Complex Declarations in C++
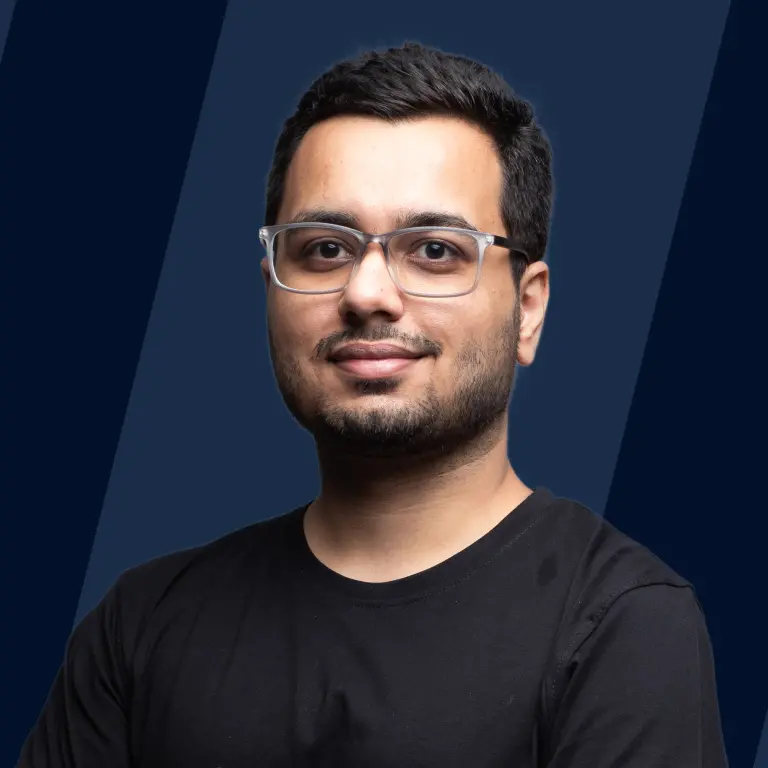
Overview
In C++ programming almost every time declarations are easy to read and understand but sometimes there are some complex declarations too that are hard to read and understand to ace in C++ programming it is important to understand complex declarations too.
Declaration
Let us see some declarations in C++:
-
int age = 21; This is a simple declaration in which we have declared an integer variable named age whose value is 21.
-
int *ptr; In this declaration, we have declared an integer pointer ptr.
-
int* ptr1, ptr2; In this declaration, we have declared an integer pointer ptr1 and an integer variable ptr2.
-
int* ptr1, *ptr2; In this declaration, we have declared two integer pointers ptr1 and ptr2.
-
int *ptr[5]; In this declaration, we have declared an array of 5 pointers to integers.
Introduction
We have seen simple declarations in C++ like int val; but there are complex declarations in C++ too like declarations involving pointers, for example, consider the following declaration char (*ptr) (), it represents a pointer to a function returning char.
The Const Modifier
In C++, the const keyword prevents a variable from being modified and when we declare a const variable we need to initialize it because we cannot initialize it after declaration. Also, const could be used with function declaration to restrict function parameters to change.
For Example :
- const int age = 21; // integer constant variable with value 21
- int const age = 21; // same meaning as above, C++ allows both types of declaration.
The examples discussed above are simple to understand but things become a little complicated when pointers are involved with the const keyword. Let us understand through a few examples:
- const int *ptr1; // pointer to a constant integer.
- int const *ptr2; // same meaning as above, C++ allows both types of declaration.
Note : Above two examples convey that we cannot change the value of the variable using *ptr1 or *ptr2.
- int * const ptr3= &age; // constant pointer to an integer, means we cannot point the pointer ptr3 to any other address.
- const int * const ptr4 = &age; // constant pointer to constant integer, here age is an constant integer.
- int * const * ptr5; // pointer to const pointer to int.
- const int * const * ptr6; // pointer to const pointer to const int.
- const int **ptr6; // pointer to pointer to const int.
- int * const * const ptr7; // const pointer to const pointer to int.
- const int * const * const ptr8; // const pointer to const pointer to const int
The Use of Typedef
In C++, typedef stands for type definition, which is a keyword used to assign a new name to the pre-existing datatype. For example, there is a datatype in C++ long long int and suppose it is used many times in the code then instead of writing this big word again and again we can use typedef to shorten the name like typedef long long int ll;, now we can use ll instead of long long int in the code.
typedef can also be used while declaring a structure in C++. For example:
Now to declare a variable of type student we can do in the following way stu s; instead of struct student s;
Function pointers
Pointers are used to point to variables, similarly, function pointers are used to point to functions.
For example:
-
int (*funptr) (int, int) The above line means there is a function pointer named funptr which points to a function which accepts two integer arguments and its return type is an integer.
-
int (*ptr) (float) The above line means there is a function pointer named ptr which points to a function which accepts one float argument and its return type is an integer.
We can call a function using function pointer, for example consider the below code.
Output:
The Right-Left Rule
There is a simple rule that helps us to read any declaration in C++ :
- Convert the given declaration into postfix form.
- To convert an expression into postfix form, begin at the innermost parentheses and go right. If the innermost parenthesis is missing, begin at the declaration's name and go right. When you reach the end of the parentheses, go to the left. Come out of parentheses once the entire parentheses have been parsed.
- Continue the process until the whole declaration is finished.
- Once the declaration is converted into postfix form read it from left to right.
Examples
Declaration | Postfix form | Meaning |
---|---|---|
char (*ptr) () | ptr * () char | ptr is a pointer to a function returning char |
float (*week)[7] | week * [7] float | week is pointer to array of 7 floating point integers. |
int (*ptr[5]) (int, char) | ptr[5] * (int, char) void | ptr is an array of 5 pointer to a function that accepts 2 arguments of type int and char and returns int |
int (*(*ptr())[]) () | ptr () * [] * () int | ptr is a function returning pointer to array of pointers to function returning int |
int (*(*ptr[2])())[4] | ptr[2] * () * [4] int | ptr is an array of 2 pointers to function returning pointer to array of 4 integers |
char ((*days[5])()) () | days[5] * () * () * char | days is an array of 5 pointers to function returning pointer to function returning pointer to character |
Conclusion
- Most of the time in programming we encounter simple declarations but there are complicated declaration too, so to read and understand them we use right-left rule.
- According to right-left rule, first convert the given declaration into postfix form and read it from left to right.
- const keyword prevents a variable from being modified and it should be initialized while declaring.
- typedef is a keyword used to assign a new name to the pre-existing datatype.
- Function pointers are used to point to function.