Abstract Class in C++
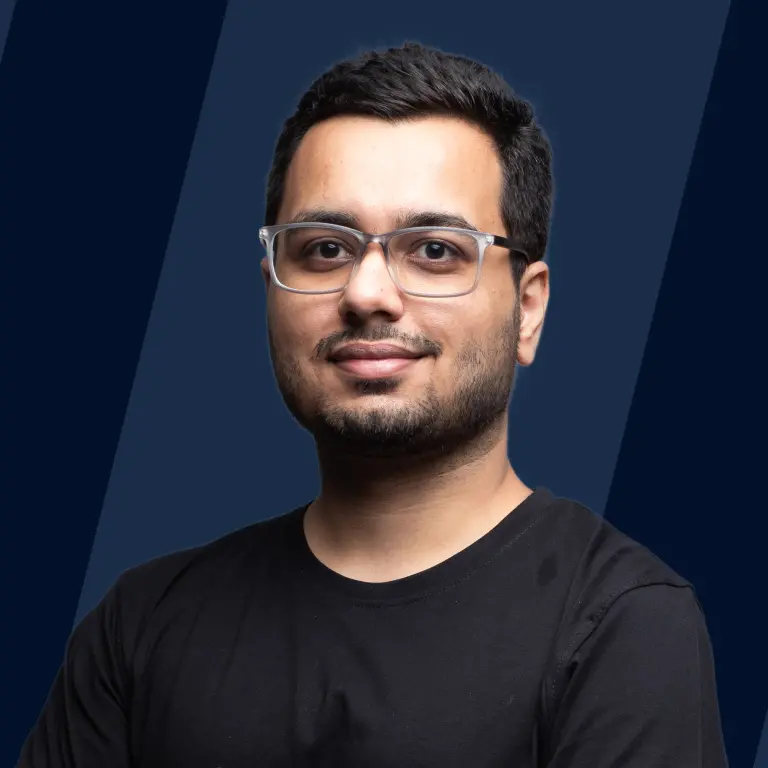
In C++, an abstract class is defined by having at least one pure virtual function, a function without a concrete definition. These classes are essential in object-oriented programming, structuring code to mirror real-life scenarios through inheritance and abstraction. Abstract classes, which cannot be instantiated, are pivotal for expressing broad concepts and upcasting, allowing derived classes to implement their interfaces. Utilize pointers and references for abstract class types, and remember that any subclass failing to define the pure virtual function becomes abstract itself. The virtual function is declared with the pure specifier (= 0).
Abstract Class in C++ Example
Let’s say we are making a calculator which returns the perimeter of the shape we put in. Think of what kind of code you would write for such a calculator. You might start with some initial shapes and hardcode the perimeter by making separate functions inside the Shape class.
The class might look something like this –
This will work, but OOP suggests that we should try to stay close to real-world logic. So, we can make a class Shape as a parent class and then make separate classes like Square and Rectangle as child classes. This will make the code easier to maintain, and if you plan to add anything new in the future, then you can add that in the child class. To implement this feature, we need to use Abstract Classes. Abstract classes in C++ need at least one pure virtual function in a class. The classes that inherit the abstract class must define the pure virtual function; otherwise, the subclass will become an abstract class.
Restrictions on Abstract Classes
There are some restrictions on abstract classes in C++.
Abstract classes cannot be used for the following –
- Variables or member data
- Argument types
- Function return types
- Types of explicit conversions.
The constructors of the abstract class in c++ can call other member functions, but if they directly or indirectly call the pure virtual function, then the result is undefined.
But wait! What is a pure virtual function anyway?
To understand the pure virtual function, first, let’s understand virtual functions.
A virtual function in C++ is a member function declared within a base class and redefined by the derived class.
A pure virtual function (or abstract function) is a virtual function with no definition/logic. It is declared by assigning 0 at the time of declaration.
Let’s take a look at an example that will make things clear.
Output –
In the above code, you can see that the function perimeter() is a pure virtual function, the “virtual” keyword is used, and it is assigned a value of 0.
In the derived classes, Rectangle and Shape redefine the pure virtual function.
Characteristics of Abstract Class in C++
- Abstract Classes must have at least one pure virtual function.
- Abstract Classes cannot be instantiated, but pointers and references of Abstract Class types can be created. You cannot create an object of an abstract class. Here is an example of a pointer to an abstract class.
Output –
- Abstract Classes are mainly used for Upcasting, which means its derived classes can use its interface.
- Classes that inherit the Abstract Class must implement all pure virtual functions. If they do not, those classes will also be treated as abstract classes.
Why Can’t We Make an Abstract Class Object?
Abstract classes in C++ cannot be instantiated because they are “abstract”. It’s like someone is telling you to draw an animal without telling you which specific animal. You can only draw if the person asks you to draw a cat, dog, etc., but an animal is abstract.
The same is the case with abstract classes in C++, so we can’t create objects, but we can create a pointer of an abstract class.
Conclusion
Through this article, you now know:
- What is Abstract Class in C++?
- What is Inheritance?
- What is Abstraction?
- How to create and work with Abstract Class in C++?
- What are virtual functions, and what is the difference between virtual and pure virtual functions?
That’s all for now, folks!
Thanks for reading.