Constants in c++
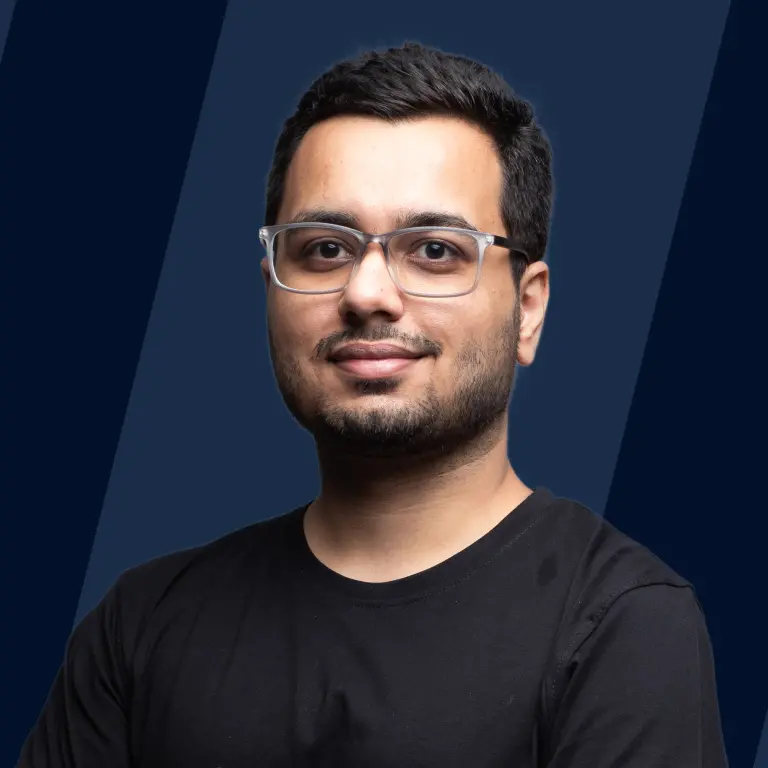
Overview
Every programming language is made up of tokens. Tokens are the smallest element that the compiler can interpret. Constants in C++ are the tokens that are defined at the time of initialization and the assigned value cannot be altered or updated after that. The define preprocessor directive and the const keyword are the two methods to define a constant. The value stored in a constant variable is known as a literal.
Defining Constants
Constants in C++ are the tokens which are defined at the time of initialization and the assigned value cannot be altered or updated after that. There are two methods to define constants in C++.
- #define preprocessor directive method
- 'const' keyword method
Define preprocessor directive method:
This preprocessor directive provides an alias or a reference name to any variable or value. It is used to define constants in C++ by giving alias names to the value. This method defines constants globally.
Syntax:
ConstantName: It is the identifier through which the value is going to be referred in the code. Value: It is the value whose reference is being created.
Example:
Output:
Explanation:
In the above example, areas of three shapes are calculated using a constant pi which is defined globally #define preprocessor directive method.
'const' keyword method:
The definition of constants in C++ is very similar to the definition of variables in C++ but the definition is started with a const keyword. The definition follows a particular pattern, starting with a const keyword followed by a datatype, an identifier, an assignment operator, and the value. Constants can be defined locally or globally through this method.
Syntax:
constantName: It is the identifier in which the value is being stored. value: It is the value that is being stored in the constantName.
Example:
Output:
Explanation:
In the above example, areas of three shapes are calculated using a constant pi which is defined globally using the 'const' keyword and in a function calculating the area of a cone, the height of the cone is declared constant and is defined locally.
Literals
The value stored in a constant variable is known as a literal. However, constants and literals are often considered synonyms. Literals can be classified on the basis of datatypes.
Types of literals:
- Integer literals
- Floating-point literals
- Characters literals
- Strings literals
- Boolean literals
- User-defined literals
Integer Literals
When integer values are stored and represented as literal, then such literals are known as integer literals. There are two types of integer literal:
- Prefixes
- Suffixes
1. Prefixes: The bases of the integer values are represented through the prefix of the integer literals. For example, 0x80 = 128, here 0x represents HexaDecimal base and the value in decimal is 128.
There are four types of prefixes used to represent integer literals:
- Decimal-literal
- Octal-literal
- Hex-literal
- Binary-literal
i. Decimal-literal: Decimal-literals have base 10, which does not contain any prefix for representation. It contains only decimal digits (0,1,2,3,4,5,6,7,8,9). For example, 10,22,34 etc.
ii. Octal-literal: The base of the octal-literals is 8 and uses 0 as prefix for representation. It contains only octal digits (0,1,2,3,4,5,6,7). For example, 010,022,034 etc.
iii. Hex-literal: The base of the Hex-literals is 16 and uses 0x or 0X as the prefix for representation. It contains only hexadecimal digits (0,1,2,3,4,5,6,7,8,9, a or A, b or B, c or C, d or D, e or E, f or F). For example, 0x80,0x16,0x4A etc.
iv. Binary-literal: The base of the Binary-literals is 2 and uses 0b or 0B as the prefix for representation. It contains only binary digits (0,1). For example, 0b11,0b110,0B111 etc.
Example:
Output:
Explanation:
In the above code, declaration and initialization of prefix integer literals of various types are performed. All the literal are then printed.
2. Suffixes:
The type of integer values are represented through the suffixes of the integer literals. For example, 3826382382688LL, 2836263826823909ULL etc. In the above example, LL represents long long int for the value 3826382382688 and ULL represents unsigned long long int for the value 2836263826823909.
Following are the types of suffixes used to represent integer literals:
i. int: It is the default integer type and hence its representation does not need any suffix. The value of integer literals ranges from -2147483648 to 2147483647.
ii. unsigned int: It is the integer type that does not contain negative int values. The value of unsigned integer literals ranges from 0 to 4294967295. A compiler error will be triggered if any negative value gets assigned to unsigned integer literals. The literal contains u or U as the suffix for its representation.
iii. long int: The value of long integer literals ranges from -2,147,483,648 to 2,147,483,647. The literal contains l or L as the suffix for its representation.
iv. unsigned long int: The value of unsigned long integer literals ranges from 0 to 4,294,967,295. The literal contains ul or UL as the suffix for its representation.
v. long long int: The value of long long integer literals ranges from -(2^63) to (2^63)-1. The literal contains ll or LL as the suffix for its representation.
vi. unsigned long long int: The value of unsigned long long integer literals ranges from 0 to 18,446,744,073,709,551,615. The literal contains ull or ULL as the suffix for its representation.
Type of integer literal | Suffixes of integer literal |
---|---|
int | No Suffix |
unsigned int | u or U |
long int | l or L |
unsigned long int | ul or UL |
long long int | ll or LL |
unsigned long long int | ull or ULL |
Example:
Output:
Explanation: In the above code, declaration and initialization of suffix integer literals of various types are performed. All the literal are then printed.
Floating-Point Literals
The floating-point literals contain the real numbers. The real numbers hold an integer part, a real part and a fractional part and an exponential part in it. A floating-point literal can be stored or represented in two forms: decimal or exponential form. In order to produce valid literals, the following rules are necessary while using floating-point literals in their decimal form:
- In the decimal form, it is necessary to add a decimal point, exponent part or both, or else it will give an error.
- In the exponential form, it is necessary to add the integer part, fractional part or both, or else, it will give an error.
Example:
Output:
Explanation:
In the above code, declaration, initialization and display of floating-point literal is performed.
Character Literals
When a single character enclosed by a single quote is stored and represented as a literal, then the literal is known as a character literal. More than one character should not be stored as a character literal otherwise, it will show a warning along with only the last character of the literal will be displayed. An array of characters is used in order to make a literal of more than one character. Character literals can be represented in the following ways:
char type: All the characters belonging to the ASCII table can be represented and stored through this type of literal. wchar_t type: All the characters belonging to the UNICODE table can be represented and stored through this type of literal. They occupy double the space of char type. The characters are followed ‘L’.
Example:
Output:
Explanation:
In the above code, declaration, initialization and display of character and wide-character literal are performed.
String Literals
When more than one character is stored in double-quotes and represented as literals. Such a literal is known as a string literal. It can store all the special as well as escape sequence characters.
Example:
Output:
Explanation:
In the above code, declaration, initialization and display of string literal is performed.
Boolean Literals
This literal stores boolean values i.e. True and false. True is used to represent success while false represents failure. True is the same as int 1 while false is similar to int 0.
Example:
Output:
Explanation:
In the above code, declaration, initialization and display of boolean literal is perfomed.
User-Defined Literals
They are the literals whose functionalities and uses are created by users with the help of either functions, classes, or structures. They come into the picture when in-built operations are unable to fulfil our needs. The name of the functions which defines the functionality of user-defined operators starts with operator"" along with the name of the operator. The user-defined literals are used similar to other literals.
Limitations:
UDLs supports only the following data-types:
- char const*
- unsigned long long
- long double
- char const*, std::size_t
- wchar_t const*, std::size_t
- char16_t const*, std::size_t
- char32_t const*, std::size_t
Example:
Output:
Explanation:
- The user-defined literals are defined in functions having the keyword operator and using unsigned long long as their respective datatypes.
- In the above code unsigned long long has been defined as ull using #define directive.
- The user-defined literal _hrs is defined with the argument as ’x’ having datatype as unsigned long long converting x into seconds by multiplying it with 3600.
- The user-defined literal _min is defined with the argument as ’x’ having datatype as unsigned long long converting x into seconds by multiplying it with 60.
- The user-defined literal _sec is defined with the argument as ’x’ having datatype as unsigned long long which is already in seconds so just return it. ‘time1’ and ‘time2’ are initialized using used defined literals.
time1 = 4_hrs+20_min+40_sec
time2 = 14_min+50_min+30_sec
which are converted into seconds with the help of user-defined literals.
time1 = 15640 seconds
time2 = 3870 seconds
- The difference between time1 and time2 is calculated and displayed.
Conclusion
- Constants in C++ are the tokens defined at the time of initialization, and the assigned value cannot be altered or updated after that.
- #define preprocessor is used to define constant by giving alias names to the value.
- The const keyword follows a particular pattern, const keyword -> datatype -> variable -> assignment operator -> value
- The value stored in a constant variable is known as a literal.
- Integer, Floating-point, Character, String and Boolean literals use in-built datatypes and work accordingly.
- User-defined literals are the literals whose functionalities and uses are created by users with the help of either functions, classes or structures.