C++ Header Files
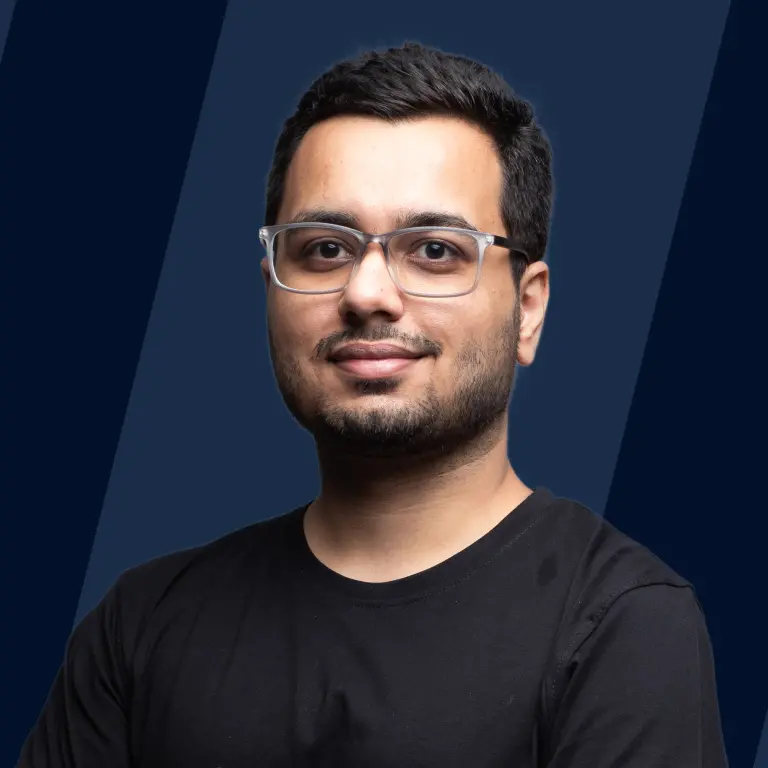
Header files are crucial in C++, housing function definitions and data types to facilitate programming. They streamline code in large projects and are included using the #include directive. This article explores the use and contents of C++ header files.
Contents of the Header File in C++
A header file in C++ contains:
Function Definitions
A header file contains many predefined functions that can be used by simply including the header file in our program. For example, pow(a,b) function in math.h header file takes two arguments , and returns ;
Data Type Definition
Header files can also contain some data types definitions, which are frequently used by C++ programmers so that we can use these predefined data types in our program. E.g., time_t is an arithmetic data type used to represent time.
Macros
Macro is a piece of code that gets replaced by its value. We use macros to avoid retyping the whole piece of code if it is frequently used in our program.
Macros are defined by the #define directive, and whenever the compiler sees a macro, it replaces it with the value contained in it.
Example:
Observe that the macro definition does not end with a semicolon(;).
We can define macros in header files and use them in our program by simply including them in our program, and we could also use pre-defined macros by including the respective header file.
eg:. __DATE__ stores the compilation date of the current source file in the form mmm dd yyyy.
Classes
Header files also contain pre-defined classes which provide many useful functionalities which make our programming easier.
For example, the C++ string class is used to store the sequence of characters and is dynamic.
Types of C++ Header Files
There are two types of header files in C++:
-
Pre-existing Header Files These are the header files that are already present in the C++ directives and to use them. We need to include them in our program. They are generally included in the program using angular brackets.
eg #include<iostream> -
User-defined Header Files
These are the header files created by the user and can be included in the program using double quotes.
To create your header file, perform the below steps:
- Write a code in C++ and save it with the .h extension.
Let's save the above code file with the multiply.h name.
- Include your header file using #include
Output:
How do C++ Header Files work?
There are two things you need to remember while including a header :
1. #include: This is the pre-processor directive that tells the compiler to process the file before compilation and includes all the functions or data members in the file.
2. <filename.h>: This is the name of the header which you want to include.
So this is how the working of the header file takes place by telling the compiler to process the header file with the name "filename" using the pre-processor directive #include.
Including Multiple Header Files in C++
We can include multiple header files in our program, but when a header file is included more than once in a program, the compiler processes the contents of that file twice, leading to an error in the program. To resolve this error, we use conditional preprocessor directives, known as guards.
These guards prevent a header file from being included twice.
Syntax:
symbol_name is usually the name of the header file in all capital letters. "#ifndef” ensures that a header is not included again, and if the header file is included again, then this condition will become false because symbol_name is defined. The preprocessor will skip over the entire file contents and move further with the execution, preventing the inclusion of the same header file twice.
Multiple conditionals include multiple header files based on the program's requirements.
Syntax:
Standard Header Files And Their Uses
C++ has a vast library providing many valuable functionalities to ease our coding.
Below are some libraries and their header files:
Input/output library
-
<iostream> It takes input and displays output from the console using cin and cout, respectively.
-
<fstream> It is used to create files and write and read information from files.
Numerics library
-
<cmath.h> It is used to perform common mathematical operations like sqrt(), pow() etc.
-
<complex.h> Deals with complex number operations and manipulations.
Algorithms library
- <algorithm> It contains algorithms that operate on C++ containers like vectors, maps, etc.
Containers library
-
<vector> Vectors are dynamic arrays in C++.
-
<deque> Double-ended queues are sequence containers with the feature of insertion and deletion of elements from both ends.
-
<set> Sets are a type of associative container which stores unique elements. The values are stored in a specific order.
-
<map> Maps are associative containers that stores key-value pair, stores unique keys.
-
<unordered_set> This container is the same as a set, but it doesn't store values in an ordered fashion and internally uses hash tables to store values.
-
<unordered_map> This container is the same as a map, but it doesn't store values in an ordered fashion and internally uses hash tables to store values.
-
<stack> Stacks are containers that store values in a LIFO(last in, first out) manner. Elements are inserted and removed from one end only.
-
<queue>
The queue is a type of container that stores values in a FIFO(first in, first out) manner. Elements are inserted at one end and removed from the other end.
Strings library
-
<string> It is used to perform operations on strings. Some of the operations include strcmp(), size() etc.
-
<ctype.h> It contains inbuilt functions to handle characters.
Angle brackets(<>) vs double quotes("")
There are two ways in which we can include a header file in our program :
Using Angle Brackets
Angle brackets tell the preprocessor that we didn't write this header file and tell the compiler to search the header file in the directories specified by the include directive only. The include directive is configured as a part of your project/IDE settings/compiler settings. The compiler will not search for the header file in your current directory in which your source code is present.
eg: #include<iostream>
Using Double Quotes
Double-quotes tell the preprocessor that we wrote this header file and tell the compiler to first search for the header file in the current directory in which our project source code is present. If it is not found there, search the header file in the directories specified by the include directive.
eg: #include "my_file.h"
Why Doesn’t iostream have a .h Extension?
Inside the older versions of C++, all the header files ended with the .h extension. The authentic version of cout and cin had been found in iostream.h. When the language became standardized through the ANSI committee, they moved all the features inside the runtime library into the std namespace. However, this created trouble as the old programs were not working anymore.
To solve this problem, a new set of header files was introduced. These new header files had the same name as earlier but without the .h extension and have all their capabilities within the std namespace. This way, older programs that include #include <iostream.h> do not need to be rewritten, and newer programs can #include <iostream>.
Including C++ Header Files from Other Directories
There are two ways in which you can include header files from other directories:
- Specify the relative path to the header file with the #include line.
Example :
This is not the recommended way because if you change your directory structure, the compiler will no longer include this header file.
- A recommended method is to tell your compiler about the location of header files so that it will search there when it can’t find them in the current directory.
To do this, use the -Ioption to specify an alternate include directory:
The good thing about this way is that when you change your directory structure then, you do not have to change your code.
Good Practices Related to C++ Header Files
These are some of the best practices that one should follow while creating and using header files:
- Always use header guards because we know that according to one definition rule, a variable or function can only have one definition. Multiple definitions will result in a compilation error. Header guards ensure that a particular variable or function is included only once in a header file.
- Don't use the same name function in different header files in the same project/directory, as it also leads to conflict.
- Name of the header file and its associated source file should be the same.
- Every header file should have a specific job.
- Only include those header files whose functionalities you want to use, and unnecessarily do not include header files that you do not need because this will only increase the execution time of your program.
- If you create your header file, always save it with the .h extension.
- User-defined header files should be included using double quotes and angle brackets for the pre-written header files.
Sample C++ Header File
my_header_file.h
The above example shows a header file's various declarations and definitions.
Your journey to becoming a certified C++ guru starts here. Enroll now in Scaler Topics Free Course and pave your way to success in the world of programming.
Conclusion
In this article, we learned about:
- Header files contain function and datatype definitions, and these header files are included in the program using the pre-processor directive #include.
- There are two types of header files, pre-existing header files that come with the compiler and user-defined header files.
- Pre-existing header files are included in the program using angle brackets, and user-defined header files are included using double quotes.
- Header guards are used to prevent the inclusion of header files multiple times in our program.