What are C++ Global Variables?
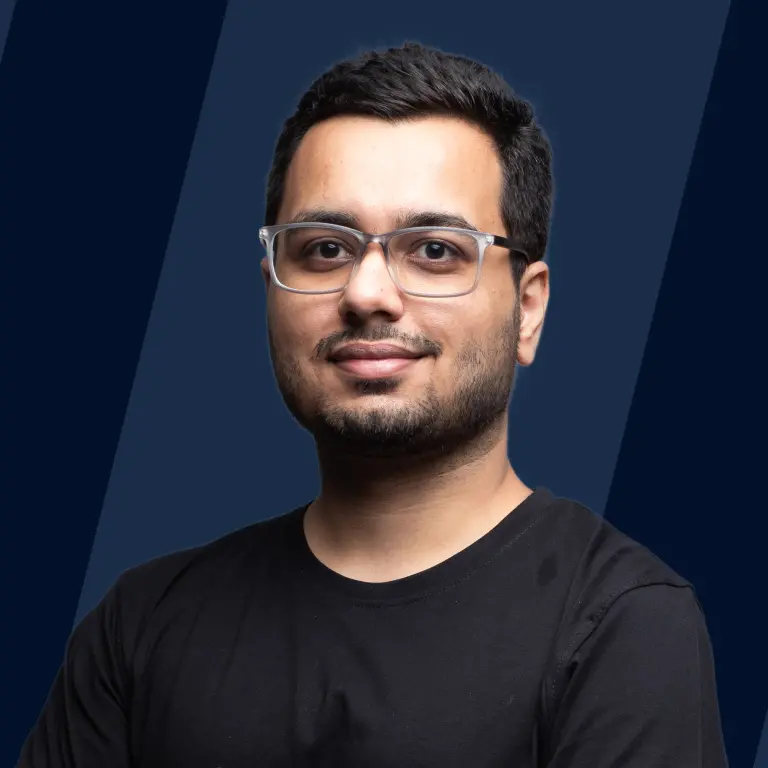
The scope of a variable refers to the extent of the block of code in which the variable can be accessed, modified, and worked with. There are mainly two kinds of variable scopes.
- Global Variables
- Local Variables
What are Global Variables in C++?
Definition of C++ Global Variable
A global variable can be accessed from anywhere in the entire program. It is usually declared at the top or start of the program outside of all blocks and functions of the program. It can be accessed from any function without any error.
Declaration of a Global Variable in C++
The global variable is declared at the top of the program outside any function or block of code. Let's see an example to understand this better.
Output
Explanation
We declare a global variable a and initialize a to the value 5 in Line 5. We access the variable and print its value in Line 10. We modify the value of a in Line 13 and print the value of a in Line 16. The global variable can be accessed and modified anywhere in the entire program.
What if a Local Variable Exists with the Same Name as that of a Global Variable Inside a Function?
If we define a local variable with the same name as a global variable, then what will happen? What will happen when we call this variable? Which variable - local or global gets called. Or will we get a compilation error.
If the scope of two variables is the same,i.e., both are global variables we will get a compilation error. However, if we call the variable, when it has different scopes, i.e, global and local, the compiler gives preference to the local variable as shown in the following example.
Output
Explanation We declare a global variable a and initialize it with value 17 in Line 5. We then declare and initialize a local variable a under the main function. The scope of the local variable is the main function while the global variable can be accessed from anywhere. We print the value of the variable a in Line 13. The value printed is 12. The local variable takes precedence over the global variable.
How to Access a Global Variable when There is a Local Variable with the Same Name?
So how do we access the global variable when there exists a local variable with the same name. To do that, we use the scope resolution operator as shown below.
Output
Explanation We declare a global variable a and initialize it with the value 3 in Line 5. We then declare and initialize a local variable a with the value 7 under the main function. When we access the variable with the scope resolution operator, it prints the value of the global variable and when we access it without the scope resolution operator, it prints the value of the local variable.
Working of C++ Global Variables
The accessibility of a variable is defined by the scope of the variable. There are two types of scope variables in C++ - local and global variables. Local variables are those which are declared inside a function or a block({}) of code. Local variables are accessible inside the function or block of code only. If we access a local variable outside its scope,i.e. the function or block in which it is declared, we will get a compilation error.
However, global variables are declared at the top of the program outside any function or block of code. These variables can be accessed from within any function of the block of code. They can be accessed and modified anywhere in the program without any compilation error.
Examples of C++ Global Variables
Example 1
Output
Explanation
We declare a global variable a at the top of the program and initialize it with the value 5. Inside the main function, we print its value in line number 18. Then we modify the value of a and print its value. In-Line number 27, we call a print function which modifies the values of a and prints its value.
Example 2
Output
Explanation
We declare a global variable product in Line 5. We declare and initialize two local variables a and b inside the main method. In Line 13, we assign the value of a*b to the variable product. In Line 16, we print the value of the variable product to the screen.
Related Articles:
Conclusion
- There are two types of variables - global and local.
- Global variables are declared at the top of the program outside any function and are accessible from anywhere in the entire program.
- Local variables are declared inside a function or a block of code and are accessible only within the function or the block of code in which it was declared.
- In case both local and global variables are present with the same name, the compiler gives preference to the local variable when it is called by any method.
- In case both local and global variables are present with the same name, we use the scope resolution operator(::) to call the local variable.
Stand out in the coding world with a C++ certification. Enroll in our Free C++ course now!