What is the size_t in C++?
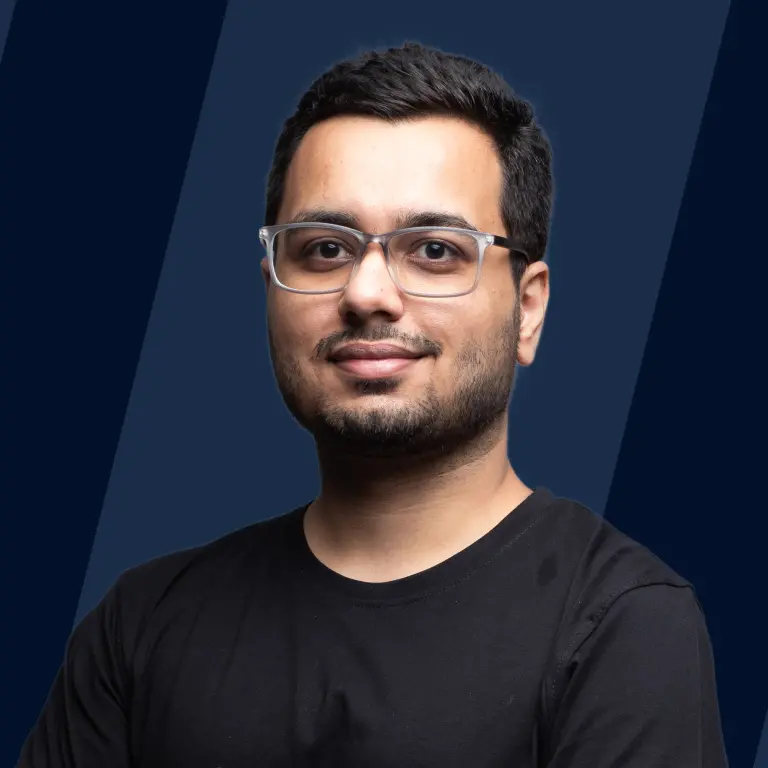
size_t in C++ is the type returned by the sizeof operator and is frequently used in the standard library to describe sizes and counts. It is a type that may express the size of any object in bytes.
The maximum size of an object of any type that is technically feasible can be stored in size_t (including array). A type is ill-formed if its size cannot be expressed by size_t. size_t is equivalent to uintptr_t when it can reliably store the value of any non-member pointer, which is the case on most platforms (systems with segmented addressing being an exception).
What are Some use cases of size_t?
Below are some use cases of C++ size_t:
The primary use of size_t in C++ is for loop counting and array indexing provided by the standard template library in C++. Programs that rely on 32-bit modular arithmetic or use other types, for example - unsigned int and indexing of array, may break on 64-bit platforms whenever the array index exceeds UINT_MAX.
The secondary use of C++ size_t, the member typedef size type offered by C++ containers like std::string, std::vector, etc., is the correct type to use when indexing them. It is normally used to refer to std::size_t.
Important Points to Remember about size_t
- The syntax for using size_t:
size_t var = val;.
There are two parameters var and val.
- var : variable name.
- val : the value to assign to that variable.
- A data type called size_t can represent any object's size in bytes.
- The size_t type is frequently used in array indexing and loop counting because it makes it simple and secure to save the contents of any non-member pointers.
- The type size_t can never contain a negative value.
- For returning sizes and lengths, this size_t is also returned by a variety of string and num functions, including strcspn(), memchr(), memcpy(), strlen(), and strspn().
Examples of C++ size_t
Output:
Explanation:
The size_t type can hold the largest object size in the system but can only have positive numbers, even though it can hold much larger numbers than the int type. In the program above, we can see that we are printing the largest value of the int type and the lowest value that the int type can hold. To output the maximum and minimum values an int type can hold, use the variables INT_MAX and INT_MIN. As a result, we can observe the output for both types of sizes.
Example
To display the size of the array and display the elements of an array type with small numbers is obtained by SIZE_MAX.
Output:
Explanation:
We can first see that a variable named s was recently declared in the above program to display its size. It can hold after being defined with the size_t type, and since we are storing it as an array, the size of this array is determined by SIZE_MAX. Next, we are attempting to display the array-type elements in small numbers because 1000 is too many to show in the output, so we have only chosen to display 50. We are starting with index 0 using the size_t type, demonstrating how we may utilize size_t for indexing and counting. The array will then be presented in descending order since the numbers will be decremented.
Fixing Compiler Warning using size_t
This type of compiler warning can be fixed in one of two ways.
- The length() member function return value can be type converted to an integer.
- Instead of explicitly converting str.length() (which returns size_t ) to int, we can declare our i variable of data type size_t.
Examples of Some Common C++ Functions Internally using size_t
Here are some common C++ functions internally using size_t C++.
- strlen()
- length()
- strcspn()
- memchr()
- memcpy()
- strlen()
- strspn()
Conclusion
- The size_t type in C++ is likewise an unsigned integer type, just like the unsigned int type given by the C++ standard library, from which we can also obtain the size_t type.
- Unsigned int and size_t are equivalent in C++, however it is recommended to use size_t when working with positive and large integers.