Copy in Python
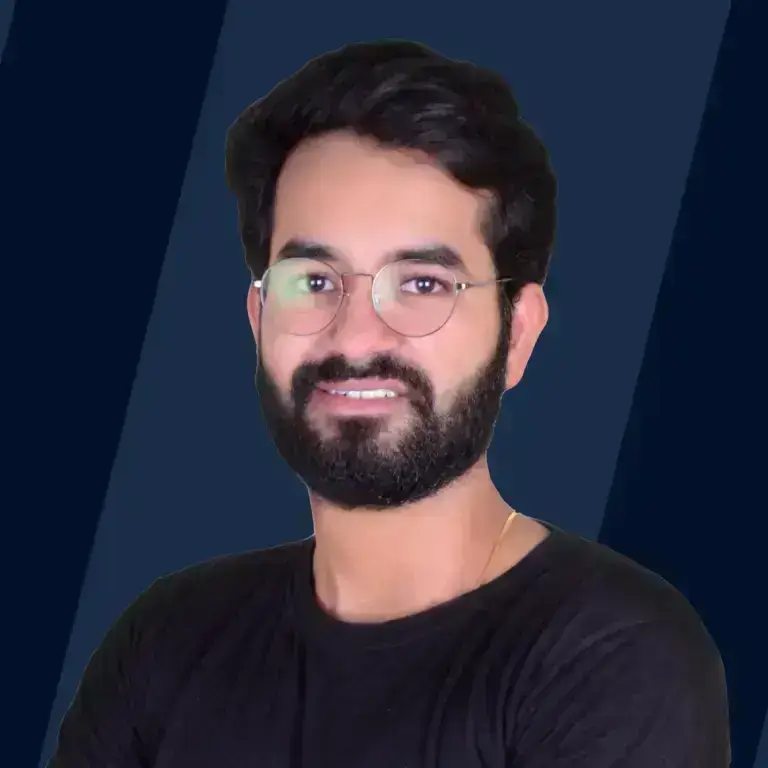
Overview
Copy() in Python Programming is a method that is used on objects to create copies of them. It returns a shallow copy of the list. Using the deepcopy() function of the copy module can provide a real/deep clone of the object.
Syntax of Copy in Python
The syntax of the copy() method in python is simple. The method is called on objects in the following way:
Parameters of Copy in Python
The copy method in python provided in the standard library does not take any parameter as input.
Return Value of Copy in Python
The python copy method returns a shallow copy of the object it was called on. We will discuss shallow copy soon.
Example
Code:
Output:
What is a Copy in Python?
Multiple times during programming, you might want to create a copy of objects that you were using. If you're using ints, floats, etc., you can create a new variable and use the assignment operator in Python to create a copy. However, using the assignment operator, these statements don't create 'copies' of the objects. Usage of assignment operator binds names to objects. It does not make any difference in immutable objects like ints, floats, etc.
But for mutable (changeable) objects like lists, we would look for methods to create "real copies" of these objects, meaning that sometimes you'd want copies of these objects so that when you make changes to the copy, you do not make changes to the original automatically. When we look at shallow and deep copies of concepts, it'll all make sense in a while.
Let's look at the normal way you'd create the copy of a list or a dict or set.
But this method would not work for custom objects (objects that you create), and using this method or the assignment operator would create what we call a shallow copy of the object in python. Now, what is a shallow copy in python?
When we talk about copying, there are two types of copies of an object - shallow copy and deep copy.
Shallow Copy - A shallow copy is the construction of a new collection object (like our list / dict / set) followed by adding references to the child objects from the original object. Shallow copies are just one level deep. The shallow copying process is not recursive, meaning the copies of child objects will not be created. Now, this sounds complex, but the crux is that in the case of shallow copy if we make a change to the copy of the original object, will reflect in the original object as well.
Deep Copy - Deep copy is the opposite of a shallow copy. In this case, the deep copy process is recursive. Like in shallow copy, we create a new collection object and recursively add copies of the child objects from the original. This way, we can produce a fully independent clone of the original object. Again, the crux is that if we make changes to the copy, they will not reflect in the original.
We'll see examples in the next section. Back to mutable objects like lists / dict / sets, and to create real clones of these objects, we need to create deep copies of them so that any changes made to the copies won't be reflected in our original object; the original will remain unchanged.
Let's see what happens if we make use of the assignment operator to make a copy of the list:
Code:
Output:
Did you see how the changes made in the copy reflected in the original list? This is exactly why we need to make real clones of the list, deep copies.
How to Use Copy in Python?
We use the' copy' module for a shallow or even deep copy in Python. The two functions - copy and deepcopy are used. The parameters for both functions are the lists we want to copy.
If we do not mind a shallow copy of an object, we can make use of the standard library function provided by python - copy(), which, as we saw earlier, does not take any parameters. However, for deep copying, we can use the copy module.
It is done in the following manner:
Code:
Output:
As you saw earlier, a shallow copy will modify the original list, but the original list remains intact when deep copying. Still, the modifications are reflected only in the deep copied list.
Here, we created shallow and deep copies of the original list in shallow_copy_list and deep_copy_list, respectively. When we changed deep_copy_list, we saw that the original copy remained unchanged. However, when we changed the shallow_copy_list, the same changes were also reflected in the original list.
Examples of Copy in Python
We just discussed examples of copying a list. However, we can make shallow and deep copies of other objects, even the ones we create.
Let's create a class - Point class (2-dimensional points).
Code:
In this point class, we created a Point object with two variables, a and b. And we have created a __repr__ function for ease of access to the values of the objects. Now we're going to see how the copy() functions work on objects that we have created.
Code:
Output:
In this case, our Point class uses immutable data types in python - the int and hence here, a shallow copy or a deep copy is the same. However, suppose we create a more complicated class using this Point class. In that case, we can differentiate between the two copy methods, including the meaning of shallow copy being a one level deep copy. We are going to have a hierarchy:
- class Rectangle (making use of type Point)
- class Point (making use of type int)
- int
- class Point (making use of type int)
Code:
Output:
Now that we have our setup ready let's make some changes to the shallow copy of our rectangle so that we can understand how the changes will be reflected.
Code:
Output:
Since we had created a shallow copy, the same changes were reflected in the original object! Let's now create a deep copy of the same object and see what happens next.
Code:
Don't worry; there's no specific reason for using the shallow_rect to create the deepcopy; you can also use the original rectangle and observe similar results.
Output:
As you can see, the changes have not been reflected in the original object. If you wish to copy any object in python shallowly, you can use the copy() method. However, if you need to deepcopy or make real clones or real copies, you can import the copy module and make use of the copy() and deepcopy() functions.
You now know how to shallow and deep copy in Python!
Conclusion
- Syntax of the copy method in Python: object.copy(). This method returns a shallow copy of the object.
- In the case of a shallow copy, changes made to the copy will reflect in the original object; however, in the case of the deepcopy, changes made to the copy will not be reflected in the original object.
- To copy arbitrary objects or deepcopy objects, use the copy module.
- Shallow copy: copy.copy(object)
- Deep copy: copy.deepcopy(object)