Why do C++ Asserts Matter?
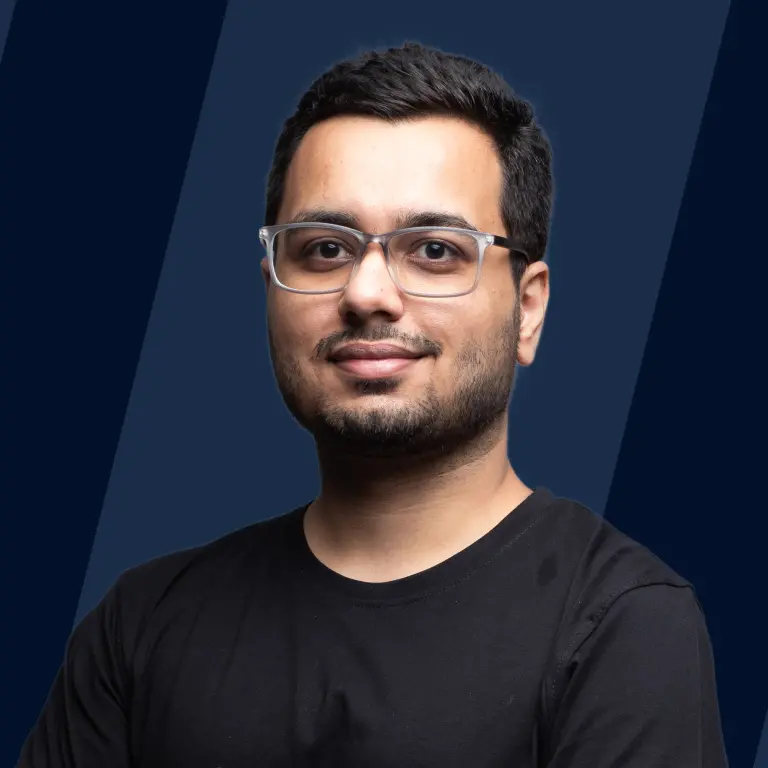
C++ Assert statements are used excessively during the debugging phase by the programmers as opposed to generic error handling statements, as C++ assert statements are very simple to use and can be disabled at once.
All the assertion statements can be deactivated simultaneously with only one macro run, unlike the generic error handling statements that must be deleted directly from the code once the issue is fixed.
It is used to test the programs that are difficult to occur without an error. Since it ends the program when the assertion is false, it is used as a debugging tool.
What is C++ Assert?
About
The purpose of C++ assertions is to test a programmer's assumptions in a program. As an example, we might use C++ assert to determine whether or not the pointer returned by the malloc() function is NULL.
If the expression passed in the C++ assert() function evaluates to 0 or false, the abort() method is invoked and the expression, source code filename, and line number are reported to the standard error.
Syntax
Following is the syntax for how to use C++ assert() function:
Declaration:
Parameters
C++ assert() has one parameter, i.e., an expression that returns a true (1) or false (0) value.
The program is terminated, if this expression evaluates to false (0) since it results in an assertion failure.
Return value
None, C++ assert function does not return any value.
Example
Let's see a C++ assert example, we will check if the entered number is an odd number or not. If the assertion fails the program terminates and the compiler throws an assertion failed error.
Input 1:
Output 1:
Input 2:
Output 2:
Explanation:
In the above C++ program, the programmer has assumed that the entered number will be odd. Now to check the same, we have used a C++ assert function by passing an expression (oddNumber % 2 != 0) as an argument. If we enter an even number, the compiler will throw an assertion failed error.
How to Create Assertions in C++?
The assert preprocessor macro, which is defined in the cassert header file, is used to implement the assertions in C++.
After importing this pre-processor file, the following syntax may be used to make an assertion:
- The assert outputs an error message and ends the program if the value is 0 (false).
- It accomplishes nothing and the application continues to run normally if the value is 1 (true).
Advantages and Disadvantages of C++ Asserts
Following are the advantages and disadvantages of the C++ assert function:
Advantages:
- In various sections of the code, assertions statements can be used to explicitly check for logically faulty situations. Checking for logically flawed statements that may be syntactically accurate is helpful to programmers.
- The assertion statement allows us to instantly turn off all error messages for the whole application. As opposed to standard error handling, where each instance of an error has to be manually eliminated, assertion statement calls just require the execution of a single macro to complete (NDEBUG).
Disadvantages:
- The assertion statement's inability to be executed at execution time is its main drawback when used for error management. While debugging the code for the intended execution, assertion statements are used to look for potential flaws but the C++ assert function ends the program and halts its execution altogether.
- The issue with C++ assert function is that while manually throwing an exception increases needless run-time costs, assert and abort both quickly stop the application without invoking the destructors.
What are Static C++ Asserts?
Assertions at the run-time of a C++ program are performed using the C++ assert function. However, assertions that are made at the compile-time of a program are performed using the C++ static_assert function.
Following is the syntax for the static_assert function:
- const_boolean_expression parameter represents an expression that is specified at the compilation time.
- message parameter represents the message to display when an assertion is false.
We don't need to include a header file because static_assert is already defined as a keyword in C++.
Example C++ Program:
Explanation:
To ensure that the integer size is at least 4 bytes on the platform where the code is running, we have used the static_assert function. The assertion will fail if the application runs on a 16-bit machine because the sizeof(int) in this case will be 2 bytes.
When to use Assertions in C++?
Unreachable Codes
When we attempt to run a program, there are codes that do not execute. We can make sure the inaccessible codes are indeed unreachable by using C++ assertions.
Let's look at an example:
Documenting Assumptions
Many programmers use comments to describe their underlying assumptions in their programs. Take this as an example:
Instead of comments, we can use C++ assertions.
As the program expands, comments in C++ may become out-of-date and out-of-sync. But we have to update the assert macros, or else they may fail under valid conditions.
When Not to Use Assertions in C++?
Public Function Argument Checking
The user may supply arguments in public functions, therefore, if these arguments are checked by an assertion, it may fail because of the conditions, which can lead to an assertion error.
In place of utilizing assertions, let the relevant run-time exceptions be thrown and handle the public function argument exceptions.
While Evaluating Expressions that Affect Program Operations
Avoid using methods or evaluating exceptions that have unintended consequences. For example,
The expression (++x && x % 2 == 0) has consequences in the example above, because we are changing the value of the variable x by incrementing it.
Consequently, we may write the program as opposed to evaluating the expressions inside the test macro:
This ensures that x has the same value in the program, whether the assertion is enabled or disabled.
Disabling Asserts
Assertions are tested at the run-time of a program. Although assertions speed up the debugging process of our program, care should be taken to avoid them in the application's release build. That is why we only release an application after being certain that it has undergone extensive testing.
Using the NDEBUG macro, we can stop a program from making assertions. When the NDEBUG macro is used in a program, all assert calls are blocked.
To remove all assert statements from the program, we may add the line shown below.
Example C++ Program:
Disabling all the assertions in a C++ program using the NDEBUG macro:
Output:
Explanation: We have defined the NDEBUG macro before the #include<cassert> statement, so we can disable all the assertions in the above C++ program. We can see from the output that the assert(2+2 == 5) is disabled and the next statement is executed.
Examples of Assertions in C++ for Understanding.
- Checking the assumption of the user that the input will be less than or equal to 5.
C++ Program:
Input 1:
Output 1:
Input 2:
Output 2:
Explanation: We have used a C++ assert x <= 5 to check the assumption that the input will always be less than equal to 5 is true or false. We can see from the output that if the input is we get an assertion to fail error.
- Checking the assumption of the user that the number of elements in an array will be between and .
C++ Program:
Input 1:
Output 1:
Input 2:
Output 2:
Explanation: We are using a C++ assertion to check the assumption of the user that the size of the array is between and . We can see from the output that if we enter size to be , the assertion is true and doesn't give an error, but if we enter , the assertion fails and gives a run-time error.
Conclusion
- C++ Assert statements are used excessively during the debugging phase by the programmers.
- The assert preprocessor macro, which is defined in the cassert header file, is used to implement the assertions in C++.
- Assertions that are made at the compile-time of a program are performed using the C++ static_assert function.
- We can make sure the inaccessible codes are indeed unreachable by using C++ assertions.