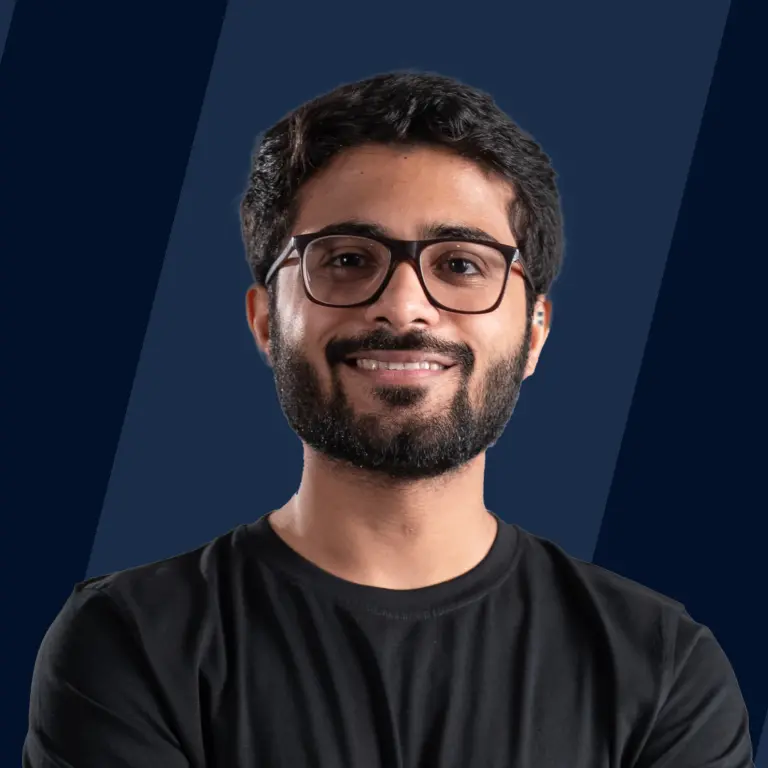
There are situations where we need to initialize an object in multiple ways. Constructor Overloading in Java allows us to achieve this flexibility. By defining multiple constructors with different parameter types or quantities in Java, we can execute different code sets to perform various tasks.
To learn about constructors in Java in detail, refer to the article Constructors in Java.
When Do We Need Constructor Overloading in Java?
Constructor overloading in Java allows multiple constructors to be defined within a class with the same name but different parameters. This facilitates flexibility in object initialization by enabling the creation of objects with different sets of initial states depending on the parameters provided.
The need for constructor overloading arises when a class requires different initialization for different objects. For instance, a class representing a geometric shape might have constructors accepting different parameters such as dimensions, colours, or starting coordinates. The class can handle various initialization scenarios by overloading constructors without needing separate method names.
Example
In the above example, we have a class designed to calculate the area of shapes. The constructor expects two arguments: width and height, making it versatile for various shapes.
However, if a user wants to calculate the area of a square, where only one dimension (either width or height) is required, attempting to use the existing constructor would result in a compile-time error. This limitation prompts the need for constructor overloading.
Example of Constructor Overloading in Java
Let's see how constructor overloading can create different instances of the Car class; each initialized with specific brand, model, and year values. This approach provides a convenient way to customize object creation and adapt it to diverse scenarios.
Explanation:
- The first constructor is a default constructor that sets the brand, model, and year to default values.
- The second constructor takes brand and model as parameters and sets the year to the default value.
- The third constructor takes brand, model, and year as parameters and sets the respective class variables accordingly.
By providing different options for initializing a Car object, we can create instances of the Car class with different combinations of arguments.
Using this() in Constructor Overloading
In Java, the implicit parameter "this" is crucial in denoting the current object within instance methods and constructors. It allows seamless access to variables, constructors, and methods within the context of a class.
Here are vital scenarios where "this" is employed effectively:
- When method parameters share names with instance variables, "this" helps distinguish between them, ensuring clarity and avoiding ambiguity in code.
- By using "this", methods can be chained together, enhancing readability and conciseness. Additionally, it references the current object, enabling manipulation or access within the method itself.
- In situations where multiple constructors exist within a class, the this() function facilitates constructor delegation.
Utilizing "this" in these scenarios optimizes code clarity, maintainability, and reusability, fostering efficient Java development practices.
Example
Output:
Here, we can see that we called the parameterized constructor, but since we called the default constructor using this() from the first line of the parameterized constructor, the default constructor execution completes first.
Conclusion
- Constructor overloading in Java allows multiple constructors with different parameter lists to be defined within a class.
- Compiler won't create default constructors if we define any parameterized constructors.
- Java does not allow calling recursive constructors.
- The default constructor is used to initialize the default values of data members of the class.
FAQs
Q: What is the difference between constructor overloading and constructor overriding?
A: Constructor overloading involves having multiple constructors with different parameter lists within the same class, while constructor overriding refers to the practice of a subclass providing a new implementation for a constructor defined in its superclass.
Q: What is constructor overriding in Java?
A: In Java, constructor overriding is not possible. Subclasses can't override constructors of their superclass. They can only call superclass constructors using super().