C++ Check if File Exists
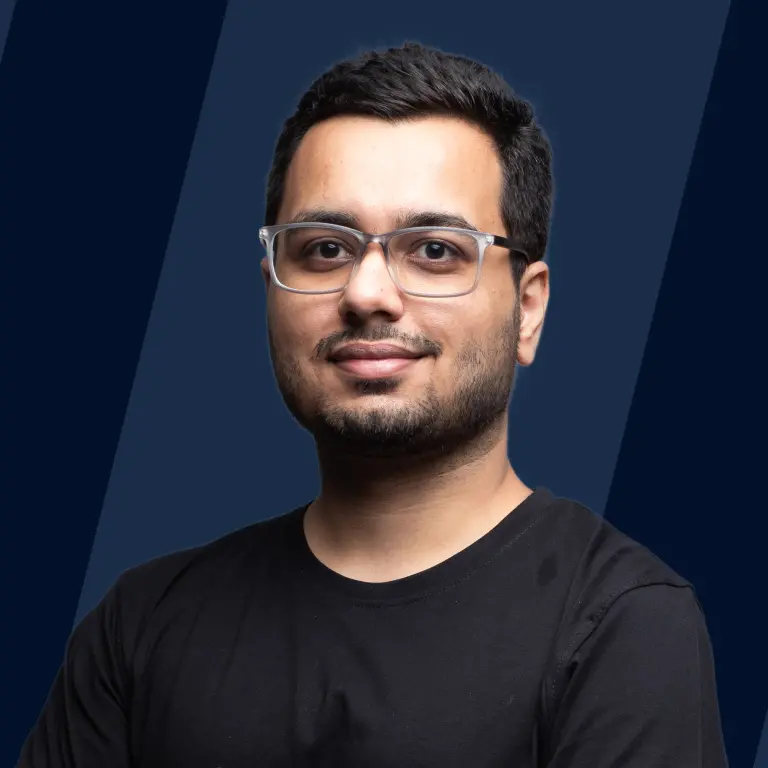
Overview
Files are entities that are used to store data in our systems. Whenever we want to do some work involving files, let's say backing up some already existing files, modifying the contents of some file, reading any system or user-created file, or something else too, we first need to be sure that the file exists.
Different ways to Check if a File Exists
Let's now discuss some of the ways through which we can check if a file exists or not in C++
1. Using open() Function with ifstream Object
In this example, we will be checking if a file exists or not using the open() function defined inside the fstream header file.
Note - In my present workspace the file "test.txt" exists, if we write the name of a file that is not present, then the program will output accordingly.
Program - c++ check if file exists
Output
Explanation
- Starting from the main() function, we are creating an ifstream class object named file to read the target file later, then after we are calling the open function on the file object with the target file name as the parameter.
- What this line does is, it will attempt to open the "test.txt" file in the read mode, now a file can only be opened if it is present there, we can not open a file that does not exist, so indirectly, here we are checking the existence of the file using the open() function.
- Then we are checking if the file object has opened the file or not using the if-else conditional statements, if the file was opened, meaning it exists on the provided path, hence printing the success message, else printing the error message.
Remember - We can even give the path of the files which are present in some else directory. For example - let's say there is a file named "parent.txt" present in the parent folder of the current working directory, then we can give the path as file. open("../parent.txt"), and the program will work as it should, it will print the success message if the file was found there at the provided path, else will print the error message.
2. Using the Function fopen()
In the below example, we will be checking if a file exists or not using the fopen() function defined inside the fstream header file.
Note - In my present workspace, the file "test2.txt" is not present.
Program - c++ check if file exists
Output
Explanation
- Starting from the main() function, we are creating a pointer of the FILE class or simply a FILE pointer to read the file, after that, we are calling the fopen() function with "test2.txt" and "r" as parameters, specifying the target file we want to work on, and the operation we want to carry on that, here the operation is "r" ie. we want to read the file.
- After fopen() has been executed, we are storing the return value of the execution in the pointer file. The function fopen() will return a file stream pointer pointing to the target file if the file opening was successful, which means if the file has existed in the first place, else will return the value NULL in case of fopen() was unable to open the file, meaning if the file has not existed.
- Then we are checking if the file pointer is not NULL, and if that is true, then we know that the file exists, hence printing the success message, else printing the error message.
3. Using std::filesystem::exists
In the below example, we will be checking if a file exists or not using the std::filesystem::exits
Program - c++ check if file exists
Output
Explanation
- Starting from the main() function, we are creating a vector of string values containing the name of certain files which we want to check if they exist or not.
- After that we are iterating over the above vector and check individually on each file name if it exists or not using the built-in exists() function defined inside the filesystem header file, and if the file is found, then we are printing the success message, else printing the error message.
Remember - The filesystem header file may not be by default present in your system, you might need to install it manually by yourself to work with the above program.
Practical Use Case of the Above Operation
1. Navigating to a Particular Directory and Checking if a File Exists or Not
In the below example, we will be seeing how we can navigate to any specific directory and can check if a file exists there or not with the help of a program.
Program - c++ check if file exists
Output
Explanation
- Starting from the main() function, we are creating an ifstream class object named file to read the target file, then after we are defining the path of the file we want to check exists or not.
- After that we are calling the open function on the file object with the provided path as a parameter, with this we are trying to open that specific file present at that path, and we know that a file can only be opened if it exists there, so indirectly, here we are checking the existence of the file into a particular directory or at a path.
- Then we are checking if the file object has opened the file or not, and if the file was opened, meaning it exists on the provided path, then we are printing the success message, else printing the error message.
Note - We can also take the path as the user input and can look for the existence of a file at the provided path.
Examples
1. Checking if a File exists or not and Writing Some Data into it
In this example, we are going to check if a file exists or not, if it exists we will be writing some data into it and if it does not exists, then we will create a new file and will write the given data into that.
Program - c++ check if file exists and write some data into it
Output
Explanation
- Starting from the main() function, we are first creating a FILE pointer to operate with the files, then after we are trying to open the specified file in reading mode, we are initializing a char array with the data that we want to write into the file.
- After that we are checking if the file opening was successful or not, meaning if the file exists or not, if it exists, we are printing the success message, and are again opening the specified file into write mode and are writing data into it using the fputs() function.
- If the file does not exist, then we are firstly printing the error message and then creating a new file with the specified name (a file automatically gets created with the specified name when it is opened in write mode if it does not exists), after that, we are writing the given data into the file.
FAQs
Q. From here to learn more about File Handling?
A. Kindly have a look at the below-mentioned articles based on file handling concepts, to understand the topics in a better way. Read more about File Handling in C++
:::
Conclusion
- There are various works we do with files in our day-to-day tasks, like opening a file, modifying some file, creating a new file, deleting a file, etc., and for these things to happen, we must be firstly sure that the file exists.
- There are various ways through which we can verify if a file exists or not with the help of our program, there are numerous built-in functions in c++, that we can use to accomplish this task.
- Functions like open(), fopen() etc. can be easily used to determine whether a file exists on our system or not, we can also check if a file is present or not in some else directory as well, by writing the appropriate path as the argument while calling these functions.