C++ String Contains
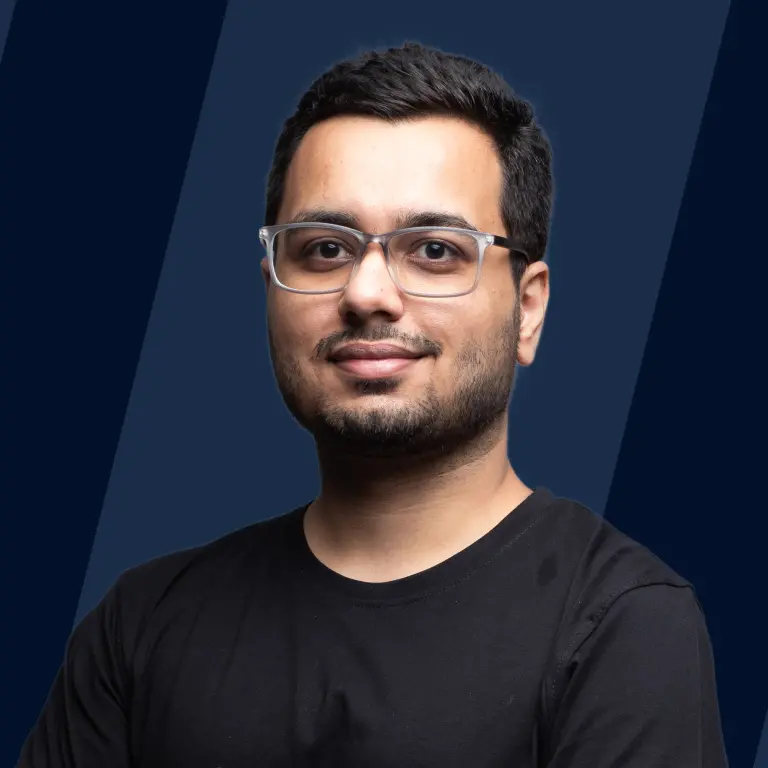
Overview
Many times in our Program, we would be in a need to check whether some specific substring is part of a given string or not. Therefore, the C++ language provides a variety of pre-defined standard methods like - find(), contains(), strstr(), etc., using which we can verify that. Most of these methods operate on the main string and take the target substring as an argument, and return the result of whether the match was successfully found or not.
Introduction
The standard string library in C++ provides various pre-defined methods to verify whether a given substring is a part of any given string or not. Let's have a look at the examples to understand them better.
Different Methods to Check If a String Contains Another String as a Substring
Using String::find()
The find() function in C++ is the most straightforward approach to finding out whether a substring exists in a given string; if found, the function returns the index of the first matched result, else it will return string::npos. string::npos means not found. It is a constant value (probably -1 in most compilers) representing a non-position value.
Program to check if a C++ string contains a specific substring using the function string::find()
Output
Explanation
First, we are initializing our main string and the substring we want to search. After that, we are calling the find() function to check if the string s2 is present in the string s1 and are storing that value in the boolean isFound. If the value returned is true, i.e., the substring is found, we are printing the success message, else we are printing the value not found message.
Using Basic_string::contains()
We can also use the basic_string::contains() function to check if a substring exists in a given substring or not; the point to remember here is the basic_string::contains() function is a part of the C++ 23 release. So it may be available in some of the compilers as of now.
Program to check if a C++ string contains a specific substring using the function basic_string::contains()
Output
Explanation
We are again initializing our main string and the substring we have to search. After that, we are calling the contains() function on the string s1 with s2 as a parameter to check if s1 contains the string s2 or not and are storing that value into the boolean isFound. If the value returned is true, then we are printing the success message; else are printing the value not found message.
Using Strstr() function
Here in this example, using the function c_str() firstly, we will be converting both the c++ styled strings to c-styled strings and then will be comparing them using the strstr() function; the function will return the index where the match is found else will return a null pointer.
Program to check if a C++ string contains a specific substring using the function strstr()
Output
Explanation
First, we are initializing our main string and the substring we want to search. After that, we are calling the strstr() function, and inside that, we are passing the strings s1 and s2. Still, before passing, we are converting them into c-styled string values using the function c_str(), and the converted string gets passed into the strstr() function, the strstr() function will return a pointer to the first character in the main string where s2 is found. If it is not found, it will return a null pointer. We are then storing the returned result into a char pointer, isFound. And if any valid value is returned, then we are printing the success message, else we are printing the value not found message.
Using Boost Library to Check if A String Contains a Substring in C++
We can also use the boost library to check if a substring is present in a given string or not. Remember, the boost library may not be available by default on your system; in that case, you may need to install it manually.
Program to check if a C++ string contains a specific substring using the boost library
Output
Explanation
Here we are initializing our main string and the substring we want to search. After that, we are using the contains() function from the boost library to check whether s2 is contained in s1, and are storing the returned value in the boolean isFound. If the returned value is true, then we are printing the success message, else we are printing the value not found message.
Using Custom Functions to Check if a String Contains a Substring in C++
In this example, we will be writing our custom function to verify it a substring is a part of a given string or not.
Program to check if a C++ string contains a specific substring using a custom function
Output
Explanation
We are calling the doesSubstringExists() function with s1 and s2 as parameters and are storing the returned value into the variable position. Inside the doesStringExists() function, we are iterating a loop over each character of the string s1 and are further iterating over that character up to the length of s2 with the help of an inner for loop. Inside the inner loop, we are firstly checking if the current character on which we are iterating of string s1 is the same as the current character of our s2 iteration; if not, then we are breaking the loop, if yes, then are further checking there till the length of the string s2. If finally, the value of j becomes equal to the length of s2, then we are returning the current index of i, else returning -1, and later printing it in the main() function.
More Examples
Program to check if a C++ string contains a specific substring and printing the found indices
Output
Explanation
Here in this example, we are first initializing our main string ad the target substring. After that, we are initializing a position variable to keep track of each happening iteration and an i variable to store the indexes where each substring is found. Then we are iterating over a while loop using the find() function like we did above, and are storing the index of matched substrings inside the variable i and are printing it.
Further Reading
We can follow an approach to solve this problem of substring finding more effectively: the Knuth Morris Pratt Algorithm or KMP Algorithm. As when we use the primary approach for string matching, just as we did above, it would be making some extra and unnecessary comparisons which will slow down the program execution for substantially large inputs and hence make its time complexity to be of order O(mn), where m is the length of the substring and n is the length of the given string. As an alternative, we can use the KMP algorithm to bring it to linear time; this algorithm has three components - the prefix, the suffix, and the LPS table. To read more about KMP Algorithm, visit here
Conclusion
- String matching is a prevalent task in day-to-day programming, and hence we must be aware of the ways how to do it.
- C++ provides many pre-defined methods to accomplish this task. For example - the find() function, the contains() function, the strstr() function, the boost library, and many other approaches.
- Apart from these pre-defined functions, we can also write our implementation of the string-matching problem.
- We can use the KMP algorithm to make our string-matching approach more effective and bring its time complexity into linear time.